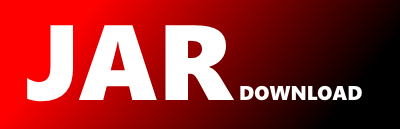
cn.com.antcloud.api.common.BaseClientRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antcloud-api-sdk Show documentation
Show all versions of antcloud-api-sdk Show documentation
Ant Fin Tech API SDK For Java
Copyright (c) 2015-present Alipay.com, https://www.alipay.com
The newest version!
/*
* Copyright (c) 2015-present Alipay.com, https://www.alipay.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package cn.com.antcloud.api.common;
import java.lang.reflect.Type;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
public class BaseClientRequest {
private final Map parameters;
private final Map headersParameters;
/**
* Constructor.
*/
public BaseClientRequest() {
this.parameters = new HashMap();
this.headersParameters = new HashMap();
}
/**
* Put parameter.
*
* @param key the key
* @param value the value
*/
public void putParameter(String key, String value) {
SDKUtils.checkNotNull(key);
parameters.put(key, value);
}
/**
* Remove parameter.
*
* @param key the key
*/
public void removeParameter(String key) {
SDKUtils.checkNotNull(key);
parameters.remove(key);
}
/**
* Gets parameter.
*
* @param key the key
* @return the parameter
*/
public String getParameter(String key) {
return parameters.get(key);
}
/**
* Put parameters.
*
* @param params the params
*/
public void putParameters(Map params) {
SDKUtils.checkNotNull(params);
if (params.containsKey(null)) {
throw new IllegalArgumentException("Null key is not allowed");
}
parameters.putAll(params);
}
/**
* Put parameters from object.
*
* @param o the o
*/
public void putParametersFromObject(Object o) {
Map params = GwKeyValues.toMap(o);
putParameters(params);
}
public Map getHeadersParameters() {
return headersParameters;
}
/**
* Gets parameters as object.
*
* @param the type parameter
* @param clazz the clazz
* @return the parameters as object
*/
public T getParametersAsObject(Class clazz) {
return GwKeyValues.toObject(parameters, clazz);
}
/**
* Gets parameters as object.
*
* @param the type parameter
* @param type the type
* @return the parameters as object
*/
public T getParametersAsObject(Type type) {
return GwKeyValues.toObject(parameters, type);
}
/**
* Gets parameters.
*
* @return the parameters
*/
public Map getParameters() {
return Collections.unmodifiableMap(parameters);
}
/**
* Gets method.
*
* @return the method
*/
public String getMethod() {
return getParameter(SDKConstants.ParamKeys.METHOD);
}
/**
* Sets method.
*
* @param method the method
*/
public void setMethod(String method) {
putParameter(SDKConstants.ParamKeys.METHOD, method);
}
/**
* Gets version.
*
* @return the version
*/
public String getVersion() {
return getParameter(SDKConstants.ParamKeys.VERSION);
}
/**
* Sets version.
*
* @param version the version
*/
public void setVersion(String version) {
putParameter(SDKConstants.ParamKeys.VERSION, version);
}
/**
* Gets req msg id.
*
* @return the req msg id
*/
public String getReqMsgId() {
return getParameter(SDKConstants.ParamKeys.REQ_MSG_ID);
}
/**
* Sets req msg id.
*
* @param reqMsgId the req msg id
*/
public void setReqMsgId(String reqMsgId) {
putParameter(SDKConstants.ParamKeys.REQ_MSG_ID, reqMsgId);
}
/**
* Gets is encrpt
*
* @return the is encrpt
*/
public String getEncrypt() {
return getParameter(SDKConstants.ParamKeys.ENCRYPT);
}
/**
* Sets req msg id.
*
* @param encrypt the is encrpt
*/
public void setEncrpt(String encrypt) {
putParameter(SDKConstants.ParamKeys.ENCRYPT, encrypt);
}
/**
* Gets sign type.
*
* @return the sign type
*/
public String getSignType() {
return getParameter(SDKConstants.ParamKeys.SIGN_TYPE);
}
/**
* Sets sign type.
*
* @param signType the sign type
*/
public void setSignType(String signType) {
putParameter(SDKConstants.ParamKeys.SIGN_TYPE, signType);
}
/**
* Gets sign key version.
*
* @return the sign key version
*/
public String getSignKeyVersion() {
return getParameter(SDKConstants.ParamKeys.SIGN_KEY_VERSION);
}
/**
* Sets sign key version.
*
* @param signKeyVersion the sign key version
*/
public void setSignKeyVersion(String signKeyVersion) {
putParameter(SDKConstants.ParamKeys.SIGN_KEY_VERSION, signKeyVersion);
}
/**
* Gets encryption version.
*
* @return the encryption version
*/
public String getEncryptionVersion() {
return getParameter(SDKConstants.ParamKeys.ENCRYPTION_VERSION);
}
/**
* Sets encryption version.
*
* @param encryptionVersion the encryption version
*/
public void setEncryptionVersion(String encryptionVersion) {
putParameter(SDKConstants.ParamKeys.ENCRYPTION_VERSION, encryptionVersion);
}
/**
* Equals boolean.
*
* @param o the o
* @return the boolean
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
BaseClientRequest that = (BaseClientRequest) o;
return parameters != null ? parameters.equals(that.parameters) : that.parameters == null;
}
/**
* Hash code int.
*
* @return the int
*/
@Override
public int hashCode() {
return parameters != null ? parameters.hashCode() : 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy