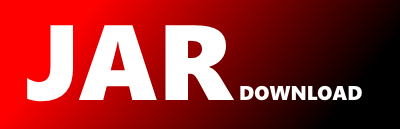
cn.com.antcloud.api.riskplus.v1_0.model.AICallbackMessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antcloud-api-riskplus Show documentation
Show all versions of antcloud-api-riskplus Show documentation
Ant Chain API SDK For Java
Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
//
//
package cn.com.antcloud.api.riskplus.v1_0.model;
import java.lang.Long;
import java.lang.String;
import javax.validation.constraints.NotNull;
/**
* ai外呼回调详情 */
public class AICallbackMessage {
private String batchId;
@NotNull
private String tag;
@NotNull
private String callId;
private Long templateId;
@NotNull
private Long statusCode;
@NotNull
private String statusDescription;
@NotNull
private String importTime;
@NotNull
private String callBeginTime;
@NotNull
private Long ringTime;
@NotNull
private String answerTime;
@NotNull
private Long speakingDuration;
@NotNull
private String hangupTime;
@NotNull
private Long speakingTurns;
@NotNull
private String intentTag;
@NotNull
private String intentDescription;
@NotNull
private String individualTag;
@NotNull
private String keywords;
private String chatRecord;
@NotNull
private String properties;
/**
* 批次号 */
public String getBatchId() {
return this.batchId;
}
/**
* 批次号 */
public void setBatchId(String batchId) {
this.batchId = batchId;
}
/**
* 用户标签 */
public String getTag() {
return this.tag;
}
/**
* 用户标签 */
public void setTag(String tag) {
this.tag = tag;
}
/**
* 外呼id */
public String getCallId() {
return this.callId;
}
/**
* 外呼id */
public void setCallId(String callId) {
this.callId = callId;
}
/**
* 外呼的话术模板Id */
public Long getTemplateId() {
return this.templateId;
}
/**
* 外呼的话术模板Id */
public void setTemplateId(Long templateId) {
this.templateId = templateId;
}
/**
* 外呼状态编码 */
public Long getStatusCode() {
return this.statusCode;
}
/**
* 外呼状态编码 */
public void setStatusCode(Long statusCode) {
this.statusCode = statusCode;
}
/**
* 外呼状态描述 */
public String getStatusDescription() {
return this.statusDescription;
}
/**
* 外呼状态描述 */
public void setStatusDescription(String statusDescription) {
this.statusDescription = statusDescription;
}
/**
* 导入时间 */
public String getImportTime() {
return this.importTime;
}
/**
* 导入时间 */
public void setImportTime(String importTime) {
this.importTime = importTime;
}
/**
* 开始通话时间 */
public String getCallBeginTime() {
return this.callBeginTime;
}
/**
* 开始通话时间 */
public void setCallBeginTime(String callBeginTime) {
this.callBeginTime = callBeginTime;
}
/**
* 振铃时长, 单位毫秒 */
public Long getRingTime() {
return this.ringTime;
}
/**
* 振铃时长, 单位毫秒 */
public void setRingTime(Long ringTime) {
this.ringTime = ringTime;
}
/**
* 接通时间 */
public String getAnswerTime() {
return this.answerTime;
}
/**
* 接通时间 */
public void setAnswerTime(String answerTime) {
this.answerTime = answerTime;
}
/**
* AI通话时长,单位s */
public Long getSpeakingDuration() {
return this.speakingDuration;
}
/**
* AI通话时长,单位s */
public void setSpeakingDuration(Long speakingDuration) {
this.speakingDuration = speakingDuration;
}
/**
* 挂断时间 */
public String getHangupTime() {
return this.hangupTime;
}
/**
* 挂断时间 */
public void setHangupTime(String hangupTime) {
this.hangupTime = hangupTime;
}
/**
* 对话轮次 */
public Long getSpeakingTurns() {
return this.speakingTurns;
}
/**
* 对话轮次 */
public void setSpeakingTurns(Long speakingTurns) {
this.speakingTurns = speakingTurns;
}
/**
* 意向标签 */
public String getIntentTag() {
return this.intentTag;
}
/**
* 意向标签 */
public void setIntentTag(String intentTag) {
this.intentTag = intentTag;
}
/**
* 意向说明 */
public String getIntentDescription() {
return this.intentDescription;
}
/**
* 意向说明 */
public void setIntentDescription(String intentDescription) {
this.intentDescription = intentDescription;
}
/**
* 个性标签 */
public String getIndividualTag() {
return this.individualTag;
}
/**
* 个性标签 */
public void setIndividualTag(String individualTag) {
this.individualTag = individualTag;
}
/**
* 回复关键词 */
public String getKeywords() {
return this.keywords;
}
/**
* 回复关键词 */
public void setKeywords(String keywords) {
this.keywords = keywords;
}
/**
* 对话录音 */
public String getChatRecord() {
return this.chatRecord;
}
/**
* 对话录音 */
public void setChatRecord(String chatRecord) {
this.chatRecord = chatRecord;
}
/**
* 参数值 */
public String getProperties() {
return this.properties;
}
/**
* 参数值 */
public void setProperties(String properties) {
this.properties = properties;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy