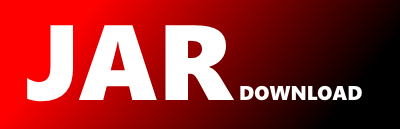
cn.com.antcloud.api.riskplus.v1_0.model.RiskLabelInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antcloud-api-riskplus Show documentation
Show all versions of antcloud-api-riskplus Show documentation
Ant Chain API SDK For Java
Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
//
//
package cn.com.antcloud.api.riskplus.v1_0.model;
import java.lang.Long;
import java.lang.String;
import javax.validation.constraints.NotNull;
/**
* 标签信息 */
public class RiskLabelInfo {
@NotNull
private String clueDetailType;
private String dt;
@NotNull
private String gmtCreate;
@NotNull
private Long id;
@NotNull
private Long isDeleted;
@NotNull
private String mctOneId;
@NotNull
private String operatorId;
@NotNull
private String opType;
@NotNull
private String orgName;
@NotNull
private String riskDetailType;
@NotNull
private String riskDimensionType;
@NotNull
private String tagClue;
@NotNull
private String tagClueDetail;
@NotNull
private String tagId;
@NotNull
private String tagListHeaders;
@NotNull
private String tagListOrderColumn;
@NotNull
private String tagListOrderType;
@NotNull
private String tagText;
@NotNull
private String tagTrendChartName;
@NotNull
private String updateDate;
/**
* 线索明细类型(字段停用) */
public String getClueDetailType() {
return this.clueDetailType;
}
/**
* 线索明细类型(字段停用) */
public void setClueDetailType(String clueDetailType) {
this.clueDetailType = clueDetailType;
}
/**
* odps数据产出时间,冗余字段,业务上不需要,以备错误排查 */
public String getDt() {
return this.dt;
}
/**
* odps数据产出时间,冗余字段,业务上不需要,以备错误排查 */
public void setDt(String dt) {
this.dt = dt;
}
/**
* 数据产生时间 */
public String getGmtCreate() {
return this.gmtCreate;
}
/**
* 数据产生时间 */
public void setGmtCreate(String gmtCreate) {
this.gmtCreate = gmtCreate;
}
/**
* 记录唯一ID */
public Long getId() {
return this.id;
}
/**
* 记录唯一ID */
public void setId(Long id) {
this.id = id;
}
/**
* 0-正常 1-删除 */
public Long getIsDeleted() {
return this.isDeleted;
}
/**
* 0-正常 1-删除 */
public void setIsDeleted(Long isDeleted) {
this.isDeleted = isDeleted;
}
/**
* 企业ID */
public String getMctOneId() {
return this.mctOneId;
}
/**
* 企业ID */
public void setMctOneId(String mctOneId) {
this.mctOneId = mctOneId;
}
/**
* 操作人ID */
public String getOperatorId() {
return this.operatorId;
}
/**
* 操作人ID */
public void setOperatorId(String operatorId) {
this.operatorId = operatorId;
}
/**
* 操作类型
* add、delete、update */
public String getOpType() {
return this.opType;
}
/**
* 操作类型
* add、delete、update */
public void setOpType(String opType) {
this.opType = opType;
}
/**
* 企业名称 */
public String getOrgName() {
return this.orgName;
}
/**
* 企业名称 */
public void setOrgName(String orgName) {
this.orgName = orgName;
}
/**
* 线索类型 */
public String getRiskDetailType() {
return this.riskDetailType;
}
/**
* 线索类型 */
public void setRiskDetailType(String riskDetailType) {
this.riskDetailType = riskDetailType;
}
/**
* 风险维度
*/
public String getRiskDimensionType() {
return this.riskDimensionType;
}
/**
* 风险维度
*/
public void setRiskDimensionType(String riskDimensionType) {
this.riskDimensionType = riskDimensionType;
}
/**
* 线索概览 */
public String getTagClue() {
return this.tagClue;
}
/**
* 线索概览 */
public void setTagClue(String tagClue) {
this.tagClue = tagClue;
}
/**
* 线索明细 */
public String getTagClueDetail() {
return this.tagClueDetail;
}
/**
* 线索明细 */
public void setTagClueDetail(String tagClueDetail) {
this.tagClueDetail = tagClueDetail;
}
/**
* 标签ID */
public String getTagId() {
return this.tagId;
}
/**
* 标签ID */
public void setTagId(String tagId) {
this.tagId = tagId;
}
/**
* 线索列表表头,英文逗号分隔
*/
public String getTagListHeaders() {
return this.tagListHeaders;
}
/**
* 线索列表表头,英文逗号分隔
*/
public void setTagListHeaders(String tagListHeaders) {
this.tagListHeaders = tagListHeaders;
}
/**
* 标签列表,排序字段 */
public String getTagListOrderColumn() {
return this.tagListOrderColumn;
}
/**
* 标签列表,排序字段 */
public void setTagListOrderColumn(String tagListOrderColumn) {
this.tagListOrderColumn = tagListOrderColumn;
}
/**
* 标签列表排序方式 */
public String getTagListOrderType() {
return this.tagListOrderType;
}
/**
* 标签列表排序方式 */
public void setTagListOrderType(String tagListOrderType) {
this.tagListOrderType = tagListOrderType;
}
/**
* 标签文本 */
public String getTagText() {
return this.tagText;
}
/**
* 标签文本 */
public void setTagText(String tagText) {
this.tagText = tagText;
}
/**
* 趋势图表名 */
public String getTagTrendChartName() {
return this.tagTrendChartName;
}
/**
* 趋势图表名 */
public void setTagTrendChartName(String tagTrendChartName) {
this.tagTrendChartName = tagTrendChartName;
}
/**
* 数据同步到公有云时间(业务上赋值当天) */
public String getUpdateDate() {
return this.updateDate;
}
/**
* 数据同步到公有云时间(业务上赋值当天) */
public void setUpdateDate(String updateDate) {
this.updateDate = updateDate;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy