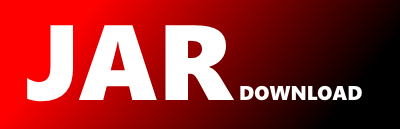
cn.com.antcloud.api.riskplus.v1_0.model.OverdueInfoResponse Maven / Gradle / Ivy
//
// Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
//
//
package cn.com.antcloud.api.riskplus.v1_0.model;
import java.lang.Boolean;
import java.lang.Long;
import java.lang.String;
import java.util.Date;
import javax.validation.constraints.NotNull;
/**
* 逾期信息查询响应 */
public class OverdueInfoResponse {
@NotNull
private Boolean overDueFlag;
@NotNull
private Long overDays;
@NotNull
private Long valuableOverDays;
@NotNull
private Long overPeriodCount;
@NotNull
private Long overPrincipal;
@NotNull
private Long overInterest;
@NotNull
private Long overPunish;
@NotNull
private Long needOverdueAmount;
@NotNull
private Long currentNeedAmount;
@NotNull
private Long totalAmount;
@NotNull
private Date settleDate;
@NotNull
private String receiptNo;
@NotNull
private Long alreadyRepayPeriodCount;
@NotNull
private Long loanPeriodCount;
@NotNull
private Long outstandingPrincipal;
@NotNull
private Date loanTime;
@NotNull
private Boolean settleFlag;
@NotNull
private Date nearestRepayTime;
/**
* 逾期标识
* true:逾期
* false:未逾期 */
public Boolean getOverDueFlag() {
return this.overDueFlag;
}
/**
* 逾期标识
* true:逾期
* false:未逾期 */
public void setOverDueFlag(Boolean overDueFlag) {
this.overDueFlag = overDueFlag;
}
/**
* 逾期天数 */
public Long getOverDays() {
return this.overDays;
}
/**
* 逾期天数 */
public void setOverDays(Long overDays) {
this.overDays = overDays;
}
/**
* 逾期金额在50元以上的客户的逾期天数 */
public Long getValuableOverDays() {
return this.valuableOverDays;
}
/**
* 逾期金额在50元以上的客户的逾期天数 */
public void setValuableOverDays(Long valuableOverDays) {
this.valuableOverDays = valuableOverDays;
}
/**
* 逾期期数 */
public Long getOverPeriodCount() {
return this.overPeriodCount;
}
/**
* 逾期期数 */
public void setOverPeriodCount(Long overPeriodCount) {
this.overPeriodCount = overPeriodCount;
}
/**
* 逾期本金 */
public Long getOverPrincipal() {
return this.overPrincipal;
}
/**
* 逾期本金 */
public void setOverPrincipal(Long overPrincipal) {
this.overPrincipal = overPrincipal;
}
/**
* 逾期利息 */
public Long getOverInterest() {
return this.overInterest;
}
/**
* 逾期利息 */
public void setOverInterest(Long overInterest) {
this.overInterest = overInterest;
}
/**
* 应还罚息 */
public Long getOverPunish() {
return this.overPunish;
}
/**
* 应还罚息 */
public void setOverPunish(Long overPunish) {
this.overPunish = overPunish;
}
/**
* 应还逾期总额 */
public Long getNeedOverdueAmount() {
return this.needOverdueAmount;
}
/**
* 应还逾期总额 */
public void setNeedOverdueAmount(Long needOverdueAmount) {
this.needOverdueAmount = needOverdueAmount;
}
/**
* 当前应还总额 */
public Long getCurrentNeedAmount() {
return this.currentNeedAmount;
}
/**
* 当前应还总额 */
public void setCurrentNeedAmount(Long currentNeedAmount) {
this.currentNeedAmount = currentNeedAmount;
}
/**
* 总剩余应还 */
public Long getTotalAmount() {
return this.totalAmount;
}
/**
* 总剩余应还 */
public void setTotalAmount(Long totalAmount) {
this.totalAmount = totalAmount;
}
/**
* 数据日期 */
public Date getSettleDate() {
return this.settleDate;
}
/**
* 数据日期 */
public void setSettleDate(Date settleDate) {
this.settleDate = settleDate;
}
/**
* 借款唯一编号 */
public String getReceiptNo() {
return this.receiptNo;
}
/**
* 借款唯一编号 */
public void setReceiptNo(String receiptNo) {
this.receiptNo = receiptNo;
}
/**
* 已还期数 */
public Long getAlreadyRepayPeriodCount() {
return this.alreadyRepayPeriodCount;
}
/**
* 已还期数 */
public void setAlreadyRepayPeriodCount(Long alreadyRepayPeriodCount) {
this.alreadyRepayPeriodCount = alreadyRepayPeriodCount;
}
/**
* 贷款期数 */
public Long getLoanPeriodCount() {
return this.loanPeriodCount;
}
/**
* 贷款期数 */
public void setLoanPeriodCount(Long loanPeriodCount) {
this.loanPeriodCount = loanPeriodCount;
}
/**
* 未还本金 */
public Long getOutstandingPrincipal() {
return this.outstandingPrincipal;
}
/**
* 未还本金 */
public void setOutstandingPrincipal(Long outstandingPrincipal) {
this.outstandingPrincipal = outstandingPrincipal;
}
/**
* 放款日期 */
public Date getLoanTime() {
return this.loanTime;
}
/**
* 放款日期 */
public void setLoanTime(Date loanTime) {
this.loanTime = loanTime;
}
/**
* 结清标志 */
public Boolean getSettleFlag() {
return this.settleFlag;
}
/**
* 结清标志 */
public void setSettleFlag(Boolean settleFlag) {
this.settleFlag = settleFlag;
}
/**
* 最近一次还款日期 */
public Date getNearestRepayTime() {
return this.nearestRepayTime;
}
/**
* 最近一次还款日期 */
public void setNearestRepayTime(Date nearestRepayTime) {
this.nearestRepayTime = nearestRepayTime;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy