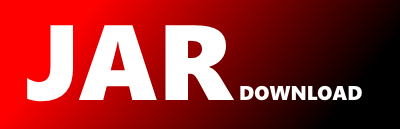
cn.easyproject.easycommons.commondao.CommonDAOImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of easycommondao-hibernate4 Show documentation
Show all versions of easycommondao-hibernate4 Show documentation
Java ORM Common DAO(Data Access Object), eliminate the interface and implements of DAO.
package cn.easyproject.easycommons.commondao;
import java.io.Serializable;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import org.hibernate.Hibernate;
import org.hibernate.Query;
import org.hibernate.Session;
import org.hibernate.Transaction;
import org.hibernate.jdbc.Work;
import org.hibernate.transform.Transformers;
import cn.easyproject.easycommons.commondao.util.EasyCriteria;
import cn.easyproject.easycommons.commondao.util.PageBean;
import cn.easyproject.easycommons.commondao.factory.HibernateSessionFactory;
/**
* Hibernat4 CommonDAO implement.
* @author Ray
* @author [email protected]
* @author easyproject.cn
* @since 1.0.0
*
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
public class CommonDAOImpl implements CommonDAO {
@Override
public void batchUpdateSQL(final String sql, final Object[] values) {
Session session=HibernateSessionFactory.getSession();
session.doWork(new Work() {
@Override
public void execute(Connection connection) throws SQLException {
PreparedStatement pstmt = null;
try {
connection.setAutoCommit(false);// 手动提交
pstmt = connection.prepareStatement(sql);// 预编译
for (int i = 0; i < values.length; i++) {
pstmt.setObject(1, values[i]);
pstmt.addBatch();
}
pstmt.executeBatch();// 将参数加入到批处理
connection.commit();// 提交
} catch (Exception e) {
e.printStackTrace();
} finally {
if (pstmt != null) {
try {
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
try {
if (connection != null && (!connection.isClosed())) {
connection.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
});
if (session.isOpen()) {
HibernateSessionFactory.closeSession();
}
}
@Override
public void batchUpdateSQL(final String sql,final Object[][] values) {
Session session=HibernateSessionFactory.getSession();
session.doWork(new Work() {
@Override
public void execute(Connection connection) throws SQLException {
PreparedStatement pstmt = null;
try {
connection.setAutoCommit(false);// 手动提交
pstmt = connection.prepareStatement(sql);// 预编译
for (Object[] row : values) {
for (int i = 0; i < row.length; i++) {
pstmt.setObject(i + 1, row[i]);
}
pstmt.addBatch();
}
pstmt.executeBatch();// 将参数加入到批处理
connection.commit();// 提交
} catch (Exception e) {
e.printStackTrace();
} finally {
if (pstmt != null) {
try {
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
try {
if (connection != null && (!connection.isClosed())) {
connection.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
});
if (session.isOpen()) {
HibernateSessionFactory.closeSession();
}
}
/**
* CommonDAO 内部方法,获得Session,并开始事务
* @return Session
*/
private Session beginTransaction(){
Session session=HibernateSessionFactory.getSession();
session.getTransaction().begin();
return session;
}
/**
* CommonDAO 内部方法,关闭Session
*/
private void close(){
HibernateSessionFactory.closeSession();
}
/**
* CommonDAO 内部方法,提交事务
*/
private void commit(Session session){
session.getTransaction().commit();
}
/**
* CommonDAO 内部方法,NamedQuery
* @param session session
* @param name name
* @param cacheable cacheable
* @param cacheRegion cacheRegion
* @param values values
* @return Query对象
*/
private Query createNamedQuery(Session session,String name, boolean cacheable,String cacheRegion, Object...values){
Query query=session.getNamedQuery(name.trim());
if(cacheable){
query.setCacheable(true);
if(cacheRegion!=null&&(!cacheRegion.equals(""))){
query.setCacheRegion(cacheRegion);
}
}
if(values!=null&&values.length>0){
for (int i = 0; i < values.length; i++) {
query.setParameter(i, values[i]);
}
}
return query;
}
/**
* CommonDAO 内部方法, NativeQuery
* @param session session
* @param sql sql
* @param values values
* @return Query对象
*/
private Query createNativeQuery(Session session,String sql,Class resultClass, boolean mapResult, Object...values){
Query query=null;
if(resultClass!=null){
query=session.createSQLQuery(sql).addEntity(resultClass);
}else{
query=session.createSQLQuery(sql);
}
// 封装为Map结果
if(mapResult){
query.setResultTransformer(Transformers.ALIAS_TO_ENTITY_MAP);
}
if(values!=null&&values.length>0){
for (int i = 0; i < values.length; i++) {
query.setParameter(i, values[i]);
}
}
return query;
}
/**
* CommonDAO 内部方法,Query
* @param session session
* @param hql jqpl
* @param cacheable cacheable
* @param cacheRegion cacheRegion
* @param values values
* @return Query对象
*/
private Query createQuery(Session session,String hql, boolean cacheable,String cacheRegion, Object...values){
Query query=session.createQuery(hql);
if(cacheable){
query.setCacheable(true);
if(cacheRegion!=null&&(!cacheRegion.equals(""))){
query.setCacheRegion(cacheRegion);
}
}
if(values!=null&&values.length>0){
for (int i = 0; i < values.length; i++) {
query.setParameter(i, values[i]);
}
}
return query;
}
@Override
public void delete(Class clazz, Serializable id) {
Session session = null;
try {
session = beginTransaction();
session.delete( session.get(clazz, id) );
commit(session);
} catch (RuntimeException e) {
rollback(session);
throw e;
} finally {
close();
}
}
@Override
public void delete(Object o) {
Session session = null;
try {
session = beginTransaction();
session.delete( o );
commit(session);
} catch (RuntimeException e) {
rollback(session);
throw e;
} finally {
close();
}
}
@Override
public Integer deleteByValues(Class cls, String idFieldName,
String[] values) {
int res=0;
Session session = null;
try {
session = beginTransaction();
StringBuilder ins=new StringBuilder();
ins.append("'");
for (String v : values) {
ins.append(v).append("','");
}
String hql = "delete from " + cls.getSimpleName() + " where "
+ idFieldName + " in(" + ins.substring(0, ins.length()-2) + ")";
res=session.createQuery(hql).executeUpdate();
commit(session);
} catch (RuntimeException e) {
rollback(session);
throw e;
} finally {
close();
}
return res;
}
@Override
public void deleteCascadeByValues(Class cls, String fieldName, String[] values) {
Session session = null;
try {
session = beginTransaction();
StringBuilder ins=new StringBuilder();
ins.append("'");
for (String v : values) {
ins.append(v).append("','");
}
String hql = "from " + cls.getSimpleName() + " where "
+ fieldName + " in(" + ins.substring(0, ins.length()-2) + ")";
for (Object o : session.createQuery(hql).list()) {
session.delete(o);
}
commit(session);
} catch (RuntimeException e) {
rollback(session);
throw e;
} finally {
close();
}
}
@Override
public void evict(Class cls) {
HibernateSessionFactory.getSessionFactory().getCache().evictEntityRegion(cls);
}
@Override
public void evict(Class cls, Serializable id) {
HibernateSessionFactory.getSessionFactory().getCache().evictEntity(cls, id);
}
@Override
public void evictAll() {
HibernateSessionFactory.getSessionFactory().getCache().evictEntityRegions();
}
@Override
public void evictCollectionRegion(String collectionRegion) {
HibernateSessionFactory.getSessionFactory().getCache()
.evictCollectionRegion(collectionRegion);
}
@Override
public void evictCollectionRegion(String collectionRegion, Serializable id) {
HibernateSessionFactory.getSessionFactory().getCache()
.evictCollection(collectionRegion, id);
}
@Override
public void evictQueries() {
if (null != DEFAULT_QUERY_CACHE_REGION
|| (!"".equals(DEFAULT_QUERY_CACHE_REGION))) {
HibernateSessionFactory.getSessionFactory().getCache()
.evictQueryRegion(DEFAULT_QUERY_CACHE_REGION);
} else {
HibernateSessionFactory.getSessionFactory().getCache()
.evictQueryRegions();
}
}
@Override
public void evictQueries(String queryCacheRegion) {
HibernateSessionFactory.getSessionFactory().getCache().evictQueryRegion( queryCacheRegion );
}
@Override
public T find(Class clazz, Serializable id) {
Session session = null;
try {
session=HibernateSessionFactory.getSession();
return (T) session.get(clazz, id);
} catch (RuntimeException e) {
throw e;
} finally {
HibernateSessionFactory.closeSession();
}
}
@Override
public List find(String hql,EasyCriteria easyCriteria){
if(easyCriteria!=null){
return find(hql+" "+easyCriteria.getCondition(), easyCriteria.getValues().toArray());
}else{
return find(hql);
}
}
@Override
public List find(String hql, Object... values) {
Session session = null;
try {
session = HibernateSessionFactory.getSession();
Query q=createQuery(session, hql, false, null, values);
List list = q.list();
return list;
} catch (RuntimeException e) {
throw e;
} finally {
HibernateSessionFactory.closeSession();
}
}
@Override
public List findAll(Class cls) {
Session session = null;
try {
session = HibernateSessionFactory.getSession();
String queryString = "from " + cls.getName();
List list = session.createQuery(queryString).list();
initialize(list);
return list;
} catch (RuntimeException e) {
throw e;
} finally {
HibernateSessionFactory.closeSession();
}
}
@Override
public List findByCache(String hql, EasyCriteria easyCriteria){
if(easyCriteria!=null){
return findByCache(hql+" "+easyCriteria.getCondition(), easyCriteria.getValues().toArray());
}else{
return findByCache(hql);
}
}
@Override
public List findByCache(String hql, Object... values) {
Session session = null;
try {
session = HibernateSessionFactory.getSession();
String cacheRegion=null;
if (null != DEFAULT_QUERY_CACHE_REGION
|| (!"".equals(DEFAULT_QUERY_CACHE_REGION))) {
cacheRegion=DEFAULT_QUERY_CACHE_REGION;
}
Query q=createQuery(session, hql, true, cacheRegion, values);
List list = q.list();
return list;
} catch (RuntimeException e) {
throw e;
} finally {
HibernateSessionFactory.closeSession();
}
}
@Override
public List findByCache(String hql, String queryCacheRegion, EasyCriteria easyCriteria){
if(easyCriteria!=null){
return findByCache(hql+" "+easyCriteria.getCondition(), queryCacheRegion, easyCriteria.getValues().toArray());
}else{
return findByCache(hql, queryCacheRegion);
}
}
@Override
public List findByCache(String hql, String queryCacheRegion,
Object... values) {
Session session = null;
try {
session = HibernateSessionFactory.getSession();
Query q=createQuery(session, hql, true, queryCacheRegion, values);
List list = q.list();
return list;
} catch (RuntimeException e) {
throw e;
} finally {
HibernateSessionFactory.closeSession();
}
}
@Override
public void findByPage(PageBean pageBean) {
this.findByPage(pageBean,new ArrayList());
}
@Override
public void findByPage(PageBean pageBean, EasyCriteria easyCriteria) {
if(easyCriteria!=null){
pageBean.setCondition(easyCriteria.getCondition());
this.findByPage(pageBean,easyCriteria.getValues());
}else{
this.findByPage(pageBean);
}
}
@Override
public void findByPage(PageBean pageBean, List values) {
String hql = pageBean.getQuery() != null ? pageBean.getQuery()
: pageBean.getAutoQuery();
Session session = null;
try {
session = HibernateSessionFactory.getSession();
Query query=createQuery(session, hql, false, null, values.toArray());
query.setFirstResult(pageBean.getRowStart());
query.setMaxResults(pageBean.getRowsPerPage());
pageBean.setData(query.list());
String queryString = "";
int end = hql.length();
if (hql.indexOf("order by") != -1) {
end = hql.indexOf("order by");
}
if (hql.toUpperCase().indexOf("SELECT") != -1) {
int i = hql.toUpperCase()
.indexOf("FROM");
queryString = "select count(1) " + hql.substring(i, end);
} else {
queryString = "select count(1) " + hql.substring(0, end);
}
// 去掉ORDER BY 的部分
int j = queryString.toUpperCase().lastIndexOf("ORDER");
if (j != -1) {
queryString = queryString.substring(0, j);
}
Query cquery = createQuery(session, queryString, false, null, values.toArray());
int maxRow = (Integer.valueOf(cquery.uniqueResult().toString())).intValue();
pageBean.setRowsCount(maxRow);
} catch (RuntimeException e) {
throw e;
} finally {
HibernateSessionFactory.closeSession();
}
}
@Override
public List findByProperty(Class cls, String propertyName, Object value) {
String queryString = "from " + cls.getName()
+ " model where model." + propertyName + "= ?";
return find(queryString, value);
}
@Override
public List findByPropertyIgnoreCase(Class cls, String propertyName,
String value) {
String queryString = "from " + cls.getName()
+ " model where lower(model." + propertyName + ")= ?";
return find(queryString, value.toLowerCase());
}
@Override
public List findBySQL(String sql, Class resultClass, EasyCriteria easyCriteria) {
if(easyCriteria!=null){
return findBySQL(sql+" "+easyCriteria.getCondition(), resultClass, easyCriteria.getValues().toArray());
}else{
return findBySQL(sql, resultClass);
}
}
@Override
public List findBySQL(String sql, Class resultClass, Object... values) {
Session session = null;
try {
session = HibernateSessionFactory.getSession();
Query q=createNativeQuery(session, sql, resultClass, false, values);
List list = q.list();
return list;
} catch (RuntimeException e) {
throw e;
} finally {
HibernateSessionFactory.closeSession();
}
}
@Override
public List findBySQL(String sql, EasyCriteria easyCriteria) {
if(easyCriteria!=null){
return findBySQL(sql+" "+easyCriteria.getCondition(), easyCriteria.getValues().toArray());
}else{
return findBySQL(sql);
}
}
@Override
public List findBySQL(String sql, Object... values) {
Session session = null;
try {
session = HibernateSessionFactory.getSession();
Query q=createNativeQuery(session, sql,null,false,values);
List list = q.list();
return list;
} catch (RuntimeException e) {
throw e;
} finally {
HibernateSessionFactory.closeSession();
}
}
@Override
public int findCount(String hql,EasyCriteria easyCriteria) {
if(easyCriteria!=null){
return findCount(hql+" "+easyCriteria.getCondition(), easyCriteria.getValues().toArray());
}else{
return findCount(hql);
}
}
@Override
public int findCount(String hql, Object... values) {
Session session = null;
try {
session = HibernateSessionFactory.getSession();
Query q=createQuery(session, hql, false, null, values);
return Integer.valueOf(q.uniqueResult().toString());
} catch (RuntimeException e) {
throw e;
} finally {
HibernateSessionFactory.closeSession();
}
}
@Override
public List findMapResultBySQL(String sql,EasyCriteria easyCriteria){
if(easyCriteria!=null){
return findMapResultBySQL(sql+" "+easyCriteria.getCondition(), easyCriteria.getValues().toArray());
}else{
return findMapResultBySQL(sql);
}
}
@Override
public List findMapResultBySQL(String sql, Object... values){
Session session = null;
try {
session = HibernateSessionFactory.getSession();
Query q=createNativeQuery(session, sql,null,true,values);
List list = q.list();
return list;
} catch (RuntimeException e) {
throw e;
} finally {
HibernateSessionFactory.closeSession();
}
}
@Override
public int findMaxPage(String hql, int rowsPerPage, EasyCriteria easyCriteria) {
return (this.findCount(hql, easyCriteria) - 1) / rowsPerPage + 1;
}
@Override
public int findMaxPage(String hql, int rowsPerPage, Object... values) {
return (this.findCount(hql, values) - 1) / rowsPerPage + 1;
}
@Override
public List findNamedQuery(String name, Object... values) {
Session session = null;
try {
session = HibernateSessionFactory.getSession();
Query q=createNamedQuery(session, name, false, null, values);
return q.list();
} catch (RuntimeException e) {
throw e;
} finally {
HibernateSessionFactory.closeSession();
}
}
@Override
public List findNamedQueryByCache(String name, String queryCacheRegion, Object... values) {
Session session = null;
try {
session = HibernateSessionFactory.getSession();
Query q=createNamedQuery(session, name, true, queryCacheRegion, values);
return q.list();
} catch (RuntimeException e) {
throw e;
} finally {
HibernateSessionFactory.closeSession();
}
}
@Override
public List findTop( String hql, int topCount, EasyCriteria easyCriteria){
if(easyCriteria!=null){
return findTop(hql+" "+easyCriteria.getCondition(), topCount, easyCriteria.getValues().toArray());
}else{
return findTop(hql, topCount);
}
}
@Override
public List findTop(String hql, int topCount,
Object... values) {
Session session = null;
try {
session = HibernateSessionFactory.getSession();
Query q=session.createQuery(hql);
q.setFirstResult(0);
q.setMaxResults(topCount);
if (values != null && values.length > 0) {
for (int i = 0; i < values.length; i++) {
q.setParameter(i, values[i]);
}
}
List list = q.list();
return list;
} catch (RuntimeException e) {
throw e;
} finally {
HibernateSessionFactory.closeSession();
}
}
@Override
public T findVal(String hql, EasyCriteria easyCriteria) {
List list = this.find(hql, easyCriteria);
return (T) (list.size() > 0 ? list.get(0) : null);
}
@Override
public T findVal(String hql, Object... values) {
List list = this.find(hql, values);
return (T) (list.size() > 0 ? list.get(0) : null);
}
@Override
public Session getCurrentSession() {
return HibernateSessionFactory.getSession();
}
@Override
public void initialize(Object proxy) {
if (!Hibernate.isInitialized(proxy)) {
Hibernate.initialize(proxy);
}
}
@Override
public void initializeDeep(Collection collection) {
if (collection == null) {
return;
}
for (Object obj : collection) {
Method[] methods = obj.getClass().getMethods();
if (methods != null) {
for (int j = 0; j < methods.length; j++) {
String getName = methods[j].getName();
if (getName.length() > 3 && getName.startsWith("get")) {
String getFix = getName.substring(3, getName.length());
for (int k = 0; k < methods.length; k++) {
String setName = methods[k].getName();
if (setName.length() > 3
&& setName.startsWith("set")) {
String setFix = setName.substring(3,
setName.length());
if (getFix.equals(setFix)) {
try {
Object o = methods[j].invoke(obj,
new Object[0]);
if (o != null) {
Hibernate.initialize(o);
methods[k].invoke(obj, o);
}
} catch (IllegalArgumentException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
} catch (InvocationTargetException e) {
e.printStackTrace();
}
}
}
}
}
}
}
}
}
@Override
public void merge(Object o) {
Session session = null;
try {
session = beginTransaction();
session.merge(o);
commit(session);
} catch (RuntimeException e) {
rollback(session);
throw e;
} finally {
close();
}
}
@Override
public void persist(Object o) {
Session session = null;
try {
session = beginTransaction();
session.persist(o);
commit(session);
} catch (RuntimeException e) {
rollback(session);
throw e;
} finally {
close();
}
}
/**
* CommonDAO 内部方法,回滚事务
*/
private void rollback(Session session){
Transaction txn=session.getTransaction();
if ( txn != null && txn.isActive()) txn.rollback();
}
@Override
public void updateByHql(String hql, Object... values) {
Session session = null;
try {
session = beginTransaction();
Query query=session.createQuery(hql);
if (values != null && values.length > 0) {
for (int i = 0; i < values.length; i++) {
query.setParameter(i, values[i]);
}
}
query.executeUpdate();
commit(session);
} catch (RuntimeException e) {
rollback(session);
throw e;
} finally {
close();
}
}
@Override
public void updateBySQL(String sql, Object... values) {
Session session = null;
try {
session = beginTransaction();
Query query=session.createSQLQuery(sql);
if (values != null && values.length > 0) {
for (int i = 0; i < values.length; i++) {
query.setParameter(i, values[i]);
}
}
query.executeUpdate();
commit(session);
} catch (RuntimeException e) {
rollback(session);
throw e;
} finally {
close();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy