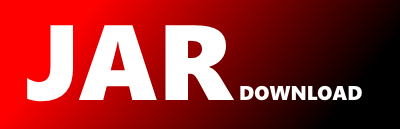
cn.com.antcloud.api.riskplus.v1_0.request.ApplyUsecreditRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antcloud-api-riskplus Show documentation
Show all versions of antcloud-api-riskplus Show documentation
Ant Chain API SDK For Java
Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
//
//
package cn.com.antcloud.api.riskplus.v1_0.request;
import cn.com.antcloud.api.product.AntCloudProdRequest;
import cn.com.antcloud.api.riskplus.v1_0.response.ApplyUsecreditResponse;
import java.lang.Long;
import java.lang.String;
import javax.validation.constraints.NotNull;
/**
* 天枢系统用信申请接口 */
public class ApplyUsecreditRequest extends AntCloudProdRequest {
@NotNull
private String originalOrderNo;
@NotNull
private Long loanAmount;
@NotNull
private Long period;
@NotNull
private String openId;
@NotNull
private String orderNo;
@NotNull
private String repayType;
@NotNull
private String loanWay;
private String repayDate;
private String channelType;
private String customType;
private String riskData;
private String loanInstCode;
private String bankCardNo;
public ApplyUsecreditRequest(String productInstanceId) {
super("riskplus.dubbridge.usecredit.apply", "1.0", "Java-SDK-20240605", productInstanceId);
}
public ApplyUsecreditRequest() {
super("riskplus.dubbridge.usecredit.apply", "1.0", null);
this.setSdkVersion("Java-SDK-20240605");
}
/**
* 授信申请订单号 */
public String getOriginalOrderNo() {
return this.originalOrderNo;
}
/**
* 授信申请订单号 */
public void setOriginalOrderNo(String originalOrderNo) {
this.originalOrderNo = originalOrderNo;
}
/**
* 用信金额 */
public Long getLoanAmount() {
return this.loanAmount;
}
/**
* 用信金额 */
public void setLoanAmount(Long loanAmount) {
this.loanAmount = loanAmount;
}
/**
* 期数 */
public Long getPeriod() {
return this.period;
}
/**
* 期数 */
public void setPeriod(Long period) {
this.period = period;
}
/**
* 资产方用户唯一标识 */
public String getOpenId() {
return this.openId;
}
/**
* 资产方用户唯一标识 */
public void setOpenId(String openId) {
this.openId = openId;
}
/**
* 订单号 */
public String getOrderNo() {
return this.orderNo;
}
/**
* 订单号 */
public void setOrderNo(String orderNo) {
this.orderNo = orderNo;
}
/**
* 还款方式:1:等额本息,2:等额本金 */
public String getRepayType() {
return this.repayType;
}
/**
* 还款方式:1:等额本息,2:等额本金 */
public void setRepayType(String repayType) {
this.repayType = repayType;
}
/**
* 1:手机数码 2:旅游 3:装修 4:教育 5:婚庆 6:租房 7:家具家居 8:健康医疗 9:其他消费 10:家用电器 */
public String getLoanWay() {
return this.loanWay;
}
/**
* 1:手机数码 2:旅游 3:装修 4:教育 5:婚庆 6:租房 7:家具家居 8:健康医疗 9:其他消费 10:家用电器 */
public void setLoanWay(String loanWay) {
this.loanWay = loanWay;
}
/**
* 还款日 */
public String getRepayDate() {
return this.repayDate;
}
/**
* 还款日 */
public void setRepayDate(String repayDate) {
this.repayDate = repayDate;
}
/**
* 渠道类型 */
public String getChannelType() {
return this.channelType;
}
/**
* 渠道类型 */
public void setChannelType(String channelType) {
this.channelType = channelType;
}
/**
* 客户类型 */
public String getCustomType() {
return this.customType;
}
/**
* 客户类型 */
public void setCustomType(String customType) {
this.customType = customType;
}
/**
* 风险数据对象(json字符串) */
public String getRiskData() {
return this.riskData;
}
/**
* 风险数据对象(json字符串) */
public void setRiskData(String riskData) {
this.riskData = riskData;
}
/**
* 资金源编码 */
public String getLoanInstCode() {
return this.loanInstCode;
}
/**
* 资金源编码 */
public void setLoanInstCode(String loanInstCode) {
this.loanInstCode = loanInstCode;
}
/**
* 银行卡号 */
public String getBankCardNo() {
return this.bankCardNo;
}
/**
* 银行卡号 */
public void setBankCardNo(String bankCardNo) {
this.bankCardNo = bankCardNo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy