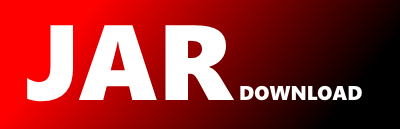
cn.com.antcloud.api.riskplus.v1_0.model.PersonalInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antcloud-api-riskplus Show documentation
Show all versions of antcloud-api-riskplus Show documentation
Ant Chain API SDK For Java
Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
//
//
package cn.com.antcloud.api.riskplus.v1_0.model;
import java.lang.String;
import javax.validation.constraints.NotNull;
/**
* 天枢系统个人信息结构体 */
public class PersonalInfo {
@NotNull
private String customName;
@NotNull
private String cardNo;
@NotNull
private String idType;
@NotNull
private String certSignDate;
@NotNull
private String certValidate;
@NotNull
private String certAdr;
@NotNull
private String mobile;
@NotNull
private String education;
private String province;
private String city;
private String area;
private String address;
private String sex;
private String nation;
private String maritalStatus;
/**
* 客户姓名 */
public String getCustomName() {
return this.customName;
}
/**
* 客户姓名 */
public void setCustomName(String customName) {
this.customName = customName;
}
/**
* 身份证号码(18位) */
public String getCardNo() {
return this.cardNo;
}
/**
* 身份证号码(18位) */
public void setCardNo(String cardNo) {
this.cardNo = cardNo;
}
/**
* 1-身份证 */
public String getIdType() {
return this.idType;
}
/**
* 1-身份证 */
public void setIdType(String idType) {
this.idType = idType;
}
/**
* 证件开始日期(格式:YYYY-MM-DD)
*/
public String getCertSignDate() {
return this.certSignDate;
}
/**
* 证件开始日期(格式:YYYY-MM-DD)
*/
public void setCertSignDate(String certSignDate) {
this.certSignDate = certSignDate;
}
/**
* 格式:YYYY-MM-DD,身份证有效期为长期的送: 9999-12-31 */
public String getCertValidate() {
return this.certValidate;
}
/**
* 格式:YYYY-MM-DD,身份证有效期为长期的送: 9999-12-31 */
public void setCertValidate(String certValidate) {
this.certValidate = certValidate;
}
/**
* 证件地址 */
public String getCertAdr() {
return this.certAdr;
}
/**
* 证件地址 */
public void setCertAdr(String certAdr) {
this.certAdr = certAdr;
}
/**
* 手机号 */
public String getMobile() {
return this.mobile;
}
/**
* 手机号 */
public void setMobile(String mobile) {
this.mobile = mobile;
}
/**
* 学历 */
public String getEducation() {
return this.education;
}
/**
* 学历 */
public void setEducation(String education) {
this.education = education;
}
/**
* 所在省份 汉字 */
public String getProvince() {
return this.province;
}
/**
* 所在省份 汉字 */
public void setProvince(String province) {
this.province = province;
}
/**
* 所在城市 汉字 */
public String getCity() {
return this.city;
}
/**
* 所在城市 汉字 */
public void setCity(String city) {
this.city = city;
}
/**
* 地区名称 汉字 */
public String getArea() {
return this.area;
}
/**
* 地区名称 汉字 */
public void setArea(String area) {
this.area = area;
}
/**
* 详细地址 */
public String getAddress() {
return this.address;
}
/**
* 详细地址 */
public void setAddress(String address) {
this.address = address;
}
/**
* 性别M-男
* F-女 */
public String getSex() {
return this.sex;
}
/**
* 性别M-男
* F-女 */
public void setSex(String sex) {
this.sex = sex;
}
/**
* 民族 */
public String getNation() {
return this.nation;
}
/**
* 民族 */
public void setNation(String nation) {
this.nation = nation;
}
/**
* 婚姻状态:00-未婚,01-已婚,02-离婚,03-丧偶,99-未知 */
public String getMaritalStatus() {
return this.maritalStatus;
}
/**
* 婚姻状态:00-未婚,01-已婚,02-离婚,03-丧偶,99-未知 */
public void setMaritalStatus(String maritalStatus) {
this.maritalStatus = maritalStatus;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy