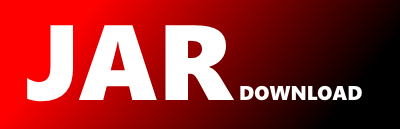
cn.fantasticmao.mundo.data.support.MemcacheLoadingCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mundo-data Show documentation
Show all versions of mundo-data Show documentation
Mundo is a tiny and out-of-the-box component for business programming.
package cn.fantasticmao.mundo.data.support;
import com.google.common.cache.AbstractLoadingCache;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.CacheStats;
import net.rubyeye.xmemcached.MemcachedClient;
import java.util.concurrent.Callable;
import java.util.concurrent.ConcurrentMap;
import java.util.concurrent.TimeUnit;
import java.util.function.Function;
/**
* MemcacheLoadingCache
*
* @author fantasticmao
* @version 1.0
* @since 2018/7/22
*/
public class MemcacheLoadingCache extends AbstractLoadingCache {
private final MemcacheClientUtil memcacheClientUtil;
private final CacheLoader cacheLoader;
private final Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy