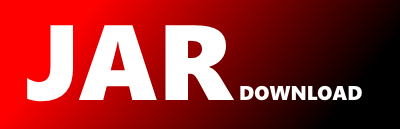
cn.featherfly.common.structure.tree.component.MifTreeNode Maven / Gradle / Ivy
/**
* @author 钟冀 - yufei
* Aug 25, 2009
*/
package cn.featherfly.common.structure.tree.component;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonIgnore;
/**
*
* MifTree节点
*
* @author 钟冀
*/
public class MifTreeNode {
private static final String NAME_KEY = "name";
private static final String OPEN_KEY = "open";
private static final String CHECKED_KEY = "checked";
private static final String ID_KEY = "id";
private static final String LOADABLE_KEY = "loadable";
/**
*/
public MifTreeNode() {
}
/**
*
* 添加属性
*
* @param name 属性名
* @param value 属性值
*/
public void addProperty(String name, Object value) {
getProperty().put(name, value);
}
/**
*
* 添加状态
*
* @param name 名
* @param value 值
*/
public void addState(String name, Object value) {
getState().put(name, value);
}
/**
*
* 添加数据
*
* @param name 名
* @param value 值
*/
public void addData(String name, Object value) {
getData().put(name, value);
}
/**
*
* 添加子节点
*
* @param child 子节点
*/
public void addChild(MifTreeNode child) {
children.add(child);
}
/**
*
* 设置id
*
* @param id id
*/
public void setId(String id) {
getData().put(ID_KEY, id);
}
/**
*
* 返回id
*
* @return id
*/
@JsonIgnore
public String getId() {
Object id = getData().get(ID_KEY);
if (id != null) {
return id.toString();
}
return null;
}
/**
*
* 设置名称
*
* @param name 名称
*/
public void setName(String name) {
getProperty().put(NAME_KEY, name);
}
/**
*
* 返回name
*
* @return name
*/
@JsonIgnore
public String getName() {
Object name = getProperty().get(NAME_KEY);
if (name != null) {
return name.toString();
}
return null;
}
/**
*
* 设置节点是否加载
*
* @param loadable 节点是否加载
*/
public void setLoadable(boolean loadable) {
getProperty().put(LOADABLE_KEY, loadable);
}
/**
*
* 返回节点是否加载
*
* @return 节点是否加载
*/
@JsonIgnore
public boolean isLoadable() {
return Boolean.parseBoolean(getProperty().get(LOADABLE_KEY) + "");
}
/**
*
* 设置是否展开
*
* @param open 是否展开
*/
public void setOpen(boolean open) {
getState().put(OPEN_KEY, true);
}
/**
*
* 返回是否展开
*
* @return 是否展开
*/
@JsonIgnore
public boolean isOpen() {
return Boolean.parseBoolean(getState().get(OPEN_KEY) + "");
}
/**
*
* 设置是否选中
*
* @param checked 是否选中
*/
public void setChecked(boolean checked) {
if (checked) {
getState().put(CHECKED_KEY, "checked");
} else {
getState().put(CHECKED_KEY, "unchecked");
}
}
/**
*
* 返回是否选中
*
* @return 是否选中
*/
@JsonIgnore
public boolean isChecked() {
return "checked".equals(getState().get(CHECKED_KEY) + "");
}
// ********************************************************************
// property
// ********************************************************************
private Map property = new HashMap(0);
private Map state = new HashMap(0);
private Map data = new HashMap(0);
private String type;
private List children = new ArrayList(0);
/**
* 返回children
* @return children
*/
public List getChildren() {
return children;
}
/**
* 设置children
* @param children children
*/
public void setChildren(List children) {
this.children = children;
}
/**
* 返回property
* @return property
*/
public Map getProperty() {
return property;
}
/**
* 设置property
* @param property property
*/
public void setProperty(Map property) {
this.property = property;
}
/**
* 返回state
* @return state
*/
public Map getState() {
return state;
}
/**
* 设置state
* @param state state
*/
public void setState(Map state) {
this.state = state;
}
/**
* 返回data
* @return data
*/
public Map getData() {
return data;
}
/**
* 设置data
* @param data data
*/
public void setData(Map data) {
this.data = data;
}
/**
* 返回type
* @return type
*/
public String getType() {
return type;
}
/**
* 设置type
* @param type type
*/
public void setType(String type) {
this.type = type;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy