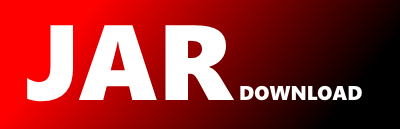
cn.com.antcloud.api.acapi.StringUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antcloud-api-sdk Show documentation
Show all versions of antcloud-api-sdk Show documentation
Ant Fin Tech API SDK For Java
Copyright (c) 2015-present Alipay.com, https://www.alipay.com
The newest version!
/*
* Copyright (c) 2015-present Alipay.com, https://www.alipay.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package cn.com.antcloud.api.acapi;
/**
* 字符串处理
*/
public abstract class StringUtils {
private StringUtils() {
}
/**
* 判空
* @param value
* @return
*/
public static boolean isEmpty(String value) {
int strLen;
if (value == null || (strLen = value.length()) == 0) {
return true;
}
for (int i = 0; i < strLen; i++) {
if ((!Character.isWhitespace(value.charAt(i)))) {
return false;
}
}
return true;
}
/**
* 判断是否是数字
* @param obj
* @return
*/
public static boolean isNumeric(Object obj) {
if (obj == null) {
return false;
}
char[] chars = obj.toString().toCharArray();
int length = chars.length;
if (length < 1)
return false;
int i = 0;
if (length > 1 && chars[0] == '-')
i = 1;
for (; i < length; i++) {
if (!Character.isDigit(chars[i])) {
return false;
}
}
return true;
}
/**
* 判读对象是否都是数字
* @param obj
* @return
*/
public static boolean isNumericAll(Object obj) {
if (obj == null) {
return false;
}
return obj.toString().matches("^[-+]?(([0-9]+)([.]([0-9]+))?|([.]([0-9]+))?)$");
}
/**
* 判断不空
* @param values
* @return
*/
public static boolean areNotEmpty(String... values) {
boolean result = true;
if (values == null || values.length == 0) {
result = false;
} else {
for (String value : values) {
result &= !isEmpty(value);
}
}
return result;
}
/**
* 改为中文
* @param unicode
* @return
*/
public static String unicodeToChinese(String unicode) {
StringBuilder out = new StringBuilder();
if (!isEmpty(unicode)) {
for (int i = 0; i < unicode.length(); i++) {
out.append(unicode.charAt(i));
}
}
return out.toString();
}
/**
* 转化为下划线格式
* @param name
* @return
*/
public static String toUnderlineStyle(String name) {
StringBuilder newName = new StringBuilder();
for (int i = 0; i < name.length(); i++) {
char c = name.charAt(i);
if (Character.isUpperCase(c)) {
if (i > 0) {
newName.append("_");
}
newName.append(Character.toLowerCase(c));
} else {
newName.append(c);
}
}
return newName.toString();
}
/**
* 转化字符串
* @param data
* @param offset
* @param length
* @return
*/
public static String convertString(byte[] data, int offset, int length) {
if (data == null) {
return null;
} else {
try {
return new String(data, offset, length, Constants.CHARSET_UTF8);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
/**
* 转化为字节
* @param data
* @return
*/
public static byte[] convertBytes(String data) {
if (data == null) {
return null;
} else {
try {
return data.getBytes(Constants.CHARSET_UTF8);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
/**
* 判断是否相等
* @param str1
* @param str2
* @return
*/
public static boolean equals(String str1, String str2) {
if (str1 == null) {
return str2 == null;
}
return str1.equals(str2);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy