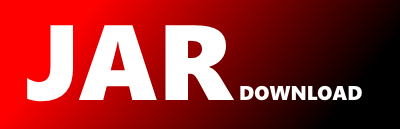
cn.featherfly.common.mqtt.EasyMqttClient Maven / Gradle / Ivy
/*
* All rights Reserved, Designed By zhongj
* @Title: EasyMqttClient.java
* @Package cn.featherfly.common.mqtt
* @Description: todo (用一句话描述该文件做什么)
* @author: zhongj
* @date: 2021-04-27 11:55:27
* @Copyright: 2021 www.featherfly.cn Inc. All rights reserved.
*/
package cn.featherfly.common.mqtt;
import java.nio.charset.Charset;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import org.eclipse.paho.client.mqttv3.IMqttDeliveryToken;
import org.eclipse.paho.client.mqttv3.MqttException;
import org.eclipse.paho.client.mqttv3.MqttMessage;
import org.eclipse.paho.client.mqttv3.MqttPersistenceException;
/**
* EasyMqttClient.
*
* @author zhongj
*/
public interface EasyMqttClient {
/**
* Gets the client id.
*
* @return the client id
*/
String getClientId();
/**
* Gets the charset.
*
* @return the charset
*/
Charset getCharset();
/**
* Connect client.
*
* @return the client
* @throws MqttException the mqtt exception
*/
EasyMqttClient connect() throws MqttException;
/**
* Subscribe.
*
* @param topicFilter the topic filter
* @param qos the qos
* @param consumer the consumer
* @return the easy client
* @throws MqttException the mqtt exception
*/
EasyMqttClient subscribe(String topicFilter, Qos qos, Consumer consumer) throws MqttException;
/**
* Subscribe client.
*
* @param topicFilter the topic filter
* @param qos the qos
* @param consumer the consumer
* @return the client
* @throws MqttException the mqtt exception
*/
EasyMqttClient subscribe(String topicFilter, Qos qos, BiConsumer consumer)
throws MqttException;
/**
* Publish client.
*
* @param topicFilter the topic
* @param msg the msg
* @return the client
* @throws MqttPersistenceException the mqtt persistence exception
* @throws MqttException the mqtt exception
*/
EasyMqttClient publish(String topicFilter, String msg) throws MqttPersistenceException, MqttException;
/**
* Publish client.
*
* @param topic the topic
* @param msg the msg
* @param consumer the consumer
* @return the client
* @throws MqttPersistenceException the mqtt persistence exception
* @throws MqttException the mqtt exception
*/
EasyMqttClient publish(String topic, String msg, Consumer consumer)
throws MqttPersistenceException, MqttException;
/**
* Publish client.
*
* @param topic the topic
* @param msg the msg
* @param qos the qos
* @return the client
* @throws MqttException the mqtt exception
*/
EasyMqttClient publish(String topic, String msg, Qos qos) throws MqttException;
/**
* Publish client.
*
* @param topic the topic
* @param msg the msg
* @param qos the qos
* @param consumer the consumer
* @return the client
* @throws MqttPersistenceException the mqtt persistence exception
* @throws MqttException the mqtt exception
*/
EasyMqttClient publish(String topic, String msg, Qos qos, Consumer consumer)
throws MqttPersistenceException, MqttException;
/**
* Publish client.
*
* @param topic the topic
* @param msg the msg
* @param qos the qos
* @param charset the charset
* @param consumer the consumer
* @return the client
* @throws MqttPersistenceException the mqtt persistence exception
* @throws MqttException the mqtt exception
*/
EasyMqttClient publish(String topic, String msg, Qos qos, Charset charset, Consumer consumer)
throws MqttPersistenceException, MqttException;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy