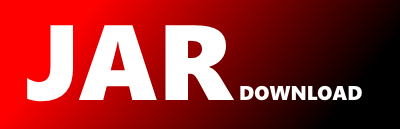
cn.com.antcloud.api.riskplus.v1_0.response.QueryRepayInfoResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antcloud-api-riskplus Show documentation
Show all versions of antcloud-api-riskplus Show documentation
Ant Chain API SDK For Java
Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Copyright (c) 2020-present antgroup.com, https://www.antgroup.com
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
//
//
package cn.com.antcloud.api.riskplus.v1_0.response;
import cn.com.antcloud.api.product.AntCloudProdResponse;
import java.lang.Long;
import java.lang.String;
/**
* 天枢系统还款信息查询 */
public class QueryRepayInfoResponse extends AntCloudProdResponse {
private String repayNo;
private String receiptNo;
private String customNo;
private String customName;
private String repayType;
private String repaySign;
private String repayDate;
private Long repayAmount;
private Long repayPrincipal;
private Long repayInterest;
private Long channelAmt;
private String repayFee;
private Long repayPunish;
private String repayAccount;
private String repayAccountName;
private String repayMobile;
private String repayBankNo;
private String repayBankName;
private String repayStatus;
private String failReason;
private String applyNo;
private Long guaranteeFee;
private Long liquidatedDamages;
/**
* 还款编号 */
public String getRepayNo() {
return this.repayNo;
}
/**
* 还款编号 */
public void setRepayNo(String repayNo) {
this.repayNo = repayNo;
}
/**
* 借据编码 */
public String getReceiptNo() {
return this.receiptNo;
}
/**
* 借据编码 */
public void setReceiptNo(String receiptNo) {
this.receiptNo = receiptNo;
}
/**
* 客户编号 */
public String getCustomNo() {
return this.customNo;
}
/**
* 客户编号 */
public void setCustomNo(String customNo) {
this.customNo = customNo;
}
/**
* 客户名称 */
public String getCustomName() {
return this.customName;
}
/**
* 客户名称 */
public void setCustomName(String customName) {
this.customName = customName;
}
/**
* 还款类型1:提前还款,2:正常还款 3:批量还款 4:自由还款 */
public String getRepayType() {
return this.repayType;
}
/**
* 还款类型1:提前还款,2:正常还款 3:批量还款 4:自由还款 */
public void setRepayType(String repayType) {
this.repayType = repayType;
}
/**
* repay_sign */
public String getRepaySign() {
return this.repaySign;
}
/**
* repay_sign */
public void setRepaySign(String repaySign) {
this.repaySign = repaySign;
}
/**
* 还款日期 */
public String getRepayDate() {
return this.repayDate;
}
/**
* 还款日期 */
public void setRepayDate(String repayDate) {
this.repayDate = repayDate;
}
/**
* 实还总额 */
public Long getRepayAmount() {
return this.repayAmount;
}
/**
* 实还总额 */
public void setRepayAmount(Long repayAmount) {
this.repayAmount = repayAmount;
}
/**
* 实还本金 */
public Long getRepayPrincipal() {
return this.repayPrincipal;
}
/**
* 实还本金 */
public void setRepayPrincipal(Long repayPrincipal) {
this.repayPrincipal = repayPrincipal;
}
/**
* 实还利息 */
public Long getRepayInterest() {
return this.repayInterest;
}
/**
* 实还利息 */
public void setRepayInterest(Long repayInterest) {
this.repayInterest = repayInterest;
}
/**
* 实还通道手续费 */
public Long getChannelAmt() {
return this.channelAmt;
}
/**
* 实还通道手续费 */
public void setChannelAmt(Long channelAmt) {
this.channelAmt = channelAmt;
}
/**
* 实还手续费 */
public String getRepayFee() {
return this.repayFee;
}
/**
* 实还手续费 */
public void setRepayFee(String repayFee) {
this.repayFee = repayFee;
}
/**
* 实收罚息 */
public Long getRepayPunish() {
return this.repayPunish;
}
/**
* 实收罚息 */
public void setRepayPunish(Long repayPunish) {
this.repayPunish = repayPunish;
}
/**
* 还款账户 */
public String getRepayAccount() {
return this.repayAccount;
}
/**
* 还款账户 */
public void setRepayAccount(String repayAccount) {
this.repayAccount = repayAccount;
}
/**
* 还款账户名称 */
public String getRepayAccountName() {
return this.repayAccountName;
}
/**
* 还款账户名称 */
public void setRepayAccountName(String repayAccountName) {
this.repayAccountName = repayAccountName;
}
/**
* 还款账户的手机号 */
public String getRepayMobile() {
return this.repayMobile;
}
/**
* 还款账户的手机号 */
public void setRepayMobile(String repayMobile) {
this.repayMobile = repayMobile;
}
/**
* 还款账户银行行号 */
public String getRepayBankNo() {
return this.repayBankNo;
}
/**
* 还款账户银行行号 */
public void setRepayBankNo(String repayBankNo) {
this.repayBankNo = repayBankNo;
}
/**
* 还款账户银行名称 */
public String getRepayBankName() {
return this.repayBankName;
}
/**
* 还款账户银行名称 */
public void setRepayBankName(String repayBankName) {
this.repayBankName = repayBankName;
}
/**
* 还款状态0:失败 1成功 2-审批中 3-还款中 */
public String getRepayStatus() {
return this.repayStatus;
}
/**
* 还款状态0:失败 1成功 2-审批中 3-还款中 */
public void setRepayStatus(String repayStatus) {
this.repayStatus = repayStatus;
}
/**
* 失败原因 */
public String getFailReason() {
return this.failReason;
}
/**
* 失败原因 */
public void setFailReason(String failReason) {
this.failReason = failReason;
}
/**
* 授信申请编号 */
public String getApplyNo() {
return this.applyNo;
}
/**
* 授信申请编号 */
public void setApplyNo(String applyNo) {
this.applyNo = applyNo;
}
/**
* 担保费 */
public Long getGuaranteeFee() {
return this.guaranteeFee;
}
/**
* 担保费 */
public void setGuaranteeFee(Long guaranteeFee) {
this.guaranteeFee = guaranteeFee;
}
/**
* 违约金 */
public Long getLiquidatedDamages() {
return this.liquidatedDamages;
}
/**
* 违约金 */
public void setLiquidatedDamages(Long liquidatedDamages) {
this.liquidatedDamages = liquidatedDamages;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy