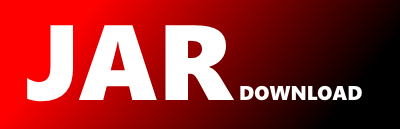
cn.com.antcloud.api.antcloud.rest.AntCloudRestClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antcloud-api-sdk Show documentation
Show all versions of antcloud-api-sdk Show documentation
Ant Fin Tech API SDK For Java
Copyright (c) 2015-present Alipay.com, https://www.alipay.com
The newest version!
/*
* Copyright (c) 2015-present Alipay.com, https://www.alipay.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package cn.com.antcloud.api.antcloud.rest;
import cn.com.antcloud.api.acapi.AntCloudHttpClient;
import cn.com.antcloud.api.acapi.AntCloudWebUtils;
import cn.com.antcloud.api.acapi.StringUtils;
import cn.com.antcloud.api.common.*;
import cn.com.antcloud.api.rest.RestConstants;
import cn.com.antcloud.api.rest.RestHeaders;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.util.Date;
/**
* rest调用client
*/
public class AntCloudRestClient extends
BaseGwClient {
private static final Logger logger = LoggerFactory
.getLogger(AntCloudRestClient.class);
private static final String URL_DELIMITER = "/";
private static final String JAVA_SDK_VERSION = "Java-SDK-20180705";
private AntCloudRestClient(String endpoint, String accessKey, String accessSecret,
boolean checkSign, boolean enableAutoRetry, int autoRetryLimit,
AntCloudHttpClient client, String securityToken, String clientId) {
super(endpoint, accessKey, accessSecret, checkSign, enableAutoRetry, autoRetryLimit, client, securityToken, clientId);
if (endpoint != null && !endpoint.contains(RestConstants.REST_API_PREFIX)) {
throw new IllegalArgumentException(
"Endpoint should contains " + RestConstants.REST_API_PREFIX);
}
}
/**
* 构造器
* @return
*/
public static Builder newBuilder() {
return new Builder();
}
/**
* 执行
* @param request
* @return
*/
@Override
public AntCloudRestClientResponse execute(AntCloudRestClientRequest request) {
SDKUtils.checkNotNull(request.getUrlPath(), "Url path cannot be null");
SDKUtils.checkNotNull(request.getHttpMethod(), "Http method cannot be null");
SDKUtils.checkNotNull(request.getApiVersion(), "Api version cannot be null");
// 确保product instance id 和 region name 两个中必须有一个
if (request.getRegionName() == null && request.getProductInstanceId() == null) {
throw new IllegalArgumentException("Please set region or product instance id");
}
// prepare parameters
request.putHeader(RestHeaders.ACCESS_KEY, this.getAccessKey());
request.putHeader(RestHeaders.REQ_MSG_ID, SDKUtils.generateReqMsgId());
request.putHeader(RestHeaders.REQ_TIME, SDKUtils.formatDate(new Date()));
request.putHeader(RestHeaders.SDK_VERSION, JAVA_SDK_VERSION);
// set sign type
String signType = request.getHeaderValue(RestHeaders.SIGN_TYPE);
if (StringUtils.isEmpty(signType)) {
signType = GwSignType.HmacSHA1.getCode();
request.putHeader(RestHeaders.SIGN_TYPE, signType);
}
// 计算签名
try {
String stringToSign = GwRestSignUtils.getStringToSign(request.getUrlPath(),
request.getHttpMethod().getCode(), request.getSignHeaders(),
request.getQueryParams(), request.getPostBody());
if (logger.isDebugEnabled()) {
logger.debug("String to sign: \n{}", stringToSign);
}
String sign = GwSigns.sign(stringToSign, signType, this.getAccessSecret(),
SDKConstants.SIGN_CHARSET);
request.putHeader(RestHeaders.SIGN, sign);
} catch (Exception e) {
throw new ClientException(SDKConstants.ResultCodes.UNKNOWN_ERROR, e);
}
// send request
try {
String apiUrl = getCorrectUrl(this.getEndpoint(), request.getUrlPath());
String responseString = AntCloudWebUtils.doRestRequest(apiUrl, request.getHttpMethod(),
request.getHeaders(), request.getQueryParams(), request.getPostBody(),
this.httpClient.getHttpConfig().getConnectionTimeoutMillis(),
this.httpClient.getHttpConfig().getReadTimeoutMillis(), null);
// HttpUriRequest httpUriRequest = this.buildRequest(apiUrl, request.getParameters());
// HttpResponse httpResponse = this.httpClient.invoke(httpUriRequest);
// String responseString = EntityUtils.toString(httpResponse.getEntity(), Charset.forName("UTF-8"));
JSONObject wholeJson = JSON.parseObject(responseString);
if (wholeJson == null) {
logger.error(responseString);
throw new ClientException(SDKConstants.ResultCodes.TRANSPORT_ERROR,
"Unexpected gateway response: " + responseString);
}
JSONObject responseNode = wholeJson.getJSONObject("response");
if (responseNode == null) {
logger.error(responseString);
throw new ClientException(SDKConstants.ResultCodes.TRANSPORT_ERROR,
"Unexpected gateway response: " + responseString);
}
AntCloudRestClientResponse response = newResponse();
response.setData(responseNode);
if (response.isSuccess() && this.isCheckSign()) {
String sign = wholeJson.getString(SDKConstants.ParamKeys.SIGN);
String stringToSign = GwSigns.extractStringToSign(responseString);
String calculatedSign;
try {
calculatedSign = GwSigns.sign(stringToSign, signType, this.getAccessSecret(),
SDKConstants.SIGN_CHARSET);
} catch (Exception e) {
throw new ClientException(SDKConstants.ResultCodes.BAD_SIGNATURE,
"Invalid signature in response");
}
if (!calculatedSign.equals(sign)) {
throw new ClientException(SDKConstants.ResultCodes.BAD_SIGNATURE,
"Invalid signature in response");
}
}
return response;
} catch (IOException e) {
throw new ClientException(SDKConstants.ResultCodes.TRANSPORT_ERROR, e);
}
}
/**
* 将多截的URL拼接在一起,并且中间不能有多余的/
* 比如拼接"http://www.alipay.com/"和"/demo/test/",最后正确的拼接结果应该为"http://www.alipay.com/demo/test",没有多余的/
* 需要注意的是,代码中并没有完整确保不会有多余的/,比如"////demo//test//"这种URL。这是为了性能的考虑,因为大概率用户不会传入这样的URL。
*
* @param urlFragments
* @return
*/
private String getCorrectUrl(String... urlFragments) {
for (int i = 0; i < urlFragments.length; i++) {
String urlFragment = urlFragments[i];
if (urlFragment == null) {
throw new IllegalArgumentException("Url fragment can't be null");
}
// 除第一截外,都需要在开头加上缺少的/
if (i != 0) {
if (!urlFragment.startsWith(URL_DELIMITER)) {
// 如果开头没有/,需要加上
urlFragment = URL_DELIMITER + urlFragment;
}
}
if (urlFragment.endsWith(URL_DELIMITER)) {
// 如果末尾有多余的/,则需要去掉
urlFragment = urlFragment.substring(0, urlFragment.length() - 1);
}
urlFragments[i] = urlFragment;
}
StringBuilder sb = new StringBuilder();
for (String urlFragment : urlFragments) {
sb.append(urlFragment);
}
return sb.toString();
}
/**
* 构造器
*/
public static class Builder extends BaseGwClient.Builder {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy