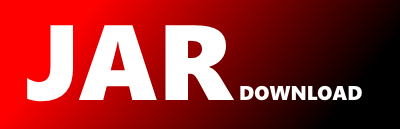
cn.featherfly.hammer.tpl.MapParamedExecutionExecutor Maven / Gradle / Ivy
The newest version!
/*
* All rights Reserved, Designed By zhongj
* @Description:
* @author: zhongj
* @date: 2024-05-10 17:17:10
* @Copyright: 2024 www.featherfly.cn Inc. All rights reserved.
*/
package cn.featherfly.hammer.tpl;
import java.io.Serializable;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
import cn.featherfly.common.lang.AutoCloseableIterable;
import cn.featherfly.common.repository.ExecutionExecutorEx;
import cn.featherfly.common.repository.ParamedExecutionExecutor;
import cn.featherfly.common.repository.ParamedMappedExecutor;
import cn.featherfly.common.repository.mapper.RowMapper;
import cn.featherfly.common.repository.mapper.TupleMapperBuilder;
import cn.featherfly.common.tuple.Tuple2;
import cn.featherfly.common.tuple.Tuple3;
import cn.featherfly.common.tuple.Tuple4;
import cn.featherfly.common.tuple.Tuple5;
import cn.featherfly.common.tuple.Tuple6;
/**
* TemplateParamedExecutionExecutor.
*
* @author zhongj
* @param the generic type
* @param the generic type
*/
public class MapParamedExecutionExecutor, E2> implements ParamedExecutionExecutor {
/** The executor. */
protected final E1 executor;
/** The execution. */
protected final E2 execution;
/** The params. */
protected final Map params;
/**
* Instantiates a new template paramed execution executor.
*
* @param executor the executor
* @param execution the execution
* @param params the params
*/
public MapParamedExecutionExecutor(E1 executor, E2 execution, Map params) {
super();
this.executor = executor;
this.execution = execution;
this.params = params;
}
/**
* {@inheritDoc}
*/
@Override
public ParamedMappedExecutor mapper(Class mapType) {
return new PrefixedBeanMapper1Impl<>(executor, execution, params, mapType);
}
/**
* {@inheritDoc}
*/
@Override
public ParamedMappedExecutor> mapper(Class type1, Class type2) {
return new PrefixedBeanMapper2Impl<>(executor, execution, params, type1, type2);
}
/**
* {@inheritDoc}
*/
@Override
public ParamedMappedExecutor> mapper(Class type1, Class type2,
Class type3) {
return new PrefixedBeanMapper3Impl<>(executor, execution, params, type1, type2, type3);
}
/**
* {@inheritDoc}
*/
@Override
public ParamedMappedExecutor> mapper(Class type1, Class type2,
Class type3, Class type4) {
return new PrefixedBeanMapper4Impl<>(executor, execution, params, type1, type2, type3, type4);
}
/**
* {@inheritDoc}
*/
@Override
public ParamedMappedExecutor> mapper(Class type1,
Class type2, Class type3, Class type4, Class type5) {
return new PrefixedBeanMapper5Impl<>(executor, execution, params, type1, type2, type3, type4, type5);
}
/**
* {@inheritDoc}
*/
@Override
public ParamedMappedExecutor> mapper(Class type1,
Class type2, Class type3, Class type4, Class type5, Class type6) {
return new PrefixedBeanMapper6Impl<>(executor, execution, params, type1, type2, type3, type4, type5, type6);
}
/**
* {@inheritDoc}
*/
@Override
public ParamedMappedExecutor mapper(
Function> mapperBuilderFunction) {
return mapperBuilderFunction.apply(new MapParamsTupleMapperBuilder<>(executor, execution, params));
}
/**
* {@inheritDoc}
*/
@Override
public int execute() {
return executor.execute(execution, params);
}
/**
* {@inheritDoc}
*/
@Override
public int intValue() {
return executor.intValue(execution, params);
}
/**
* {@inheritDoc}
*/
@Override
public long longValue() {
return executor.longValue(execution, params);
}
/**
* {@inheritDoc}
*/
@Override
public double doubleValue() {
return executor.doubleValue(execution, params);
}
/**
* {@inheritDoc}
*/
@Override
public boolean bool() {
return executor.bool(execution, params);
}
/**
* {@inheritDoc}
*/
@Override
public V value() {
return executor.value(execution, params);
}
/**
* {@inheritDoc}
*/
@Override
public V value(Class valueType) {
return executor.value(execution, valueType, params);
}
/**
* {@inheritDoc}
*/
@Override
public Map single() {
return executor.single(execution, params);
}
/**
* {@inheritDoc}
*/
@Override
public T single(Class mapType) {
return executor.single(execution, mapType, params);
}
/**
* {@inheritDoc}
*/
@Override
public T single(RowMapper rowMapper) {
return executor.single(execution, rowMapper, params);
}
/**
* {@inheritDoc}
*/
@Override
public Map unique() {
return executor.unique(execution, params);
}
/**
* {@inheritDoc}
*/
@Override
public T unique(Class mapType) {
return executor.unique(execution, mapType, params);
}
/**
* {@inheritDoc}
*/
@Override
public T unique(RowMapper rowMapper) {
return executor.unique(execution, rowMapper, params);
}
/**
* {@inheritDoc}
*/
@Override
public List