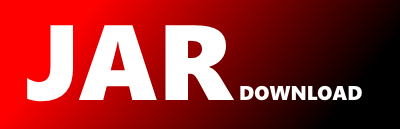
cn.featherfly.hammer.expression.condition.AbstractConditionExpression Maven / Gradle / Ivy
The newest version!
/*
* All rights Reserved, Designed By zhongj
* @Description:
* @author: zhongj
* @date: 2023-11-15 16:07:15
* @Copyright: 2023 www.featherfly.cn Inc. All rights reserved.
*/
package cn.featherfly.hammer.expression.condition;
import java.io.Serializable;
import java.util.function.Predicate;
import cn.featherfly.common.function.serializable.SerializableBooleanSupplier;
import cn.featherfly.common.function.serializable.SerializableDoubleSupplier;
import cn.featherfly.common.function.serializable.SerializableFunction;
import cn.featherfly.common.function.serializable.SerializableIntSupplier;
import cn.featherfly.common.function.serializable.SerializableLongSupplier;
import cn.featherfly.common.function.serializable.SerializablePredicate;
import cn.featherfly.common.function.serializable.SerializableSupplier;
import cn.featherfly.common.function.serializable.SerializableToDoubleFunction;
import cn.featherfly.common.function.serializable.SerializableToIntFunction;
import cn.featherfly.common.function.serializable.SerializableToLongFunction;
import cn.featherfly.common.lang.AssertIllegalArgument;
import cn.featherfly.common.lang.LambdaUtils;
import cn.featherfly.common.repository.mapping.PropertyMapping;
import cn.featherfly.hammer.config.dsl.ConditionConfig;
/**
* abstract condition expression.
*
* @author zhongj
* @param the generic type
*/
public abstract class AbstractConditionExpression> implements IgnorableExpression {
/** The condition config. */
protected final C conditionConfig;
/**
* Instantiates a new abstract muliti condition expression.
*
* @param conditionConfig the condition config
*/
protected AbstractConditionExpression(C conditionConfig) {
AssertIllegalArgument.isNotNull(conditionConfig, "conditionConfig");
this.conditionConfig = conditionConfig;
}
/**
* Gets the property name.
*
* @param name the name
* @return the property name
*/
protected String getPropertyName(Serializable name) {
return LambdaUtils.getLambdaPropertyName(name);
}
/**
* Gets the property name.
*
* @param the generic type
* @param name the name
* @return the property name
*/
protected String getPropertyName(SerializableSupplier name) {
return LambdaUtils.getLambdaPropertyName(name);
}
/**
* Gets the property name.
*
* @param name the name
* @return the property name
*/
protected String getPropertyName(SerializableIntSupplier name) {
return LambdaUtils.getLambdaPropertyName(name);
}
/**
* Gets the property name.
*
* @param name the name
* @return the property name
*/
protected String getPropertyName(SerializableLongSupplier name) {
return LambdaUtils.getLambdaPropertyName(name);
}
/**
* Gets the property name.
*
* @param name the name
* @return the property name
*/
protected String getPropertyName(SerializableDoubleSupplier name) {
return LambdaUtils.getLambdaPropertyName(name);
}
/**
* Gets the property name.
*
* @param name the name
* @return the property name
*/
protected String getPropertyName(SerializableBooleanSupplier name) {
return LambdaUtils.getLambdaPropertyName(name);
}
/**
* Gets the property name.
*
* @param the generic type
* @param the generic type
* @param name the name
* @return the property name
*/
protected String getPropertyName(SerializableFunction name) {
return LambdaUtils.getLambdaPropertyName(name);
}
/**
* Gets the property name.
*
* @param the generic type
* @param name the name
* @return the property name
*/
protected String getPropertyName(SerializableToIntFunction name) {
return LambdaUtils.getLambdaPropertyName(name);
}
/**
* Gets the property name.
*
* @param the generic type
* @param name the name
* @return the property name
*/
protected String getPropertyName(SerializableToLongFunction name) {
return LambdaUtils.getLambdaPropertyName(name);
}
/**
* Gets the property name.
*
* @param the generic type
* @param name the name
* @return the property name
*/
protected String getPropertyName(SerializableToDoubleFunction name) {
return LambdaUtils.getLambdaPropertyName(name);
}
/**
* Gets the property name.
*
* @param the generic type
* @param name the name
* @return the property name
*/
protected String getPropertyName(SerializablePredicate name) {
return LambdaUtils.getLambdaPropertyName(name);
}
/**
* Gets the in param.
*
* @param paramsForField the params for field
* @param pm the pm
* @param value the value
* @return the in param
*/
protected abstract Object getInParam(Object paramsForField, PropertyMapping pm, Object value);
/**
* Gets the in param.
*
* @param pm the pm
* @param value the value
* @return the in param
*/
protected abstract Object getInParam(PropertyMapping pm, Object value);
/**
* Gets the in param.
*
* @param value the value
* @return the in param
*/
protected abstract Object getInParam(Object value);
/**
* Gets the in param.
*
* @param paramsForField the params for field
* @param value the value
* @return the in param
*/
protected abstract Object getInParam(Object paramsForField, Object value);
/**
* {@inheritDoc}
*/
@Override
public Predicate