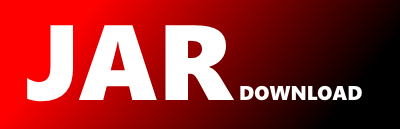
cn.com.antcloud.api.riskplus.v1_0.model.CommonRobotCallDetail Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antcloud-api-riskplus Show documentation
Show all versions of antcloud-api-riskplus Show documentation
Ant Chain API SDK For Java
Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
//
//
package cn.com.antcloud.api.riskplus.v1_0.model;
import java.lang.Long;
import java.lang.String;
import javax.validation.constraints.NotNull;
/**
* 营销盾外呼记录 */
public class CommonRobotCallDetail {
@NotNull
private String extInfo;
@NotNull
private String resultCode;
@NotNull
private String customerKey;
@NotNull
private Long currentCallTimes;
@NotNull
private String keyTemplate;
@NotNull
private String batchId;
@NotNull
private Long callType;
private String tag;
@NotNull
private String callId;
@NotNull
private Long taskId;
private Long templateId;
@NotNull
private Long statusCode;
@NotNull
private String statusDescription;
@NotNull
private Long transferStatusCode;
@NotNull
private String transferStatus;
private Long agentId;
private String agentTag;
private String agentExtension;
@NotNull
private String importTime;
@NotNull
private String callBeginTime;
@NotNull
private Long ringTime;
private String answerTime;
@NotNull
private String speakingTime;
@NotNull
private Long speakingDuration;
@NotNull
private String hangupTime;
@NotNull
private Long speakingTurns;
@NotNull
private String agentSpeakingTime;
@NotNull
private Long agentSpeakingDuration;
@NotNull
private String intentTag;
@NotNull
private String intentDescription;
private String individualTag;
private String keywords;
@NotNull
private Long hungupType;
@NotNull
private String sms;
private String chatRecord;
private String chats;
private Long addWx;
private String addWxStatus;
@NotNull
private Long answerRecall;
private String properties;
private String bizProperties;
private String interceptReason;
/**
* 客户请求时的透传字段 */
public String getExtInfo() {
return this.extInfo;
}
/**
* 客户请求时的透传字段 */
public void setExtInfo(String extInfo) {
this.extInfo = extInfo;
}
/**
* 成功触达:OK;未触达:AI_ROBOT_CALL_REQUEST_NOT_EXIST */
public String getResultCode() {
return this.resultCode;
}
/**
* 成功触达:OK;未触达:AI_ROBOT_CALL_REQUEST_NOT_EXIST */
public void setResultCode(String resultCode) {
this.resultCode = resultCode;
}
/**
* 外呼号码 */
public String getCustomerKey() {
return this.customerKey;
}
/**
* 外呼号码 */
public void setCustomerKey(String customerKey) {
this.customerKey = customerKey;
}
/**
* 呼叫次数 */
public Long getCurrentCallTimes() {
return this.currentCallTimes;
}
/**
* 呼叫次数 */
public void setCurrentCallTimes(Long currentCallTimes) {
this.currentCallTimes = currentCallTimes;
}
/**
* 号码模版 */
public String getKeyTemplate() {
return this.keyTemplate;
}
/**
* 号码模版 */
public void setKeyTemplate(String keyTemplate) {
this.keyTemplate = keyTemplate;
}
/**
* 导入号码时返回的批次号 */
public String getBatchId() {
return this.batchId;
}
/**
* 导入号码时返回的批次号 */
public void setBatchId(String batchId) {
this.batchId = batchId;
}
/**
* 2001:批量-预测外呼,2002:批量-AI外呼-不转人工,2003:批量-AI外呼-接通转人工,2004: 批量-AI外呼-智能转人工,2005:批量-语音通知 */
public Long getCallType() {
return this.callType;
}
/**
* 2001:批量-预测外呼,2002:批量-AI外呼-不转人工,2003:批量-AI外呼-接通转人工,2004: 批量-AI外呼-智能转人工,2005:批量-语音通知 */
public void setCallType(Long callType) {
this.callType = callType;
}
/**
* 用户自定义标签 */
public String getTag() {
return this.tag;
}
/**
* 用户自定义标签 */
public void setTag(String tag) {
this.tag = tag;
}
/**
* 外呼id */
public String getCallId() {
return this.callId;
}
/**
* 外呼id */
public void setCallId(String callId) {
this.callId = callId;
}
/**
* 外呼任务编号 */
public Long getTaskId() {
return this.taskId;
}
/**
* 外呼任务编号 */
public void setTaskId(Long taskId) {
this.taskId = taskId;
}
/**
* AI话术ID */
public Long getTemplateId() {
return this.templateId;
}
/**
* AI话术ID */
public void setTemplateId(Long templateId) {
this.templateId = templateId;
}
/**
* 外呼状态编码 */
public Long getStatusCode() {
return this.statusCode;
}
/**
* 外呼状态编码 */
public void setStatusCode(Long statusCode) {
this.statusCode = statusCode;
}
/**
* 外呼状态描述 */
public String getStatusDescription() {
return this.statusDescription;
}
/**
* 外呼状态描述 */
public void setStatusDescription(String statusDescription) {
this.statusDescription = statusDescription;
}
/**
* 转人工状态编码 */
public Long getTransferStatusCode() {
return this.transferStatusCode;
}
/**
* 转人工状态编码 */
public void setTransferStatusCode(Long transferStatusCode) {
this.transferStatusCode = transferStatusCode;
}
/**
* 转人工状态 */
public String getTransferStatus() {
return this.transferStatus;
}
/**
* 转人工状态 */
public void setTransferStatus(String transferStatus) {
this.transferStatus = transferStatus;
}
/**
* 分配坐席ID */
public Long getAgentId() {
return this.agentId;
}
/**
* 分配坐席ID */
public void setAgentId(Long agentId) {
this.agentId = agentId;
}
/**
* 坐席在贵司业务系统唯一标识,用于查询对应agentId;可以为空。 */
public String getAgentTag() {
return this.agentTag;
}
/**
* 坐席在贵司业务系统唯一标识,用于查询对应agentId;可以为空。 */
public void setAgentTag(String agentTag) {
this.agentTag = agentTag;
}
/**
* 坐席分机号 */
public String getAgentExtension() {
return this.agentExtension;
}
/**
* 坐席分机号 */
public void setAgentExtension(String agentExtension) {
this.agentExtension = agentExtension;
}
/**
* 导入时间 */
public String getImportTime() {
return this.importTime;
}
/**
* 导入时间 */
public void setImportTime(String importTime) {
this.importTime = importTime;
}
/**
* 开始通话时间 */
public String getCallBeginTime() {
return this.callBeginTime;
}
/**
* 开始通话时间 */
public void setCallBeginTime(String callBeginTime) {
this.callBeginTime = callBeginTime;
}
/**
* 振铃时长,单位ms */
public Long getRingTime() {
return this.ringTime;
}
/**
* 振铃时长,单位ms */
public void setRingTime(Long ringTime) {
this.ringTime = ringTime;
}
/**
* 接通时间 */
public String getAnswerTime() {
return this.answerTime;
}
/**
* 接通时间 */
public void setAnswerTime(String answerTime) {
this.answerTime = answerTime;
}
/**
* 通话时长,单位:大于1分钟,显示分钟秒,小于1分钟,显示秒 */
public String getSpeakingTime() {
return this.speakingTime;
}
/**
* 通话时长,单位:大于1分钟,显示分钟秒,小于1分钟,显示秒 */
public void setSpeakingTime(String speakingTime) {
this.speakingTime = speakingTime;
}
/**
* 通话时长,单位:秒 */
public Long getSpeakingDuration() {
return this.speakingDuration;
}
/**
* 通话时长,单位:秒 */
public void setSpeakingDuration(Long speakingDuration) {
this.speakingDuration = speakingDuration;
}
/**
* 挂断时间 */
public String getHangupTime() {
return this.hangupTime;
}
/**
* 挂断时间 */
public void setHangupTime(String hangupTime) {
this.hangupTime = hangupTime;
}
/**
* 对话轮次 */
public Long getSpeakingTurns() {
return this.speakingTurns;
}
/**
* 对话轮次 */
public void setSpeakingTurns(Long speakingTurns) {
this.speakingTurns = speakingTurns;
}
/**
* 人工通话时长,单位:大于1分钟,显示分钟秒,小于1分钟,显示秒 */
public String getAgentSpeakingTime() {
return this.agentSpeakingTime;
}
/**
* 人工通话时长,单位:大于1分钟,显示分钟秒,小于1分钟,显示秒 */
public void setAgentSpeakingTime(String agentSpeakingTime) {
this.agentSpeakingTime = agentSpeakingTime;
}
/**
* 人工通话时长,单位:秒 */
public Long getAgentSpeakingDuration() {
return this.agentSpeakingDuration;
}
/**
* 人工通话时长,单位:秒 */
public void setAgentSpeakingDuration(Long agentSpeakingDuration) {
this.agentSpeakingDuration = agentSpeakingDuration;
}
/**
* 意向标签 */
public String getIntentTag() {
return this.intentTag;
}
/**
* 意向标签 */
public void setIntentTag(String intentTag) {
this.intentTag = intentTag;
}
/**
* 意向说明 */
public String getIntentDescription() {
return this.intentDescription;
}
/**
* 意向说明 */
public void setIntentDescription(String intentDescription) {
this.intentDescription = intentDescription;
}
/**
* 个性标签 */
public String getIndividualTag() {
return this.individualTag;
}
/**
* 个性标签 */
public void setIndividualTag(String individualTag) {
this.individualTag = individualTag;
}
/**
* 回复关键词 */
public String getKeywords() {
return this.keywords;
}
/**
* 回复关键词 */
public void setKeywords(String keywords) {
this.keywords = keywords;
}
/**
* 挂机方式,AI挂机1,坐席挂机2,客户挂机3 */
public Long getHungupType() {
return this.hungupType;
}
/**
* 挂机方式,AI挂机1,坐席挂机2,客户挂机3 */
public void setHungupType(Long hungupType) {
this.hungupType = hungupType;
}
/**
* 挂机短信,可选值:1、2
* 1:发送,2:不发送 */
public String getSms() {
return this.sms;
}
/**
* 挂机短信,可选值:1、2
* 1:发送,2:不发送 */
public void setSms(String sms) {
this.sms = sms;
}
/**
* 对话录音,URL,可以为空 */
public String getChatRecord() {
return this.chatRecord;
}
/**
* 对话录音,URL,可以为空 */
public void setChatRecord(String chatRecord) {
this.chatRecord = chatRecord;
}
/**
* 聊天记录,可以为空 */
public String getChats() {
return this.chats;
}
/**
* 聊天记录,可以为空 */
public void setChats(String chats) {
this.chats = chats;
}
/**
* 可选值:0、1
* 0:不添加,1:添加 */
public Long getAddWx() {
return this.addWx;
}
/**
* 可选值:0、1
* 0:不添加,1:添加 */
public void setAddWx(Long addWx) {
this.addWx = addWx;
}
/**
* 加微进度,可选值:已申请、加微成功 */
public String getAddWxStatus() {
return this.addWxStatus;
}
/**
* 加微进度,可选值:已申请、加微成功 */
public void setAddWxStatus(String addWxStatus) {
this.addWxStatus = addWxStatus;
}
/**
* 是否接通重呼,可选值:0、1
* 0正常外呼,1接通重呼 */
public Long getAnswerRecall() {
return this.answerRecall;
}
/**
* 是否接通重呼,可选值:0、1
* 0正常外呼,1接通重呼 */
public void setAnswerRecall(Long answerRecall) {
this.answerRecall = answerRecall;
}
/**
* 导入号码时的参数值 */
public String getProperties() {
return this.properties;
}
/**
* 导入号码时的参数值 */
public void setProperties(String properties) {
this.properties = properties;
}
/**
* 导入号码时的业务参数值,原样返回 */
public String getBizProperties() {
return this.bizProperties;
}
/**
* 导入号码时的业务参数值,原样返回 */
public void setBizProperties(String bizProperties) {
this.bizProperties = bizProperties;
}
/**
* 拦截原因:当状态为已拦截时,可选值:黑名单拦截,灰名单拦截,异常号码拦截 */
public String getInterceptReason() {
return this.interceptReason;
}
/**
* 拦截原因:当状态为已拦截时,可选值:黑名单拦截,灰名单拦截,异常号码拦截 */
public void setInterceptReason(String interceptReason) {
this.interceptReason = interceptReason;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy