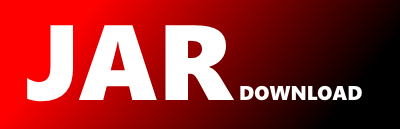
cn.com.antcloud.api.riskplus.v1_0.model.ReceiptInfo Maven / Gradle / Ivy
//
// Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
//
//
package cn.com.antcloud.api.riskplus.v1_0.model;
import java.lang.Long;
import java.lang.String;
import java.util.Date;
import javax.validation.constraints.NotNull;
/**
* 天枢系统专用ReceiptInfo结构体 */
public class ReceiptInfo {
@NotNull
private String customName;
@NotNull
private String cardNo;
@NotNull
private String mobile;
@NotNull
private Long applyAmount;
@NotNull
private Long loanAmount;
@NotNull
private Long period;
@NotNull
private Long curPeriod;
@NotNull
private String repayType;
@NotNull
private String repayDate;
@NotNull
private Date loanTime;
@NotNull
private String status;
@NotNull
private Long alreadyCorpus;
@NotNull
private Long alreadyAccrual;
@NotNull
private Date alreadyDate;
@NotNull
private String workflowStatus;
@NotNull
private String receiptNo;
/**
* 客户名 */
public String getCustomName() {
return this.customName;
}
/**
* 客户名 */
public void setCustomName(String customName) {
this.customName = customName;
}
/**
* 证件号码 */
public String getCardNo() {
return this.cardNo;
}
/**
* 证件号码 */
public void setCardNo(String cardNo) {
this.cardNo = cardNo;
}
/**
* 手机号 */
public String getMobile() {
return this.mobile;
}
/**
* 手机号 */
public void setMobile(String mobile) {
this.mobile = mobile;
}
/**
* 贷款金额 */
public Long getApplyAmount() {
return this.applyAmount;
}
/**
* 贷款金额 */
public void setApplyAmount(Long applyAmount) {
this.applyAmount = applyAmount;
}
/**
* 发放金额 */
public Long getLoanAmount() {
return this.loanAmount;
}
/**
* 发放金额 */
public void setLoanAmount(Long loanAmount) {
this.loanAmount = loanAmount;
}
/**
* 期数 */
public Long getPeriod() {
return this.period;
}
/**
* 期数 */
public void setPeriod(Long period) {
this.period = period;
}
/**
* 当前期数 */
public Long getCurPeriod() {
return this.curPeriod;
}
/**
* 当前期数 */
public void setCurPeriod(Long curPeriod) {
this.curPeriod = curPeriod;
}
/**
* 还款方式1:等额本息,2:等额本金,3:按月付息到期还本,4:利随本清,5:自由还款 */
public String getRepayType() {
return this.repayType;
}
/**
* 还款方式1:等额本息,2:等额本金,3:按月付息到期还本,4:利随本清,5:自由还款 */
public void setRepayType(String repayType) {
this.repayType = repayType;
}
/**
* 还款日 */
public String getRepayDate() {
return this.repayDate;
}
/**
* 还款日 */
public void setRepayDate(String repayDate) {
this.repayDate = repayDate;
}
/**
* 放款时间 */
public Date getLoanTime() {
return this.loanTime;
}
/**
* 放款时间 */
public void setLoanTime(Date loanTime) {
this.loanTime = loanTime;
}
/**
* 借据状态0:未还清,1:已还清,2:已提前还清 */
public String getStatus() {
return this.status;
}
/**
* 借据状态0:未还清,1:已还清,2:已提前还清 */
public void setStatus(String status) {
this.status = status;
}
/**
* 已还本金 */
public Long getAlreadyCorpus() {
return this.alreadyCorpus;
}
/**
* 已还本金 */
public void setAlreadyCorpus(Long alreadyCorpus) {
this.alreadyCorpus = alreadyCorpus;
}
/**
* 已还利息 */
public Long getAlreadyAccrual() {
return this.alreadyAccrual;
}
/**
* 已还利息 */
public void setAlreadyAccrual(Long alreadyAccrual) {
this.alreadyAccrual = alreadyAccrual;
}
/**
* 结清日期 */
public Date getAlreadyDate() {
return this.alreadyDate;
}
/**
* 结清日期 */
public void setAlreadyDate(Date alreadyDate) {
this.alreadyDate = alreadyDate;
}
/**
* 审批状态0:通过 1:拒绝 2:审批中 3:失败 */
public String getWorkflowStatus() {
return this.workflowStatus;
}
/**
* 审批状态0:通过 1:拒绝 2:审批中 3:失败 */
public void setWorkflowStatus(String workflowStatus) {
this.workflowStatus = workflowStatus;
}
/**
* 借据编号 */
public String getReceiptNo() {
return this.receiptNo;
}
/**
* 借据编号 */
public void setReceiptNo(String receiptNo) {
this.receiptNo = receiptNo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy