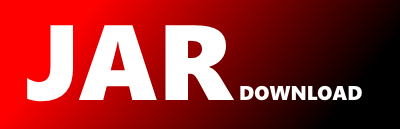
cn.com.antcloud.api.catronus.v1_0.request.QueryRiskTasksRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antcloud-api-catronus Show documentation
Show all versions of antcloud-api-catronus Show documentation
Ant Chain API SDK For Java
Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
The newest version!
//
// Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
//
//
package cn.com.antcloud.api.catronus.v1_0.request;
import cn.com.antcloud.api.catronus.v1_0.response.QueryRiskTasksResponse;
import cn.com.antcloud.api.product.AntCloudProdRequest;
import java.lang.Long;
import java.lang.String;
import java.util.Date;
import javax.validation.constraints.NotNull;
/**
* 查询风险任务列表 */
public class QueryRiskTasksRequest extends AntCloudProdRequest {
@NotNull
private Long page;
@NotNull
private Long pageSize;
private String riskTaskId;
private String displayName;
private String priority;
private String riskType;
private String riskTaskState;
private Date creationTimeFrom;
private Date creationTimeTo;
private String _prod_code = "CATRONUS";
public QueryRiskTasksRequest(String productInstanceId) {
super("antcloud.catronus.risk.tasks.query", "1.0", "Java-SDK-20230525", productInstanceId);
}
public QueryRiskTasksRequest() {
super("antcloud.catronus.risk.tasks.query", "1.0", null);
this.setSdkVersion("Java-SDK-20230525");
}
/**
* Product code */
public String get_prod_code() {
return this._prod_code;
}
/**
* Product code */
public void set_prod_code(String _prod_code) {
this._prod_code = _prod_code;
}
/**
* 当前页数 */
public Long getPage() {
return this.page;
}
/**
* 当前页数 */
public void setPage(Long page) {
this.page = page;
}
/**
* 页大小 */
public Long getPageSize() {
return this.pageSize;
}
/**
* 页大小 */
public void setPageSize(Long pageSize) {
this.pageSize = pageSize;
}
/**
* 风险ID */
public String getRiskTaskId() {
return this.riskTaskId;
}
/**
* 风险ID */
public void setRiskTaskId(String riskTaskId) {
this.riskTaskId = riskTaskId;
}
/**
* 风险名称 */
public String getDisplayName() {
return this.displayName;
}
/**
* 风险名称 */
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
/**
* 风险等级 */
public String getPriority() {
return this.priority;
}
/**
* 风险等级 */
public void setPriority(String priority) {
this.priority = priority;
}
/**
* 风险类型 */
public String getRiskType() {
return this.riskType;
}
/**
* 风险类型 */
public void setRiskType(String riskType) {
this.riskType = riskType;
}
/**
* 风险状态 */
public String getRiskTaskState() {
return this.riskTaskState;
}
/**
* 风险状态 */
public void setRiskTaskState(String riskTaskState) {
this.riskTaskState = riskTaskState;
}
/**
* FROM时间点, 按照时间范围搜索的开始时间点 */
public Date getCreationTimeFrom() {
return this.creationTimeFrom;
}
/**
* FROM时间点, 按照时间范围搜索的开始时间点 */
public void setCreationTimeFrom(Date creationTimeFrom) {
this.creationTimeFrom = creationTimeFrom;
}
/**
* to时间点, 按照时间范围搜索的结束时间点
*/
public Date getCreationTimeTo() {
return this.creationTimeTo;
}
/**
* to时间点, 按照时间范围搜索的结束时间点
*/
public void setCreationTimeTo(Date creationTimeTo) {
this.creationTimeTo = creationTimeTo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy