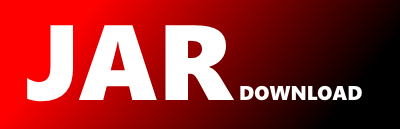
cn.featherfly.hammer.tpl.PrefixedBeanMapper1Impl Maven / Gradle / Ivy
The newest version!
/*
* All rights Reserved, Designed By zhongj
* @Description:
* @author: zhongj
* @date: 2022-05-10 18:29:10
* @Copyright: 2022 www.featherfly.cn Inc. All rights reserved.
*/
package cn.featherfly.hammer.tpl;
import java.io.Serializable;
import java.util.List;
import java.util.Map;
import cn.featherfly.common.lang.AutoCloseableIterable;
import cn.featherfly.common.repository.ExecutionExecutorEx;
import cn.featherfly.common.repository.mapper.PrefixedBeanMapper1;
import cn.featherfly.common.repository.mapper.PrefixedBeanMapper2;
import cn.featherfly.common.structure.page.PaginationResults;
import cn.featherfly.common.tuple.Tuple1;
import cn.featherfly.common.tuple.Tuples;
/**
* The Class PrefixedBeanMapper1Impl.
*
* @author zhongj
* @param the generic type
* @param the generic type
* @param the generic type
*/
public class PrefixedBeanMapper1Impl, E2, T1>
extends AbstractPrefixEntityMapper> implements PrefixedBeanMapper1 {
private final Class type1;
/**
* Instantiates a new tpl prefix entity mapper 1.
*
* @param executor the executor
* @param execution the execution
* @param params the params
* @param type1 the type 1
*/
public PrefixedBeanMapper1Impl(E1 executor, E2 execution, Map params, Class type1) {
this(executor, execution, params, "", type1);
}
/**
* Instantiates a new tpl prefix entity mapper 1.
*
* @param executor the executor
* @param execution the execution
* @param params the params
* @param prefix the prefix
* @param type1 the type 1
*/
public PrefixedBeanMapper1Impl(E1 executor, E2 execution, Map params, String prefix,
Class type1) {
super(executor, execution, params, Tuples.of(prefix));
this.type1 = type1;
}
/**
* Instantiates a new tpl prefix entity mapper 1.
*
* @param executor the executor
* @param execution the execution
* @param params the params
* @param type1 the type 1
*/
public PrefixedBeanMapper1Impl(E1 executor, E2 execution, Object[] params, Class type1) {
this(executor, execution, params, "", type1);
}
/**
* Instantiates a new tpl prefix entity mapper 1.
*
* @param executor the executor
* @param execution the execution
* @param params the params
* @param prefix the prefix
* @param type1 the type 1
*/
public PrefixedBeanMapper1Impl(E1 executor, E2 execution, Object[] params, String prefix, Class type1) {
super(executor, execution, params, Tuples.of(prefix));
this.type1 = type1;
}
/**
* {@inheritDoc}
*/
@Override
public T1 single() {
if (params instanceof Map) {
return executor.single(execution, type1, getParamsMap());
} else {
return executor.single(execution, type1, getParams());
}
}
/**
* {@inheritDoc}
*/
@Override
public T1 unique() {
if (params instanceof Map) {
return executor.unique(execution, type1, getParamsMap());
} else {
return executor.unique(execution, type1, getParams());
}
}
/**
* {@inheritDoc}
*/
@Override
public List list() {
if (params instanceof Map) {
return executor.list(execution, type1, getParamsMap());
} else {
return executor.list(execution, type1, getParams());
}
}
/**
* {@inheritDoc}
*/
@Override
public List list(int offset, int limit) {
if (params instanceof Map) {
return executor.list(execution, type1, getParamsMap(), offset, limit);
} else {
return executor.list(execution, type1, getParams(), offset, limit);
}
}
/**
* {@inheritDoc}
*/
@Override
public AutoCloseableIterable each() {
if (params instanceof Map) {
return executor.each(execution, type1, getParamsMap());
} else {
return executor.each(execution, type1, getParams());
}
}
/**
* {@inheritDoc}
*/
@Override
public AutoCloseableIterable each(int offset, int limit) {
if (params instanceof Map) {
return executor.each(execution, type1, getParamsMap(), offset, limit);
} else {
return executor.each(execution, type1, getParams(), offset, limit);
}
}
/**
* {@inheritDoc}
*/
@Override
public PaginationResults pagination(int offset, int limit) {
if (params instanceof Map) {
return executor.pagination(execution, type1, getParamsMap(), offset, limit);
} else {
return executor.pagination(execution, type1, getParams(), offset, limit);
}
}
/**
* {@inheritDoc}
*/
@Override
public PrefixedBeanMapper2 map(String prefix, Class type) {
if (params instanceof Map) {
return new PrefixedBeanMapper2Impl<>(executor, execution, getParamsMap(), type1, type,
Tuples.of(prefixes.get0(), prefix));
} else {
return new PrefixedBeanMapper2Impl<>(executor, execution, getParams(), type1, type,
Tuples.of(prefixes.get0(), prefix));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy