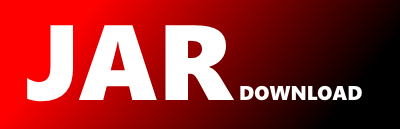
cn.com.antcloud.api.riskplus.v1_0.model.RepayResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antcloud-api-riskplus Show documentation
Show all versions of antcloud-api-riskplus Show documentation
Ant Chain API SDK For Java
Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
//
//
package cn.com.antcloud.api.riskplus.v1_0.model;
import java.lang.Long;
import java.lang.String;
import java.util.Date;
import javax.validation.constraints.NotNull;
/**
* 天枢系统专用RepayResult结构体 */
public class RepayResult {
@NotNull
private String customNo;
@NotNull
private String period;
@NotNull
private Long needAmount;
@NotNull
private Long needCorpus;
@NotNull
private Long needAccrual;
@NotNull
private Long needFee;
@NotNull
private Long alreadyAmount;
@NotNull
private Long alreadyCorpus;
@NotNull
private Long alreadyOvercorpus;
@NotNull
private Long alreadyAccrual;
@NotNull
private Long alreadyPunish;
@NotNull
private Long alreadyFee;
@NotNull
private Long rate;
@NotNull
private Long penaltyValue;
@NotNull
private Long restAmount;
@NotNull
private Long restCorpus;
@NotNull
private Long restAccrual;
@NotNull
private Long restPunish;
@NotNull
private Long remainCorpus;
@NotNull
private String receiptNo;
@NotNull
private String status;
@NotNull
private Date settleDate;
@NotNull
private Date tradeDate;
@NotNull
private Long alreadyGuaranteeFee;
@NotNull
private Long alreadyLiquidatedDamages;
@NotNull
private Long restGuaranteeFee;
@NotNull
private Long restLiquidatedDamages;
@NotNull
private Long needGuaranteeFee;
@NotNull
private Long needLiquidatedDamages;
/**
* 客户编码 */
public String getCustomNo() {
return this.customNo;
}
/**
* 客户编码 */
public void setCustomNo(String customNo) {
this.customNo = customNo;
}
/**
* 当前期数 */
public String getPeriod() {
return this.period;
}
/**
* 当前期数 */
public void setPeriod(String period) {
this.period = period;
}
/**
* 应还总额 */
public Long getNeedAmount() {
return this.needAmount;
}
/**
* 应还总额 */
public void setNeedAmount(Long needAmount) {
this.needAmount = needAmount;
}
/**
* 应还本金 */
public Long getNeedCorpus() {
return this.needCorpus;
}
/**
* 应还本金 */
public void setNeedCorpus(Long needCorpus) {
this.needCorpus = needCorpus;
}
/**
* 应还利息 */
public Long getNeedAccrual() {
return this.needAccrual;
}
/**
* 应还利息 */
public void setNeedAccrual(Long needAccrual) {
this.needAccrual = needAccrual;
}
/**
* 应还手续费 */
public Long getNeedFee() {
return this.needFee;
}
/**
* 应还手续费 */
public void setNeedFee(Long needFee) {
this.needFee = needFee;
}
/**
* 已还总额 */
public Long getAlreadyAmount() {
return this.alreadyAmount;
}
/**
* 已还总额 */
public void setAlreadyAmount(Long alreadyAmount) {
this.alreadyAmount = alreadyAmount;
}
/**
* 已还本金 */
public Long getAlreadyCorpus() {
return this.alreadyCorpus;
}
/**
* 已还本金 */
public void setAlreadyCorpus(Long alreadyCorpus) {
this.alreadyCorpus = alreadyCorpus;
}
/**
* 已还逾期本金 */
public Long getAlreadyOvercorpus() {
return this.alreadyOvercorpus;
}
/**
* 已还逾期本金 */
public void setAlreadyOvercorpus(Long alreadyOvercorpus) {
this.alreadyOvercorpus = alreadyOvercorpus;
}
/**
* 已还利息 */
public Long getAlreadyAccrual() {
return this.alreadyAccrual;
}
/**
* 已还利息 */
public void setAlreadyAccrual(Long alreadyAccrual) {
this.alreadyAccrual = alreadyAccrual;
}
/**
* 已还逾期息 */
public Long getAlreadyPunish() {
return this.alreadyPunish;
}
/**
* 已还逾期息 */
public void setAlreadyPunish(Long alreadyPunish) {
this.alreadyPunish = alreadyPunish;
}
/**
* 已还手续费 */
public Long getAlreadyFee() {
return this.alreadyFee;
}
/**
* 已还手续费 */
public void setAlreadyFee(Long alreadyFee) {
this.alreadyFee = alreadyFee;
}
/**
* 利率 */
public Long getRate() {
return this.rate;
}
/**
* 利率 */
public void setRate(Long rate) {
this.rate = rate;
}
/**
* 罚息率 */
public Long getPenaltyValue() {
return this.penaltyValue;
}
/**
* 罚息率 */
public void setPenaltyValue(Long penaltyValue) {
this.penaltyValue = penaltyValue;
}
/**
* 当期剩余总额 */
public Long getRestAmount() {
return this.restAmount;
}
/**
* 当期剩余总额 */
public void setRestAmount(Long restAmount) {
this.restAmount = restAmount;
}
/**
* 当期剩余本金 */
public Long getRestCorpus() {
return this.restCorpus;
}
/**
* 当期剩余本金 */
public void setRestCorpus(Long restCorpus) {
this.restCorpus = restCorpus;
}
/**
* 当期剩余利息 */
public Long getRestAccrual() {
return this.restAccrual;
}
/**
* 当期剩余利息 */
public void setRestAccrual(Long restAccrual) {
this.restAccrual = restAccrual;
}
/**
* 当期剩余罚息 */
public Long getRestPunish() {
return this.restPunish;
}
/**
* 当期剩余罚息 */
public void setRestPunish(Long restPunish) {
this.restPunish = restPunish;
}
/**
* 期末本金 */
public Long getRemainCorpus() {
return this.remainCorpus;
}
/**
* 期末本金 */
public void setRemainCorpus(Long remainCorpus) {
this.remainCorpus = remainCorpus;
}
/**
* 借据编号 */
public String getReceiptNo() {
return this.receiptNo;
}
/**
* 借据编号 */
public void setReceiptNo(String receiptNo) {
this.receiptNo = receiptNo;
}
/**
* 还款状态1:已还清 2 未还 3 部分还款 */
public String getStatus() {
return this.status;
}
/**
* 还款状态1:已还清 2 未还 3 部分还款 */
public void setStatus(String status) {
this.status = status;
}
/**
* 应还日期 */
public Date getSettleDate() {
return this.settleDate;
}
/**
* 应还日期 */
public void setSettleDate(Date settleDate) {
this.settleDate = settleDate;
}
/**
* 还款日期 */
public Date getTradeDate() {
return this.tradeDate;
}
/**
* 还款日期 */
public void setTradeDate(Date tradeDate) {
this.tradeDate = tradeDate;
}
/**
* 已还担保费 */
public Long getAlreadyGuaranteeFee() {
return this.alreadyGuaranteeFee;
}
/**
* 已还担保费 */
public void setAlreadyGuaranteeFee(Long alreadyGuaranteeFee) {
this.alreadyGuaranteeFee = alreadyGuaranteeFee;
}
/**
* 已还违约金 */
public Long getAlreadyLiquidatedDamages() {
return this.alreadyLiquidatedDamages;
}
/**
* 已还违约金 */
public void setAlreadyLiquidatedDamages(Long alreadyLiquidatedDamages) {
this.alreadyLiquidatedDamages = alreadyLiquidatedDamages;
}
/**
* 当期剩余担保费 */
public Long getRestGuaranteeFee() {
return this.restGuaranteeFee;
}
/**
* 当期剩余担保费 */
public void setRestGuaranteeFee(Long restGuaranteeFee) {
this.restGuaranteeFee = restGuaranteeFee;
}
/**
* 当期剩余违约金 */
public Long getRestLiquidatedDamages() {
return this.restLiquidatedDamages;
}
/**
* 当期剩余违约金 */
public void setRestLiquidatedDamages(Long restLiquidatedDamages) {
this.restLiquidatedDamages = restLiquidatedDamages;
}
/**
* 应还担保费 */
public Long getNeedGuaranteeFee() {
return this.needGuaranteeFee;
}
/**
* 应还担保费 */
public void setNeedGuaranteeFee(Long needGuaranteeFee) {
this.needGuaranteeFee = needGuaranteeFee;
}
/**
* 应还违约金 */
public Long getNeedLiquidatedDamages() {
return this.needLiquidatedDamages;
}
/**
* 应还违约金 */
public void setNeedLiquidatedDamages(Long needLiquidatedDamages) {
this.needLiquidatedDamages = needLiquidatedDamages;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy