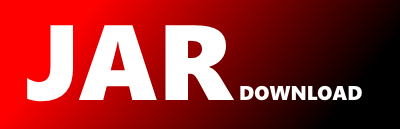
com.dtp.core.parser.JsonConfigParser Maven / Gradle / Ivy
package com.dtp.core.parser;
import com.dtp.common.em.ConfigFileTypeEnum;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.collections.MapUtils;
import org.apache.commons.lang3.StringUtils;
import java.io.IOException;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import static com.dtp.common.constant.DynamicTpConst.*;
/**
* JsonConfigParser related
*
* @author yanhom
* @since 1.0.5
**/
@Slf4j
@SuppressWarnings("unchecked")
public class JsonConfigParser extends AbstractConfigParser {
private static final List CONFIG_TYPES = Lists.newArrayList(ConfigFileTypeEnum.JSON);
private static final ObjectMapper MAPPER;
static {
MAPPER = new ObjectMapper();
}
@Override
public List types() {
return CONFIG_TYPES;
}
@Override
public Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy