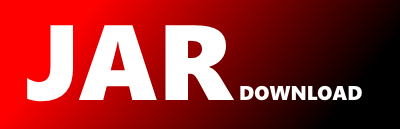
cn.featherfly.common.lang.LangUtils Maven / Gradle / Ivy
package cn.featherfly.common.lang;
import java.io.File;
import java.lang.reflect.Array;
import java.util.Collection;
import java.util.Map;
/**
*
*
* 对一些在语法上显得拖沓的常用操作进行封装的工具类
*
* @author 钟冀
* @since 1.0
* @version 1.0
*/
public final class LangUtils {
private LangUtils() {
}
/**
*
* 如果第一个参数为空(null),返回第二个参数,否则返回第一个参数
*
* @param 泛型
* @param target 目标参数
* @param defaultTarget 默认值
* @return 第一个参数为空(null),返回第二个参数,否则返回第一个参数
*/
public static T pick(T target, T defaultTarget) {
return target == null ? defaultTarget : target;
}
/**
*
* 返回第一个非空的项,!=null
*
*
* @param
* 泛型
* @param pickedItems
* 需要选择的对象
* @return 第一个非空的对象
*/
@SafeVarargs
public static T pickFirst(T...pickedItems) {
if (pickedItems != null) {
for (T t : pickedItems) {
if (t != null) {
return t;
}
}
}
return null;
}
/**
*
* 返回最后一个非空的对象,!=null
*
* @param
* 泛型
* @param pickedItems
* 需要选择的对象
* @return 最后一个非空的对象
*/
@SafeVarargs
public static T pickLast(T...pickedItems) {
if (pickedItems != null) {
for (int i = pickedItems.length - 1; i >= 0; i--) {
T t = pickedItems[i];
if (t != null) {
return t;
}
}
}
return null;
}
/**
*
* 返回传入对象是否为空,(String、Collection、Map、Array还要判断长度是否为0)
*
* @param object
* 传入的对象
* @return 传入对象是否为空
*/
public static boolean isEmpty(Object object) {
if (object == null) {
return true;
}
if (object instanceof String) {
return isEmpty((String) object);
}
if (object instanceof Collection) {
return isEmpty((Collection>) object);
}
if (object instanceof Map) {
return isEmpty((Map, ?>) object);
}
if (object.getClass().isArray()) {
return Array.getLength(object) == 0;
}
return false;
}
/**
*
* 返回传入对象是否不为空(String、Collection、Map、Array还要判断长度是否为0)
*
* @param object
* 传入的对象
* @return 传入对象是否不为空
*/
public static boolean isNotEmpty(Object object) {
return !isEmpty(object);
}
/**
*
* 返回传入字符串是否为空(是null或是空字符串)
*
* @param string
* 传入的字符串
* @return 传入字符串是否为空
*/
public static boolean isEmpty(String string) {
return string == null || "".equals(string);
}
/**
*
* 返回传入字符串是否不为空(不是null或不是空字符串)
*
* @param string
* 传入的字符串
* @return 传入字符串是否不为空
*/
public static boolean isNotEmpty(String string) {
return !isEmpty(string);
}
/**
*
* 返回数组是否为空(是null或是空数组)
*
* @param array
* 传入的数组
* @return 传入数组是否为空
*/
public static boolean isEmpty(Object[] array) {
return array == null || array.length == 0;
}
/**
*
* 返回数组是否不为空(null或空数组)
*
* @param array
* 传入的数组
* @return 传入数组是否不为空
*/
public static boolean isNotEmpty(Object[] array) {
return !isEmpty(array);
}
/**
*
* 返回传入集合是否为空(是null或size=0)
*
* @param collection
* 传入的集合
* @return 传入集合是否为空
*/
public static boolean isEmpty(Collection> collection) {
return collection == null || collection.isEmpty();
}
/**
*
* 返回传入集合是否不为空(不是null或size>0)
*
* @param collection
* 传入的集合
* @return 传入集合是否不为空
*/
public static boolean isNotEmpty(Collection> collection) {
return !isEmpty(collection);
}
/**
*
* 返回传入map是否为空(是null或size=0)
*
* @param map
* 传入的map
* @return 传入map是否为空
*/
public static boolean isEmpty(Map, ?> map) {
return map == null || map.isEmpty();
}
/**
*
* 返回传入map是否不为空(不是null或size>0)
*
* @param map
* 传入的map
* @return 传入map是否不为空
*/
public static boolean isNotEmpty(Map, ?> map) {
return !isEmpty(map);
}
/**
*
* 判断传入文件对象代表的物理文件是否存在
*
* @param file 判断的文件
* @return 传入文件对象代表的物理文件是否存在
*/
public static boolean isExists(File file) {
return file != null && file.exists();
}
/**
*
* 将传入对象转换为枚举
*
* @param toClass 枚举的类型
* @param object 需要转换的对象
* @param 泛型
* @return 转换后的枚举,如果是无法转换或不存在的枚举类型,则返回null
*/
public static > T toEnum(Class toClass, Object object) {
if (object != null) {
if (object instanceof String[]) {
return Enum.valueOf(toClass, ((String[]) object)[0]);
} else if (object instanceof String) {
return Enum.valueOf(toClass, (String) object);
} else if (object instanceof Integer || object.getClass() == int.class) {
Integer ordinal = (Integer) object;
T[] es = (T[]) toClass.getEnumConstants();
for (T e : es) {
if (e.ordinal() == ordinal) {
return (T) e;
}
}
}
}
return null;
}
/**
*
* 安全的equals,防止空指针异常
*
* @param target 比较对象
* @param otherTarget 另一个比较对象
* @return 比较结果
*/
public static boolean equals(Object target, Object otherTarget) {
return (target == otherTarget) || (target != null && target.equals(otherTarget));
}
/**
* 返回hash code,如果传入参数为null,返回0.
* @param o 对象
* @return hash code
* @see Object#hashCode
*/
public static int hashCode(Object o) {
return o != null ? o.hashCode() : 0;
}
/**
*
* 转换为String,如果不能转换(null)则使用默认值
*
* @param obj obj
* @param defaultValue defaultValue
* @return string
*/
public static String toString(Object obj, String defaultValue) {
return obj != null ? obj.toString() : defaultValue;
}
/**
*
* 转换为String,如果不能转换(null)则返回null
*
* @param obj obj
* @return string
*/
public static String toString(Object obj) {
return toString(obj, null);
}
}