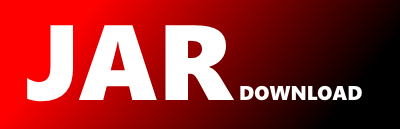
cn.com.antcloud.api.common.GwEncrypt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antcloud-api-sdk Show documentation
Show all versions of antcloud-api-sdk Show documentation
Ant Fin Tech API SDK For Java
Copyright (c) 2015-present Alipay.com, https://www.alipay.com
The newest version!
/*
* Copyright (c) 2015-present Alipay.com, https://www.alipay.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package cn.com.antcloud.api.common;
import org.apache.commons.codec.binary.Base64;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
import java.security.SecureRandom;
/**
* 加密
*/
public class GwEncrypt {
private static final Logger logger = LoggerFactory.getLogger(GwEncrypt.class);
private static SecretKey getSecretKey(String password) throws Exception {
KeyGenerator keygen = KeyGenerator.getInstance("AES");
SecureRandom random = SecureRandom.getInstance("SHA1PRNG");
random.setSeed(password.getBytes());
keygen.init(128, random);
SecretKey original_key = keygen.generateKey();
byte[] raw = original_key.getEncoded();
return new SecretKeySpec(raw, "AES");
}
/**
* aes加密
* @param content
* @param secret
* @return
* @throws Exception
*/
public static String AESEncript(String content, String secret) throws Exception {
SecretKey key = getSecretKey(secret);
Cipher cipher = Cipher.getInstance("AES");
// 初始化密码器,第一个参数为加密(ENCRYPT_MODE)或者解密(DECRYPT_MODE)操作,第二个参数为使用的KEY
cipher.init(Cipher.ENCRYPT_MODE, key);
// 获取加密内容的字节数组(这里要设置为utf-8)不然内容中如果有中文和英文混合中文就会解密为乱码
byte[] encode = content.getBytes("utf-8");
// 根据密码器的初始化方式--加密:将数据加密
byte[] aesByte = cipher.doFinal(encode);
// 将加密后的数据转换为字符串
return parseByte2HexStr(Base64.encodeBase64(aesByte));
}
/**
* aes加密
* @param content
* @param secret
* @return
* @throws Exception
*/
public static String AESDecript(String content, String secret) throws Exception {
SecretKey key = getSecretKey(secret);
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.DECRYPT_MODE, key);
// 将加密并编码后的内容解码成字节数组
byte[] contentByte = Base64.decodeBase64(parseHexStr2Byte(content));
// 解密
byte[] decodeByte = cipher.doFinal(contentByte);
return new String(decodeByte, "utf-8");
}
private static String parseByte2HexStr(byte buf[]) {
StringBuilder sb = new StringBuilder();
for (byte aBuf : buf) {
String hex = Integer.toHexString(aBuf & 0xFF);
if (hex.length() == 1) {
hex = '0' + hex;
}
sb.append(hex.toUpperCase());
}
return sb.toString();
}
private static byte[] parseHexStr2Byte(String hexStr) {
byte[] result = new byte[hexStr.length() / 2];
for (int i = 0; i < hexStr.length() / 2; i++) {
int high = Integer.parseInt(hexStr.substring(i * 2, i * 2 + 1), 16);
int low = Integer.parseInt(hexStr.substring(i * 2 + 1, i * 2 + 2), 16);
result[i] = (byte) (high * 16 + low);
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy