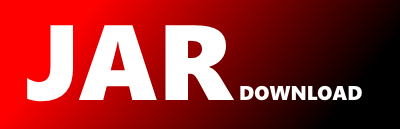
cn.com.antcloud.api.riskplus.v1_0.model.CompanyInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antcloud-api-riskplus Show documentation
Show all versions of antcloud-api-riskplus Show documentation
Ant Chain API SDK For Java
Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
//
//
package cn.com.antcloud.api.riskplus.v1_0.model;
import java.lang.Long;
import java.lang.String;
import javax.validation.constraints.NotNull;
/**
* 企业信息 */
public class CompanyInfo {
@NotNull
private String activeAddrJson;
@NotNull
private String activeCity;
@NotNull
private String activeCounty;
@NotNull
private String activeProvince;
@NotNull
private String categories;
@NotNull
private String checkDate;
@NotNull
private String delFlag;
@NotNull
private String dt;
@NotNull
private String formerOrgNames;
@NotNull
private String headOffice;
@NotNull
private Long id;
@NotNull
private Long involvedAmount;
@NotNull
private Long involvedPeople;
@NotNull
private String keyRelaOrgs;
@NotNull
private String legalRepresentative;
@NotNull
private String mctOneId;
@NotNull
private String operatingAddrJson;
@NotNull
private String operatingCity;
@NotNull
private String operatingCounty;
@NotNull
private String operatingPlace;
@NotNull
private String operatingProvince;
@NotNull
private String oprtActvState;
@NotNull
private String oprtEndDate;
@NotNull
private String oprtScope;
@NotNull
private String oprtStartDate;
@NotNull
private String opType;
@NotNull
private String orgCode;
@NotNull
private String orgEmail;
@NotNull
private String orgName;
@NotNull
private String orgRegCptlRmb;
@NotNull
private String orgState;
@NotNull
private String orgTel;
@NotNull
private String orgType;
@NotNull
private String platformName;
@NotNull
private String platformStates;
@NotNull
private String registerCapitalCurrency;
@NotNull
private String registerCapitalValue;
@NotNull
private String registerCity;
@NotNull
private String registerCounty;
@NotNull
private String registerDate;
@NotNull
private String registerPlace;
@NotNull
private String registerProvince;
@NotNull
private String registrationAuthority;
@NotNull
private String regNo;
@NotNull
private String riskstormCompanyId;
@NotNull
private String riskFactors;
@NotNull
private String riskGraphJson;
@NotNull
private String riskMessage;
@NotNull
private Long riskScore;
@NotNull
private String riskScoreTrend;
@NotNull
private Long riskScoreWeeklyFloat;
@NotNull
private String riskTags;
@NotNull
private String riskTagsId;
@NotNull
private String riskType;
@NotNull
private String searchContent;
@NotNull
private Long spreadNumber;
@NotNull
private String spreadNumDistribution;
@NotNull
private String ucCode;
@NotNull
private String updateDate;
@NotNull
private String platform;
/**
* 活跃地json */
public String getActiveAddrJson() {
return this.activeAddrJson;
}
/**
* 活跃地json */
public void setActiveAddrJson(String activeAddrJson) {
this.activeAddrJson = activeAddrJson;
}
/**
* 活跃市(字段停用) */
public String getActiveCity() {
return this.activeCity;
}
/**
* 活跃市(字段停用) */
public void setActiveCity(String activeCity) {
this.activeCity = activeCity;
}
/**
* 活跃县(字段停用) */
public String getActiveCounty() {
return this.activeCounty;
}
/**
* 活跃县(字段停用) */
public void setActiveCounty(String activeCounty) {
this.activeCounty = activeCounty;
}
/**
* 活跃省(字段停用) */
public String getActiveProvince() {
return this.activeProvince;
}
/**
* 活跃省(字段停用) */
public void setActiveProvince(String activeProvince) {
this.activeProvince = activeProvince;
}
/**
* 类经融行业分类 */
public String getCategories() {
return this.categories;
}
/**
* 类经融行业分类 */
public void setCategories(String categories) {
this.categories = categories;
}
/**
* 核准日期 */
public String getCheckDate() {
return this.checkDate;
}
/**
* 核准日期 */
public void setCheckDate(String checkDate) {
this.checkDate = checkDate;
}
/**
* 删除标志 */
public String getDelFlag() {
return this.delFlag;
}
/**
* 删除标志 */
public void setDelFlag(String delFlag) {
this.delFlag = delFlag;
}
/**
* odps数据产生时间,业务上不关心 */
public String getDt() {
return this.dt;
}
/**
* odps数据产生时间,业务上不关心 */
public void setDt(String dt) {
this.dt = dt;
}
/**
* 企业曾用名 */
public String getFormerOrgNames() {
return this.formerOrgNames;
}
/**
* 企业曾用名 */
public void setFormerOrgNames(String formerOrgNames) {
this.formerOrgNames = formerOrgNames;
}
/**
* {"mct_one_id":"ID1","org_name":"总公司名称1"} */
public String getHeadOffice() {
return this.headOffice;
}
/**
* {"mct_one_id":"ID1","org_name":"总公司名称1"} */
public void setHeadOffice(String headOffice) {
this.headOffice = headOffice;
}
/**
* 记录ID */
public Long getId() {
return this.id;
}
/**
* 记录ID */
public void setId(Long id) {
this.id = id;
}
/**
* 影响金额 */
public Long getInvolvedAmount() {
return this.involvedAmount;
}
/**
* 影响金额 */
public void setInvolvedAmount(Long involvedAmount) {
this.involvedAmount = involvedAmount;
}
/**
* 影响人数 */
public Long getInvolvedPeople() {
return this.involvedPeople;
}
/**
* 影响人数 */
public void setInvolvedPeople(Long involvedPeople) {
this.involvedPeople = involvedPeople;
}
/**
* 重要关联企业,json格式 */
public String getKeyRelaOrgs() {
return this.keyRelaOrgs;
}
/**
* 重要关联企业,json格式 */
public void setKeyRelaOrgs(String keyRelaOrgs) {
this.keyRelaOrgs = keyRelaOrgs;
}
/**
* 法人 */
public String getLegalRepresentative() {
return this.legalRepresentative;
}
/**
* 法人 */
public void setLegalRepresentative(String legalRepresentative) {
this.legalRepresentative = legalRepresentative;
}
/**
* 企业ID */
public String getMctOneId() {
return this.mctOneId;
}
/**
* 企业ID */
public void setMctOneId(String mctOneId) {
this.mctOneId = mctOneId;
}
/**
* 经营地json */
public String getOperatingAddrJson() {
return this.operatingAddrJson;
}
/**
* 经营地json */
public void setOperatingAddrJson(String operatingAddrJson) {
this.operatingAddrJson = operatingAddrJson;
}
/**
* 经营市(字段停用) */
public String getOperatingCity() {
return this.operatingCity;
}
/**
* 经营市(字段停用) */
public void setOperatingCity(String operatingCity) {
this.operatingCity = operatingCity;
}
/**
* 经营县(字段停用) */
public String getOperatingCounty() {
return this.operatingCounty;
}
/**
* 经营县(字段停用) */
public void setOperatingCounty(String operatingCounty) {
this.operatingCounty = operatingCounty;
}
/**
* 经营地址(字段停用) */
public String getOperatingPlace() {
return this.operatingPlace;
}
/**
* 经营地址(字段停用) */
public void setOperatingPlace(String operatingPlace) {
this.operatingPlace = operatingPlace;
}
/**
* 经营省(字段停用) */
public String getOperatingProvince() {
return this.operatingProvince;
}
/**
* 经营省(字段停用) */
public void setOperatingProvince(String operatingProvince) {
this.operatingProvince = operatingProvince;
}
/**
* 营运状态(1:营运0:不营运) */
public String getOprtActvState() {
return this.oprtActvState;
}
/**
* 营运状态(1:营运0:不营运) */
public void setOprtActvState(String oprtActvState) {
this.oprtActvState = oprtActvState;
}
/**
* 经营期限至 */
public String getOprtEndDate() {
return this.oprtEndDate;
}
/**
* 经营期限至 */
public void setOprtEndDate(String oprtEndDate) {
this.oprtEndDate = oprtEndDate;
}
/**
* 经营范围 */
public String getOprtScope() {
return this.oprtScope;
}
/**
* 经营范围 */
public void setOprtScope(String oprtScope) {
this.oprtScope = oprtScope;
}
/**
* 经营期限自 */
public String getOprtStartDate() {
return this.oprtStartDate;
}
/**
* 经营期限自 */
public void setOprtStartDate(String oprtStartDate) {
this.oprtStartDate = oprtStartDate;
}
/**
* 操作类型
*/
public String getOpType() {
return this.opType;
}
/**
* 操作类型
*/
public void setOpType(String opType) {
this.opType = opType;
}
/**
* 组织机构代码 */
public String getOrgCode() {
return this.orgCode;
}
/**
* 组织机构代码 */
public void setOrgCode(String orgCode) {
this.orgCode = orgCode;
}
/**
* 电子邮箱 */
public String getOrgEmail() {
return this.orgEmail;
}
/**
* 电子邮箱 */
public void setOrgEmail(String orgEmail) {
this.orgEmail = orgEmail;
}
/**
* 企业名 */
public String getOrgName() {
return this.orgName;
}
/**
* 企业名 */
public void setOrgName(String orgName) {
this.orgName = orgName;
}
/**
* 注册资本折人民币(万元) */
public String getOrgRegCptlRmb() {
return this.orgRegCptlRmb;
}
/**
* 注册资本折人民币(万元) */
public void setOrgRegCptlRmb(String orgRegCptlRmb) {
this.orgRegCptlRmb = orgRegCptlRmb;
}
/**
* 企业状态 */
public String getOrgState() {
return this.orgState;
}
/**
* 企业状态 */
public void setOrgState(String orgState) {
this.orgState = orgState;
}
/**
* 联系电话 */
public String getOrgTel() {
return this.orgTel;
}
/**
* 联系电话 */
public void setOrgTel(String orgTel) {
this.orgTel = orgTel;
}
/**
* 企业类型 */
public String getOrgType() {
return this.orgType;
}
/**
* 企业类型 */
public void setOrgType(String orgType) {
this.orgType = orgType;
}
/**
* 平台名 */
public String getPlatformName() {
return this.platformName;
}
/**
* 平台名 */
public void setPlatformName(String platformName) {
this.platformName = platformName;
}
/**
* 平台状态 */
public String getPlatformStates() {
return this.platformStates;
}
/**
* 平台状态 */
public void setPlatformStates(String platformStates) {
this.platformStates = platformStates;
}
/**
* 注册资本币种 */
public String getRegisterCapitalCurrency() {
return this.registerCapitalCurrency;
}
/**
* 注册资本币种 */
public void setRegisterCapitalCurrency(String registerCapitalCurrency) {
this.registerCapitalCurrency = registerCapitalCurrency;
}
/**
* 注册资本值 */
public String getRegisterCapitalValue() {
return this.registerCapitalValue;
}
/**
* 注册资本值 */
public void setRegisterCapitalValue(String registerCapitalValue) {
this.registerCapitalValue = registerCapitalValue;
}
/**
* 注册市 */
public String getRegisterCity() {
return this.registerCity;
}
/**
* 注册市 */
public void setRegisterCity(String registerCity) {
this.registerCity = registerCity;
}
/**
* 注册区县 */
public String getRegisterCounty() {
return this.registerCounty;
}
/**
* 注册区县 */
public void setRegisterCounty(String registerCounty) {
this.registerCounty = registerCounty;
}
/**
* 注册时间 */
public String getRegisterDate() {
return this.registerDate;
}
/**
* 注册时间 */
public void setRegisterDate(String registerDate) {
this.registerDate = registerDate;
}
/**
* 注册地址 */
public String getRegisterPlace() {
return this.registerPlace;
}
/**
* 注册地址 */
public void setRegisterPlace(String registerPlace) {
this.registerPlace = registerPlace;
}
/**
* 注册省 */
public String getRegisterProvince() {
return this.registerProvince;
}
/**
* 注册省 */
public void setRegisterProvince(String registerProvince) {
this.registerProvince = registerProvince;
}
/**
* 登记机关 */
public String getRegistrationAuthority() {
return this.registrationAuthority;
}
/**
* 登记机关 */
public void setRegistrationAuthority(String registrationAuthority) {
this.registrationAuthority = registrationAuthority;
}
/**
* 工商注册号 */
public String getRegNo() {
return this.regNo;
}
/**
* 工商注册号 */
public void setRegNo(String regNo) {
this.regNo = regNo;
}
/**
* 风报企业ID */
public String getRiskstormCompanyId() {
return this.riskstormCompanyId;
}
/**
* 风报企业ID */
public void setRiskstormCompanyId(String riskstormCompanyId) {
this.riskstormCompanyId = riskstormCompanyId;
}
/**
* 风险维度 */
public String getRiskFactors() {
return this.riskFactors;
}
/**
* 风险维度 */
public void setRiskFactors(String riskFactors) {
this.riskFactors = riskFactors;
}
/**
* 风险图谱可视化数据 */
public String getRiskGraphJson() {
return this.riskGraphJson;
}
/**
* 风险图谱可视化数据 */
public void setRiskGraphJson(String riskGraphJson) {
this.riskGraphJson = riskGraphJson;
}
/**
* 风险报文 */
public String getRiskMessage() {
return this.riskMessage;
}
/**
* 风险报文 */
public void setRiskMessage(String riskMessage) {
this.riskMessage = riskMessage;
}
/**
* 风险指数 */
public Long getRiskScore() {
return this.riskScore;
}
/**
* 风险指数 */
public void setRiskScore(Long riskScore) {
this.riskScore = riskScore;
}
/**
* 风险指数,按日的趋势图 */
public String getRiskScoreTrend() {
return this.riskScoreTrend;
}
/**
* 风险指数,按日的趋势图 */
public void setRiskScoreTrend(String riskScoreTrend) {
this.riskScoreTrend = riskScoreTrend;
}
/**
* 风险指数周波动 */
public Long getRiskScoreWeeklyFloat() {
return this.riskScoreWeeklyFloat;
}
/**
* 风险指数周波动 */
public void setRiskScoreWeeklyFloat(Long riskScoreWeeklyFloat) {
this.riskScoreWeeklyFloat = riskScoreWeeklyFloat;
}
/**
* 风险标签(字段停用) */
public String getRiskTags() {
return this.riskTags;
}
/**
* 风险标签(字段停用) */
public void setRiskTags(String riskTags) {
this.riskTags = riskTags;
}
/**
* 风险标签ID */
public String getRiskTagsId() {
return this.riskTagsId;
}
/**
* 风险标签ID */
public void setRiskTagsId(String riskTagsId) {
this.riskTagsId = riskTagsId;
}
/**
* 风险定性 */
public String getRiskType() {
return this.riskType;
}
/**
* 风险定性 */
public void setRiskType(String riskType) {
this.riskType = riskType;
}
/**
* 搜索内容 */
public String getSearchContent() {
return this.searchContent;
}
/**
* 搜索内容 */
public void setSearchContent(String searchContent) {
this.searchContent = searchContent;
}
/**
* 传播人次 */
public Long getSpreadNumber() {
return this.spreadNumber;
}
/**
* 传播人次 */
public void setSpreadNumber(Long spreadNumber) {
this.spreadNumber = spreadNumber;
}
/**
* 影响地区人次分布,json格式如下 */
public String getSpreadNumDistribution() {
return this.spreadNumDistribution;
}
/**
* 影响地区人次分布,json格式如下 */
public void setSpreadNumDistribution(String spreadNumDistribution) {
this.spreadNumDistribution = spreadNumDistribution;
}
/**
* 社会统一信用代码 */
public String getUcCode() {
return this.ucCode;
}
/**
* 社会统一信用代码 */
public void setUcCode(String ucCode) {
this.ucCode = ucCode;
}
/**
* 公有云数据库产生时间 */
public String getUpdateDate() {
return this.updateDate;
}
/**
* 公有云数据库产生时间 */
public void setUpdateDate(String updateDate) {
this.updateDate = updateDate;
}
/**
* 平台json */
public String getPlatform() {
return this.platform;
}
/**
* 平台json */
public void setPlatform(String platform) {
this.platform = platform;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy