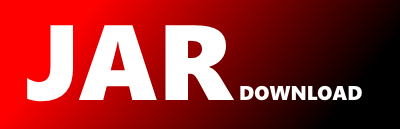
cn.com.antcloud.api.catronus.v1_0.model.RiskTask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antcloud-api-catronus Show documentation
Show all versions of antcloud-api-catronus Show documentation
Ant Chain API SDK For Java
Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
The newest version!
//
// Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
//
//
package cn.com.antcloud.api.catronus.v1_0.model;
import java.lang.Long;
import java.lang.String;
import java.util.Date;
import java.util.List;
import javax.validation.constraints.NotNull;
/**
* 风险任务/工单 */
public class RiskTask {
@NotNull
private String id;
@NotNull
private String displayName;
@NotNull
private String priority;
@NotNull
private String state;
@NotNull
private String riskType;
@NotNull
private String owner;
@NotNull
private Date deadlineTime;
@NotNull
private Date createdTime;
@NotNull
private String respondPhase;
@NotNull
private List followers;
@NotNull
private String secOwner;
@NotNull
private Long daysLeft;
@NotNull
private String riskId;
/**
* ID */
public String getId() {
return this.id;
}
/**
* ID */
public void setId(String id) {
this.id = id;
}
/**
* 风险名称 */
public String getDisplayName() {
return this.displayName;
}
/**
* 风险名称 */
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
/**
* 优先级 */
public String getPriority() {
return this.priority;
}
/**
* 优先级 */
public void setPriority(String priority) {
this.priority = priority;
}
/**
* 当前状态 */
public String getState() {
return this.state;
}
/**
* 当前状态 */
public void setState(String state) {
this.state = state;
}
/**
* 风险类型 */
public String getRiskType() {
return this.riskType;
}
/**
* 风险类型 */
public void setRiskType(String riskType) {
this.riskType = riskType;
}
/**
* 负责人 */
public String getOwner() {
return this.owner;
}
/**
* 负责人 */
public void setOwner(String owner) {
this.owner = owner;
}
/**
* 到期时间 */
public Date getDeadlineTime() {
return this.deadlineTime;
}
/**
* 到期时间 */
public void setDeadlineTime(Date deadlineTime) {
this.deadlineTime = deadlineTime;
}
/**
* 创建时间 */
public Date getCreatedTime() {
return this.createdTime;
}
/**
* 创建时间 */
public void setCreatedTime(Date createdTime) {
this.createdTime = createdTime;
}
/**
* 响应阶段 */
public String getRespondPhase() {
return this.respondPhase;
}
/**
* 响应阶段 */
public void setRespondPhase(String respondPhase) {
this.respondPhase = respondPhase;
}
/**
* 风险关注者 */
public List getFollowers() {
return this.followers;
}
/**
* 风险关注者 */
public void setFollowers(List followers) {
this.followers = followers;
}
/**
* 安全工程师 */
public String getSecOwner() {
return this.secOwner;
}
/**
* 安全工程师 */
public void setSecOwner(String secOwner) {
this.secOwner = secOwner;
}
/**
* 到期天数 */
public Long getDaysLeft() {
return this.daysLeft;
}
/**
* 到期天数 */
public void setDaysLeft(Long daysLeft) {
this.daysLeft = daysLeft;
}
/**
* 风险ID */
public String getRiskId() {
return this.riskId;
}
/**
* 风险ID */
public void setRiskId(String riskId) {
this.riskId = riskId;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy