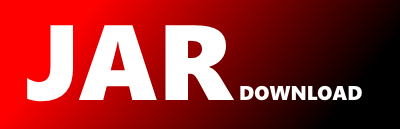
cn.featherfly.data.office.excel.ExcelObjectMapper Maven / Gradle / Ivy
package cn.featherfly.data.office.excel;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.FormulaEvaluator;
import org.apache.poi.ss.usermodel.Row;
import cn.featherfly.common.bean.BeanDescriptor;
import cn.featherfly.common.bean.BeanUtils;
import cn.featherfly.common.lang.ClassUtils;
import cn.featherfly.common.lang.Lang;
import cn.featherfly.common.lang.NumberUtils;
import cn.featherfly.common.lang.reflect.GenericClass;
/**
*
* ExcelSwaggerModelMapper
*
*
* @param 要映射的具体类
* @author 钟冀
*/
public class ExcelObjectMapper extends ExcelDataMapper {
private Map columnPropertyNameMap;
private List titles;
private Class type;
/**
* @param type Record Type Class
*/
public ExcelObjectMapper(Class type) {
this(type, new HashMap<>(), new ArrayList<>());
}
/**
* @param type Record Type Class
* @param columnPropertyNameMap columnPropertyNameMap
*/
public ExcelObjectMapper(Class type, Map columnPropertyNameMap) {
this(type, columnPropertyNameMap, new ArrayList<>());
}
/**
* @param type Record Type Class
* @param columnPropertyNameMap columnPropertyNameMap
* @param titles titles
*/
public ExcelObjectMapper(Class type, Map columnPropertyNameMap, List titles) {
this.type = type;
this.columnPropertyNameMap = columnPropertyNameMap;
this.titles = titles;
}
/**
* {@inheritDoc}
*/
@SuppressWarnings("unchecked")
@Override
public R mapRecord(Row row, int rowNum) {
R record = ClassUtils.newInstance(type);
if (row != null) {
FormulaEvaluator evaluator = getFormulaEvaluator(row.getSheet().getWorkbook());
BeanDescriptor bd = BeanDescriptor.getBeanDescriptor(type);
for (Entry columnPropertyName : columnPropertyNameMap.entrySet()) {
Cell cell = row.getCell(columnPropertyName.getKey());
if (cell != null) {
Object value = getCellValue(cell, evaluator);
Class> propertyType = bd.getBeanProperty(columnPropertyName.getValue()).getType();
if (value.getClass() != propertyType) {
if (value instanceof Number) {
value = NumberUtils.convert((Number) value, (Class) propertyType);
} else {
value = conversion.targetToSource(value.toString(), new GenericClass<>(propertyType));
}
}
BeanUtils.setProperty(record, columnPropertyName.getValue(), value);
} else {
logger.debug("cell of column[{}] is null", columnPropertyName.getKey());
}
}
}
return record;
}
/**
* {@inheritDoc}
*/
@Override
public void fillData(Row row, R record, int rowNum) {
if (Lang.isNotEmpty(titles) && rowNum == 0) {
for (int i = 0; i < titles.size(); i++) {
String title = titles.get(i);
Cell cell = row.createCell(i);
setCellValue(title, cell);
}
row = row.getSheet().createRow(rowNum + 1);
}
if (record != null) {
for (Entry columnPropertyName : columnPropertyNameMap.entrySet()) {
Object value = BeanUtils.getProperty(record, columnPropertyName.getValue());
Cell cell = row.createCell(columnPropertyName.getKey());
setCellValue(value, cell);
}
}
}
/**
* 返回columnPropertyNameMap
*
* @return columnPropertyNameMap
*/
public Map getColumnPropertyNameMap() {
return columnPropertyNameMap;
}
/**
* 设置columnPropertyNameMap
*
* @param columnPropertyNameMap columnPropertyNameMap
*/
public void setColumnPropertyNameMap(Map columnPropertyNameMap) {
this.columnPropertyNameMap = columnPropertyNameMap;
}
/**
* 返回type
*
* @return type
*/
public Class getType() {
return type;
}
/**
* 返回titles
*
* @return titles
*/
public List getTitles() {
return titles;
}
/**
* 设置titles
*
* @param titles titles
*/
public void setTitles(List titles) {
this.titles = titles;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy