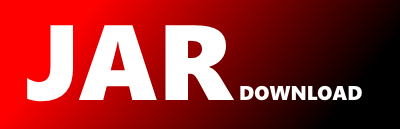
cn.featherfly.common.net.http.HttpRequest Maven / Gradle / Ivy
package cn.featherfly.common.net.http;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.Charset;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import org.apache.commons.io.Charsets;
import org.apache.commons.io.IOUtils;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpDelete;
import org.apache.http.client.methods.HttpEntityEnclosingRequestBase;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.client.methods.HttpPut;
import org.apache.http.client.methods.HttpRequestBase;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.entity.mime.content.StringBody;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.message.BasicNameValuePair;
import cn.featherfly.common.lang.Assert;
import cn.featherfly.common.lang.AssertIllegalArgument;
import cn.featherfly.common.lang.LangUtils;
import cn.featherfly.common.net.NetException;
/**
*
* Http请求
*
*
* @author 钟冀
*/
public class HttpRequest {
private static final Assert asserts = new Assert(NetException.class);
public static void main(String[] args) throws Exception {
HttpRequest request = new HttpRequest("http://wwww.baidu.com", HttpClientBuilder.create().build());
HttpResponse response = request.execute(HttpMethod.GET);
HttpEntity responseEntity = response.getEntity();
InputStream content = responseEntity.getContent();
List values = IOUtils.readLines(content, "UTF-8");
StringBuilder value = new StringBuilder();
for (String v : values) {
value.append(v).append("\n");
}
System.out.println("http code : " + response.getStatusLine().getStatusCode());
System.out.println(value.toString());
}
private HttpClient httpClient;
/**
* @param url 请求地址
*/
public HttpRequest(String url) {
this(url, HttpClientBuilder.create().build());
}
/**
* @param url 请求地址
* @param httpClient httpClient
*/
public HttpRequest(String url, HttpClient httpClient) {
AssertIllegalArgument.isNotEmpty(url, "url不能为空");
AssertIllegalArgument.isNotEmpty(httpClient, "httpClient不能为空");
this.url = url;
this.httpClient = httpClient;
}
/**
*
* 添加参数
*
* @param name 参数名
* @param values 参数值
*/
public HttpRequest addParam(String name, String...values) {
if (values != null) {
List valueList = this.params.get(name);
if (valueList == null) {
valueList = new ArrayList();
this.params.put(name, valueList);
}
Collections.addAll(valueList, values);
}
return this;
}
/**
*
* 添加参数(文件)
*
* @param name 参数名
* @param files 文件
*/
public HttpRequest addParam(String name, File...files) {
if (files != null) {
List valueList = this.files.get(name);
if (valueList == null) {
valueList = new ArrayList();
this.files.put(name, valueList);
}
Collections.addAll(valueList, files);
}
return this;
}
/**
*
* 添加请求头
*
* @param name 请求名
* @param values 参数值
*/
public HttpRequest addHeader(String name, String...values) {
if (values != null) {
List valueList = this.headers.get(name);
if (valueList == null) {
valueList = new ArrayList();
this.headers.put(name, valueList);
}
Collections.addAll(valueList, values);
}
return this;
}
/**
*
* 请求(使用method作用请求方式)
*
* @return 请求结果
*/
public HttpResponse execute() {
return execute(method);
}
/**
*
* 请求(使用传入method作用请求方式)
*
* @param method HttpMethod
* @return 请求结果
*/
public HttpResponse execute(HttpMethod method) {
AssertIllegalArgument.isNotNull(method, "method不能为空");
HttpResponse response = null;
switch(method) {
case GET: response = get(); break;
case POST : response = post(); break;
case PUT: response = put(); break;
case DELETE: response = delete(); break;
default :
throw new NetException("#http.method.no.support", new Object[] {method});
}
return response;
}
/**
*
* get请求
*
* @return 请求结果
*/
public HttpResponse get() {
String requestUrl = url;
if (!requestUrl.contains("?")) {
requestUrl += "?";
}
for (Entry> entry : getParams().entrySet()) {
String name = entry.getKey();
List values = entry.getValue();
for (String value : values) {
requestUrl += name + "=" + value + "&";
}
}
HttpGet httpGet = new HttpGet(requestUrl);
setHeaders(httpGet);
try {
return httpClient.execute(httpGet);
} catch (IOException e) {
throw new NetException(e);
}
}
/**
*
* post请求.
* 不会验证file对象,当file对象非法(为空或不存在)时将忽略
*
* @return 请求结果
*/
public HttpResponse post() {
return post(this.params, this.files);
}
public HttpResponse put() {
try {
HttpPut put = new HttpPut(url);
setParam(put);
setHeaders(put);
return httpClient.execute(put);
} catch (Exception e) {
throw new NetException(e);
}
}
public HttpResponse delete() {
try {
HttpDelete delete = new HttpDelete(url);
setHeaders(delete);
return httpClient.execute(delete);
} catch (Exception e) {
throw new NetException(e);
}
}
/**
*
* 上传文件.
* 会验证file对象,当file对象非法(为空或不存在)时会抛出异常
*
* @return 结果
*/
public HttpResponse upload() {
asserts.isNotEmpty(this.files, "#http.upload.argu.null");
for (Entry> entry : files.entrySet()) {
List files = entry.getValue();
for (File file : files) {
asserts.isNotNull(file, "#http.upload.file.null");
if (!LangUtils.isExists(file)) {
throw new NetException("#http.upload.file.exists", new Object[] {file.getAbsolutePath()});
}
}
}
// AssertIllegalArgument.isNotEmpty(this.files, "尼玛! 没有文件对象传入你上传个毛啊!");
// for (Entry> entry : files.entrySet()) {
// List files = entry.getValue();
// for (File file : files) {
// AssertIllegalArgument.isNotNull(file, "哎哟喂,文件对象为空你让我如何上传!");
// AssertIllegalArgument.isExists(file, "哦买糕的,这货[" + file.getAbsolutePath() + "]根本不存在!");
// }
// }
return post(this.params, this.files);
}
// ********************************************************************
// private method
// ********************************************************************
private HttpResponse post(Map> params, Map> files) {
HttpPost httpPost = new HttpPost(url);
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
try {
Charset charset = Charset.forName("UTF-8") ;
// 请求参数
for (Entry> entry : params.entrySet()) {
String name = entry.getKey();
List values = entry.getValue();
for (String value : values) {
builder.addPart(name, new StringBody(value, ContentType.create("text/plain", charset)));
}
}
// 文件
if (files != null) {
for (Entry> entry : files.entrySet()) {
String name = entry.getKey();
List values = entry.getValue();
if (values != null) {
for (File file : values) {
if (file != null && file.exists()) {
builder.addBinaryBody(name, file);
}
}
}
}
}
setHeaders(httpPost);
httpPost.setEntity(builder.build());
return httpClient.execute(httpPost);
} catch (IOException e) {
throw new NetException(e);
}
}
private void setHeaders(HttpRequestBase httpRequest) {
for (Entry> entry : getHeaders().entrySet()) {
String name = entry.getKey();
List values = entry.getValue();
for (String value : values) {
httpRequest.addHeader(name, value);
}
}
}
private void setParam(HttpEntityEnclosingRequestBase request) {
request.setEntity(new UrlEncodedFormEntity(createNameValuePairs(), charset));
}
private List createNameValuePairs() {
List nameValuePairList = new ArrayList();
//设置请求参数
if (LangUtils.isNotEmpty(params)) {
Set>> set = params.entrySet();
Iterator>> iterator = set.iterator();
while (iterator.hasNext()) {
Entry> entry = iterator.next();
for (Object value : entry.getValue()) {
BasicNameValuePair basicNameValuePair = new BasicNameValuePair(entry.getKey(), value.toString());
nameValuePairList.add(basicNameValuePair);
}
}
}
return nameValuePairList;
}
// ********************************************************************
// property
// ********************************************************************
private HttpMethod method = HttpMethod.GET;
private String url;
private Charset charset = Charsets.UTF_8;
private Map> params = new HashMap>();
private Map> headers = new HashMap>();
private Map> files = new HashMap>();
/**
* 返回charset
* @return charset
*/
public Charset getCharset() {
return charset;
}
/**
* 设置charset
* @param charset charset
*/
public void setCharset(Charset charset) {
this.charset = charset;
}
/**
* 返回method
* @return method
*/
public HttpMethod getMethod() {
return method;
}
/**
* 设置method
* @param method method
*/
public void setMethod(HttpMethod method) {
this.method = method;
}
/**
* 返回url
* @return url
*/
public String getUrl() {
return url;
}
/**
* 返回params
* @return params
*/
public Map> getParams() {
return params;
}
/**
* 返回headers
* @return headers
*/
public Map> getHeaders() {
return headers;
}
/**
* 返回files
* @return files
*/
public Map> getFiles() {
return files;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy