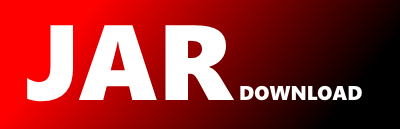
cn.eova.common.config.ConfigManager Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2023 EOVA.CN. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package cn.eova.common.config;
import java.io.FileNotFoundException;
import java.net.URL;
import java.util.HashMap;
import java.util.Properties;
import java.util.Set;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import cn.eova.tools.x;
import cn.eova.tools.file.FileUtil;
public class ConfigManager {
private static Logger logger = LoggerFactory.getLogger(ConfigManager.class);
private static HashMap props = new HashMap<>();
private static final String USER_HOME = System.getenv("HOME");
private static final String APP_ID = System.getProperty("APP_ID");
public ConfigManager(String configFiles) {
synchronized (this) {
// 默认配置
load("classpath:default", configFiles);
// 本地配置
load("classpath:dev", configFiles);
// 环境项目公共配置
if (USER_HOME != null) {
load(String.format("file:%s/config", USER_HOME), configFiles);
}
// 环境应用私有配置
if (APP_ID != null) {
load(String.format("file:%s/config/%s", USER_HOME, APP_ID), configFiles);
}
}
// String.format("");
//
// ev.str.format("");
// ev.str.formatJson("");
//
// x.str.format("");
// x.str.formatJson("");
}
public void load(String configDir, String configFiles) {
logger.info(String.format("Loading Configs:[%s]", configDir));
for (String configFile : configFiles.split(",")) {
try {
configFile = configFile.trim();
String path = String.format("%s/%s", configDir, configFile);
URL url = x.resource.getURL(path);
if (url == null) {
continue;
}
loadProperties(FileUtil.getProp(url));
logger.info(" --> " + url.toString());
} catch (FileNotFoundException e) {
continue;
} catch (Exception e) {
logger.error(String.format("加载环境配置异常:%s", e.getMessage()));
}
}
}
private void loadProperties(Properties properties) {
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy