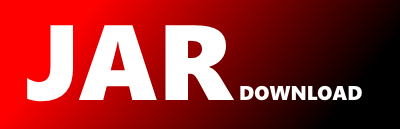
cn.com.antcloud.api.acapi.AntCloudWebUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antcloud-api-sdk Show documentation
Show all versions of antcloud-api-sdk Show documentation
Ant Fin Tech API SDK For Java
Copyright (c) 2015-present Alipay.com, https://www.alipay.com
The newest version!
/*
* Copyright (c) 2015-present Alipay.com, https://www.alipay.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package cn.com.antcloud.api.acapi;
import cn.com.antcloud.api.rest.RestHttpMethod;
import javax.net.ssl.*;
import java.io.*;
import java.net.*;
import java.security.SecureRandom;
import java.security.cert.CertificateException;
import java.security.cert.X509Certificate;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
public abstract class AntCloudWebUtils {
private static final String DEFAULT_CHARSET = Constants.CHARSET_UTF8;
private static final String METHOD_POST = "POST";
private static final String METHOD_GET = "GET";
private AntCloudWebUtils() {
}
public static String doRestRequest(String url, RestHttpMethod method, Map headers, Map queryParams,
String postBody, int connectTimeout, int readTimeout, Proxy proxy) throws IOException {
// content type
String ctype = null;
if (method.hasPostBody()) {
ctype = "application/json;charset=UTF-8";
}
// url with query string
if (queryParams != null && !queryParams.isEmpty()) {
url = url + "?" + buildQuery(queryParams, DEFAULT_CHARSET, true);
}
// http post content
byte[] content = {};
if (method.hasPostBody() && postBody != null) {
content = postBody.getBytes(DEFAULT_CHARSET);
}
HttpURLConnection conn = null;
OutputStream out = null;
String rsp;
try {
conn = getConnection(new URL(url), method.getCode(), ctype, headers, proxy);
conn.setConnectTimeout(connectTimeout);
conn.setReadTimeout(readTimeout);
if (method.hasPostBody()) {
out = conn.getOutputStream();
out.write(content);
}
rsp = getResponseAsString(conn);
} finally {
if (out != null) {
out.close();
}
if (conn != null) {
conn.disconnect();
}
}
return rsp;
}
public static String doRestRequest(String url, RestHttpMethod method, Map headers, Map queryParams,
String postBody, int connectTimeout, int readTimeout) throws IOException {
return doRestRequest(url, method, headers, queryParams, postBody, connectTimeout, readTimeout, null);
}
public static String doPost(String url, Map params, int connectTimeout,
int readTimeout, Proxy proxy) throws IOException {
return doPost(url, params, DEFAULT_CHARSET, connectTimeout, readTimeout, proxy);
}
public static String doPost(String url, Map params, String charset,
int connectTimeout, int readTimeout, Proxy proxy) throws IOException {
return doPost(url, params, charset, connectTimeout, readTimeout, null, proxy);
}
public static String doPost(String url, Map params, int connectTimeout,
int readTimeout) throws IOException {
return doPost(url, params, DEFAULT_CHARSET, connectTimeout, readTimeout, Proxy.NO_PROXY);
}
public static String doPost(String url, Map params, String charset,
int connectTimeout, int readTimeout) throws IOException {
return doPost(url, params, charset, connectTimeout, readTimeout, Proxy.NO_PROXY);
}
public static String doPost(String url, Map params, String charset,
int connectTimeout, int readTimeout, Map headerMap, Proxy proxy)
throws IOException {
String ctype = "application/x-www-form-urlencoded;charset=" + charset;
String query = buildQuery(params, charset, false);
byte[] content = {};
if (query != null) {
content = query.getBytes(charset);
}
return _doPost(url, ctype, content, connectTimeout, readTimeout, headerMap, proxy);
}
public static String doPost(String url, Map params, String charset,
int connectTimeout, int readTimeout, Map headerMap)
throws IOException {
return doPost(url, params, charset, connectTimeout, readTimeout, headerMap, null);
}
@Deprecated
public static String doPost(String url, String ctype, byte[] content, int connectTimeout,
int readTimeout, Proxy proxy) throws IOException {
return _doPost(url, ctype, content, connectTimeout, readTimeout, null, proxy);
}
@Deprecated
public static String doPost(String url, String ctype, byte[] content, int connectTimeout,
int readTimeout) throws IOException {
return doPost(url, ctype, content, connectTimeout, readTimeout, null);
}
private static String _doPost(String url, String ctype, byte[] content, int connectTimeout,
int readTimeout, Map headerMap, Proxy proxy)
throws IOException {
HttpURLConnection conn = null;
OutputStream out = null;
String rsp = null;
try {
try {
conn = getConnection(new URL(url), METHOD_POST, ctype, headerMap, proxy);
conn.setConnectTimeout(connectTimeout);
conn.setReadTimeout(readTimeout);
} catch (IOException e) {
throw e;
}
try {
out = conn.getOutputStream();
out.write(content);
rsp = getResponseAsString(conn);
} catch (IOException e) {
throw e;
}
} finally {
if (out != null) {
out.close();
}
if (conn != null) {
conn.disconnect();
}
}
return rsp;
}
public static String doPost(String url, Map params,
Map fileParams, int connectTimeout,
int readTimeout, Proxy proxy) throws IOException {
if (fileParams == null || fileParams.isEmpty()) {
return doPost(url, params, DEFAULT_CHARSET, connectTimeout, readTimeout, proxy);
} else {
return doPost(url, params, fileParams, DEFAULT_CHARSET, connectTimeout, readTimeout);
}
}
public static String doPost(String url, Map params,
Map fileParams, int connectTimeout,
int readTimeout) throws IOException {
return doPost(url, params, fileParams, connectTimeout, readTimeout, null);
}
public static String doPost(String url, Map params,
Map fileParams, String charset,
int connectTimeout, int readTimeout, Proxy proxy) throws IOException {
return doPost(url, params, fileParams, charset, connectTimeout, readTimeout, null, proxy);
}
public static String doPost(String url, Map params,
Map fileParams, String charset,
int connectTimeout, int readTimeout) throws IOException {
return doPost(url, params, fileParams, charset, connectTimeout, readTimeout, null, null);
}
public static String doPost(String url, Map params,
Map fileParams, String charset,
int connectTimeout, int readTimeout, Map headerMap, Proxy proxy)
throws IOException {
if (fileParams == null || fileParams.isEmpty()) {
return doPost(url, params, charset, connectTimeout, readTimeout, headerMap, proxy);
} else {
return _doPostWithFile(url, params, fileParams, charset, connectTimeout, readTimeout,
headerMap);
}
}
public static String doPost(String url, Map params,
Map fileParams, String charset,
int connectTimeout, int readTimeout, Map headerMap)
throws IOException {
return doPost(url, params, fileParams, charset, connectTimeout, readTimeout, headerMap, null);
}
private static String _doPostWithFile(String url, Map params,
Map fileParams, String charset,
int connectTimeout, int readTimeout,
Map headerMap) throws IOException {
String boundary = System.currentTimeMillis() + ""; // 随机分隔线
HttpURLConnection conn = null;
OutputStream out = null;
String rsp = null;
try {
try {
String ctype = "multipart/form-data;charset=" + charset + ";boundary=" + boundary;
conn = getConnection(new URL(url), METHOD_POST, ctype, headerMap);
conn.setConnectTimeout(connectTimeout);
conn.setReadTimeout(readTimeout);
} catch (IOException e) {
throw e;
}
try {
out = conn.getOutputStream();
byte[] entryBoundaryBytes = ("\r\n--" + boundary + "\r\n").getBytes(charset);
// 组装文本请求参数
Set> textEntrySet = params.entrySet();
for (Entry textEntry : textEntrySet) {
byte[] textBytes = getTextEntry(textEntry.getKey(), textEntry.getValue(),
charset);
out.write(entryBoundaryBytes);
out.write(textBytes);
}
// 组装文件请求参数
Set> fileEntrySet = fileParams.entrySet();
for (Entry fileEntry : fileEntrySet) {
FileItem fileItem = fileEntry.getValue();
if (fileItem.getContent() == null) {
continue;
}
byte[] fileBytes = getFileEntry(fileEntry.getKey(), fileItem.getFileName(),
fileItem.getMimeType(), charset);
out.write(entryBoundaryBytes);
out.write(fileBytes);
out.write(fileItem.getContent());
}
// 添加请求结束标志
byte[] endBoundaryBytes = ("\r\n--" + boundary + "--\r\n").getBytes(charset);
out.write(endBoundaryBytes);
rsp = getResponseAsString(conn);
} catch (IOException e) {
throw e;
}
} finally {
if (out != null) {
out.close();
}
if (conn != null) {
conn.disconnect();
}
}
return rsp;
}
private static byte[] getTextEntry(String fieldName, String fieldValue, String charset)
throws IOException {
String entry = "Content-Disposition:form-data;name=\"" +
fieldName +
"\"\r\nContent-Type:text/plain\r\n\r\n" +
fieldValue;
return entry.getBytes(charset);
}
private static byte[] getFileEntry(String fieldName, String fileName, String mimeType,
String charset) throws IOException {
String entry = "Content-Disposition:form-data;name=\"" +
fieldName +
"\";filename=\"" +
fileName +
"\"\r\nContent-Type:" +
mimeType +
"\r\n\r\n";
return entry.getBytes(charset);
}
public static String doGet(String url, Map params) throws IOException {
return doGet(url, params, DEFAULT_CHARSET, null);
}
public static String doGet(String url, Map params, Proxy proxy) throws IOException {
return doGet(url, params, DEFAULT_CHARSET, proxy);
}
public static String doGet(String url, Map params, String charset, Proxy proxy)
throws IOException {
HttpURLConnection conn = null;
String rsp = null;
try {
String ctype = "application/x-www-form-urlencoded;charset=" + charset;
String query = buildQuery(params, charset, false);
try {
conn = getConnection(buildGetUrl(url, query), METHOD_GET, ctype, null, proxy);
} catch (IOException e) {
throw e;
}
try {
rsp = getResponseAsString(conn);
} catch (IOException e) {
throw e;
}
} finally {
if (conn != null) {
conn.disconnect();
}
}
return rsp;
}
public static String doGet(String url, Map params, String charset)
throws IOException {
return doGet(url, params, charset, null);
}
private static HttpURLConnection getConnection(URL url, String method, String ctype,
Map headerMap) throws IOException {
return getConnection(url, method, ctype, headerMap, null);
}
private static HttpURLConnection getConnection(URL url, String method, String ctype,
Map headerMap, Proxy proxy)
throws IOException {
HttpURLConnection conn = null;
if (proxy == null) {
proxy = Proxy.NO_PROXY;
}
if ("https".equals(url.getProtocol())) {
SSLContext ctx = null;
try {
ctx = SSLContext.getInstance("TLS");
ctx.init(new KeyManager[0], new TrustManager[]{new DefaultTrustManager()},
new SecureRandom());
} catch (Exception e) {
throw new IOException(e);
}
HttpsURLConnection connHttps = (HttpsURLConnection) url.openConnection(proxy);
connHttps.setSSLSocketFactory(ctx.getSocketFactory());
connHttps.setHostnameVerifier(new HostnameVerifier() {
public boolean verify(String hostname, SSLSession session) {
return true;// 默认都认证通过
}
});
conn = connHttps;
} else {
conn = (HttpURLConnection) url.openConnection(proxy);
}
conn.setRequestMethod(method);
conn.setDoInput(true);
conn.setDoOutput(true);
conn.setRequestProperty("Accept", "text/xml,text/javascript,text/html");
conn.setRequestProperty("User-Agent", "acapi-sdk-java");
conn.setRequestProperty("Content-Type", ctype);
if (headerMap != null) {
for (Map.Entry entry : headerMap.entrySet()) {
conn.setRequestProperty(entry.getKey(), entry.getValue());
}
}
return conn;
}
private static URL buildGetUrl(String strUrl, String query) throws IOException {
URL url = new URL(strUrl);
if (StringUtils.isEmpty(query)) {
return url;
}
if (StringUtils.isEmpty(url.getQuery())) {
if (strUrl.endsWith("?")) {
strUrl = strUrl + query;
} else {
strUrl = strUrl + "?" + query;
}
} else {
if (strUrl.endsWith("&")) {
strUrl = strUrl + query;
} else {
strUrl = strUrl + "&" + query;
}
}
return new URL(strUrl);
}
public static String buildQuery(Map params, String charset, boolean isRest) throws IOException {
if (params == null || params.isEmpty()) {
return null;
}
StringBuilder query = new StringBuilder();
Set> entries = params.entrySet();
for (Entry entry : entries) {
String name = entry.getKey();
String value = entry.getValue();
if (name == null) {
throw new IllegalArgumentException("Key cannot be null");
}
if (query.length() > 0) {
query.append("&");
}
if (isRest) {
name = URLEncoder.encode(name, charset);
}
query.append(name);
if (value != null) {
query.append("=");
query.append(URLEncoder.encode(value, charset));
}
}
return query.toString();
}
protected static String getResponseAsString(HttpURLConnection conn) throws IOException {
String charset = getResponseCharset(conn.getContentType());
InputStream es = conn.getErrorStream();
if (es == null) {
return getStreamAsString(conn.getInputStream(), charset);
} else {
String msg = getStreamAsString(es, charset);
if (StringUtils.isEmpty(msg)) {
throw new IOException(conn.getResponseCode() + ":" + conn.getResponseMessage());
} else {
return msg;
}
}
}
private static String getStreamAsString(InputStream stream, String charset) throws IOException {
try {
Reader reader = new InputStreamReader(stream, charset);
StringBuilder response = new StringBuilder();
final char[] buff = new char[1024];
int read = 0;
while ((read = reader.read(buff)) > 0) {
response.append(buff, 0, read);
}
return response.toString();
} finally {
if (stream != null) {
stream.close();
}
}
}
private static String getResponseCharset(String ctype) {
String charset = DEFAULT_CHARSET;
if (!StringUtils.isEmpty(ctype)) {
String[] params = ctype.split(";");
for (String param : params) {
param = param.trim();
if (param.startsWith("charset")) {
String[] pair = param.split("=", 2);
if (pair.length == 2) {
if (!StringUtils.isEmpty(pair[1])) {
charset = pair[1].trim();
}
}
break;
}
}
}
return charset;
}
/**
* 使用默认的UTF-8字符集反编码请求参数值。
*
* @param value 参数值
* @return 反编码后的参数值
*/
public static String decode(String value) {
return decode(value, DEFAULT_CHARSET);
}
/**
* 使用默认的UTF-8字符集编码请求参数值。
*
* @param value 参数值
* @return 编码后的参数值
*/
public static String encode(String value) {
return encode(value, DEFAULT_CHARSET);
}
/**
* 使用指定的字符集反编码请求参数值。
*
* @param value 参数值
* @param charset 字符集
* @return 反编码后的参数值
*/
public static String decode(String value, String charset) {
String result = null;
if (!StringUtils.isEmpty(value)) {
try {
result = URLDecoder.decode(value, charset);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
return result;
}
/**
* 使用指定的字符集编码请求参数值。
*
* @param value 参数值
* @param charset 字符集
* @return 编码后的参数值
*/
public static String encode(String value, String charset) {
String result = null;
if (!StringUtils.isEmpty(value)) {
try {
result = URLEncoder.encode(value, charset);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
return result;
}
/**
* 从URL中提取所有的参数。
*
* @param query URL地址
* @return 参数映射
*/
public static Map splitUrlQuery(String query) {
Map result = new HashMap();
String[] pairs = query.split("&");
if (pairs != null && pairs.length > 0) {
for (String pair : pairs) {
String[] param = pair.split("=", 2);
if (param != null && param.length == 2) {
result.put(param[0], param[1]);
}
}
}
return result;
}
private static class DefaultTrustManager implements X509TrustManager {
@Override
public X509Certificate[] getAcceptedIssuers() {
return null;
}
@Override
public void checkClientTrusted(X509Certificate[] chain, String authType)
throws CertificateException {
}
@Override
public void checkServerTrusted(X509Certificate[] chain, String authType)
throws CertificateException {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy