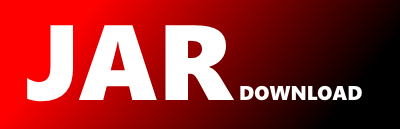
cn.crazywalker.fsf.oss.OSSServiceImpl Maven / Gradle / Ivy
The newest version!
package cn.crazywalker.fsf.oss;
import cn.crazywalker.fsf.oss.exception.OSSException;
import org.slf4j.Logger;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.text.MessageFormat;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* 对象存储逻辑接口实现类
* @author CrazyWalker
* @since 周日, 12/18 2022 17:41 GMT+8
*/
public class OSSServiceImpl implements OSSService {
private static final Logger LOGGER = org.slf4j.LoggerFactory.getLogger(OSSServiceImpl.class);
private static final String MODULE_NAME_EXCEPTION_MESSAGE_TEMPLATE =
"OOSServiceImpl constructor: module name {0} is duplication.";
private static final String MODULE_HANDLE_NOT_FOUND = "upload: handler for module name {0} is not found.";
private static final String OOS_KEY_PATTERN = "{0}/{1}";
private final OSSClient oosClient;
private final Map moduleFileHandlerMap;
public OSSServiceImpl(OSSClient aliyunOOSClient, List ossFileHandlerList) {
this.oosClient = aliyunOOSClient;
if (ossFileHandlerList == null) {
moduleFileHandlerMap = new HashMap<>();
return ;
}
moduleFileHandlerMap = new HashMap<>(ossFileHandlerList.size());
ossFileHandlerList.forEach(handler -> {
String moduleName = handler.getModuleName();
if (moduleFileHandlerMap.containsKey(moduleName)) {
throw new OSSException(MessageFormat.format(MODULE_NAME_EXCEPTION_MESSAGE_TEMPLATE, moduleName));
}
moduleFileHandlerMap.put(moduleName, handler);
});
}
@Override
public String upload(String moduleName, Object uploadInfo, MultipartFile file) {
OSSFileHandler handler = moduleFileHandlerMap.get(moduleName);
if (handler == null) {
throw new OSSException(MessageFormat.format(MODULE_HANDLE_NOT_FOUND, moduleName));
}
String filePath = handler.filePath(uploadInfo, file);
try {
byte[] fileBytes = file.getBytes();
return this.innerUpload(moduleName, filePath, fileBytes);
} catch (IOException e) {
LOGGER.error("阿里云对象存储上传失败: ", e);
throw new OSSException("MultipartFile获取字节流失败.");
}
}
@Override
public String upload(String moduleName, Object uploadInfo, byte[] fileBytes) {
OSSFileHandler handler = moduleFileHandlerMap.get(moduleName);
if (handler == null) {
throw new OSSException(MessageFormat.format(MODULE_HANDLE_NOT_FOUND, moduleName));
}
String filePath = handler.filePath(uploadInfo, fileBytes);
return this.innerUpload(moduleName, filePath, fileBytes);
}
private String innerUpload(String moduleName, String filePath, byte[] fileBytes) {
String oosKey = MessageFormat.format(OOS_KEY_PATTERN, moduleName, filePath);
oosClient.simpleUpload(oosKey, fileBytes);
return oosKey;
}
@Override
public String getPath(String oosKey) {
return oosClient.getPathByOosKey(oosKey);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy