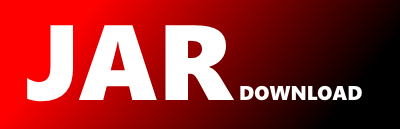
cn.com.antcloud.api.riskplus.v1_0.response.QueryCrowdriskDetailResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antcloud-api-riskplus Show documentation
Show all versions of antcloud-api-riskplus Show documentation
Ant Chain API SDK For Java
Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
//
//
package cn.com.antcloud.api.riskplus.v1_0.response;
import cn.com.antcloud.api.product.AntCloudProdResponse;
import cn.com.antcloud.api.riskplus.v1_0.model.RtopAgeDistribution;
import cn.com.antcloud.api.riskplus.v1_0.model.RtopCrowdRiskFeatureResp;
import cn.com.antcloud.api.riskplus.v1_0.model.RtopDateDistribution;
import cn.com.antcloud.api.riskplus.v1_0.model.RtopGenderDistribution;
import cn.com.antcloud.api.riskplus.v1_0.model.RtopPopulationDistribution;
import java.lang.Long;
import java.lang.String;
import java.util.Date;
import java.util.List;
/**
* 查询涉众风险企业的详细信息
*/
public class QueryCrowdriskDetailResponse extends AntCloudProdResponse {
private String activeCity;
private String activeCounty;
private String activeProvince;
private List ageDistribution;
private List clueTags;
private String conclusion;
private String crowdRiskLevel;
private Long crowdRiskScore;
private String crowdRiskType;
private Date detectedTime;
private List features;
private List genderDistribution;
private Date lastActiveTime;
private Long moneyInvolved;
private List moneyInvolvedHistory;
private Long peopleInvolved;
private List peopleInvolvedHistory;
private List populationDistribution;
private String registeredCity;
private String registeredCounty;
private String registeredProvince;
/**
* 活跃市 */
public String getActiveCity() {
return this.activeCity;
}
/**
* 活跃市 */
public void setActiveCity(String activeCity) {
this.activeCity = activeCity;
}
/**
* 活跃县 */
public String getActiveCounty() {
return this.activeCounty;
}
/**
* 活跃县 */
public void setActiveCounty(String activeCounty) {
this.activeCounty = activeCounty;
}
/**
* 活跃省 */
public String getActiveProvince() {
return this.activeProvince;
}
/**
* 活跃省 */
public void setActiveProvince(String activeProvince) {
this.activeProvince = activeProvince;
}
/**
* 年龄分布 */
public List getAgeDistribution() {
return this.ageDistribution;
}
/**
* 年龄分布 */
public void setAgeDistribution(List ageDistribution) {
this.ageDistribution = ageDistribution;
}
/**
* 线索标签 */
public List getClueTags() {
return this.clueTags;
}
/**
* 线索标签 */
public void setClueTags(List clueTags) {
this.clueTags = clueTags;
}
/**
* 报文 */
public String getConclusion() {
return this.conclusion;
}
/**
* 报文 */
public void setConclusion(String conclusion) {
this.conclusion = conclusion;
}
/**
* 风险等级 */
public String getCrowdRiskLevel() {
return this.crowdRiskLevel;
}
/**
* 风险等级 */
public void setCrowdRiskLevel(String crowdRiskLevel) {
this.crowdRiskLevel = crowdRiskLevel;
}
/**
* 涉众风险分析的分数 */
public Long getCrowdRiskScore() {
return this.crowdRiskScore;
}
/**
* 涉众风险分析的分数 */
public void setCrowdRiskScore(Long crowdRiskScore) {
this.crowdRiskScore = crowdRiskScore;
}
/**
* 风险类型 */
public String getCrowdRiskType() {
return this.crowdRiskType;
}
/**
* 风险类型 */
public void setCrowdRiskType(String crowdRiskType) {
this.crowdRiskType = crowdRiskType;
}
/**
* 发现时间 */
public Date getDetectedTime() {
return this.detectedTime;
}
/**
* 发现时间 */
public void setDetectedTime(Date detectedTime) {
this.detectedTime = detectedTime;
}
/**
* 特征 */
public List getFeatures() {
return this.features;
}
/**
* 特征 */
public void setFeatures(List features) {
this.features = features;
}
/**
* 性别分布 */
public List getGenderDistribution() {
return this.genderDistribution;
}
/**
* 性别分布 */
public void setGenderDistribution(List genderDistribution) {
this.genderDistribution = genderDistribution;
}
/**
* 上次活跃时间 */
public Date getLastActiveTime() {
return this.lastActiveTime;
}
/**
* 上次活跃时间 */
public void setLastActiveTime(Date lastActiveTime) {
this.lastActiveTime = lastActiveTime;
}
/**
* 涉案金额 */
public Long getMoneyInvolved() {
return this.moneyInvolved;
}
/**
* 涉案金额 */
public void setMoneyInvolved(Long moneyInvolved) {
this.moneyInvolved = moneyInvolved;
}
/**
* 影响金额的历史曲线 */
public List getMoneyInvolvedHistory() {
return this.moneyInvolvedHistory;
}
/**
* 影响金额的历史曲线 */
public void setMoneyInvolvedHistory(List moneyInvolvedHistory) {
this.moneyInvolvedHistory = moneyInvolvedHistory;
}
/**
* 涉案人数 */
public Long getPeopleInvolved() {
return this.peopleInvolved;
}
/**
* 涉案人数 */
public void setPeopleInvolved(Long peopleInvolved) {
this.peopleInvolved = peopleInvolved;
}
/**
* 影响人数的历史曲线 */
public List getPeopleInvolvedHistory() {
return this.peopleInvolvedHistory;
}
/**
* 影响人数的历史曲线 */
public void setPeopleInvolvedHistory(List peopleInvolvedHistory) {
this.peopleInvolvedHistory = peopleInvolvedHistory;
}
/**
* 影响的人数分布,key为地区城市,value为影响的人数 */
public List getPopulationDistribution() {
return this.populationDistribution;
}
/**
* 影响的人数分布,key为地区城市,value为影响的人数 */
public void setPopulationDistribution(List populationDistribution) {
this.populationDistribution = populationDistribution;
}
/**
* 注册市 */
public String getRegisteredCity() {
return this.registeredCity;
}
/**
* 注册市 */
public void setRegisteredCity(String registeredCity) {
this.registeredCity = registeredCity;
}
/**
* 注册县 */
public String getRegisteredCounty() {
return this.registeredCounty;
}
/**
* 注册县 */
public void setRegisteredCounty(String registeredCounty) {
this.registeredCounty = registeredCounty;
}
/**
* 注册省 */
public String getRegisteredProvince() {
return this.registeredProvince;
}
/**
* 注册省 */
public void setRegisteredProvince(String registeredProvince) {
this.registeredProvince = registeredProvince;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy