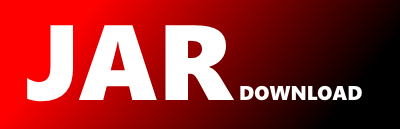
cn.featherfly.common.net.mail.client.MailSender Maven / Gradle / Ivy
package cn.featherfly.common.net.mail.client;
import java.io.UnsupportedEncodingException;
import java.util.Date;
import java.util.Map;
import java.util.Map.Entry;
import javax.activation.DataHandler;
import javax.activation.DataSource;
import javax.activation.FileDataSource;
import javax.mail.BodyPart;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.Multipart;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeBodyPart;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.MimeMultipart;
import javax.mail.internet.MimeUtility;
import cn.featherfly.common.lang.AssertIllegalArgument;
import cn.featherfly.common.lang.UUIDGenerator;
import cn.featherfly.common.net.NetException;
import cn.featherfly.common.net.mail.MailAddress;
import cn.featherfly.common.net.mail.MailApiUtils;
import cn.featherfly.common.net.mail.MailAttach;
import cn.featherfly.common.net.mail.MailBody;
import cn.featherfly.common.net.mail.MailUser;
import cn.featherfly.common.net.mail.SmtpMailServer;
/**
*
* 邮件发送客户端
*
*
* @author 钟冀
*/
public class MailSender extends AbstractMailClient{
/**
*
* @param mailUser mailUser
* @param smtpServer smtpServer
*/
public MailSender(MailUser mailUser, SmtpMailServer smtpServer) {
this(mailUser, smtpServer, null);
}
/**
*
* @param mailUser mailUser
* @param smtpServer smtpServer
* @param props props
*/
public MailSender(MailUser mailUser, SmtpMailServer smtpServer, Map props) {
super(mailUser, smtpServer, null, props);
AssertIllegalArgument.isNotNull(mailUser, "mailUser不能为空");
AssertIllegalArgument.isNotNull(smtpServer, "smtpServer不能为空");
}
/**
*
* 发送邮件
*
* @throws MessagingException
*/
public void send(MailAddress mailAddress, MailBody mailBody) {
Transport transport = null;
try {
setDebug();
Message message = createMessage(mailAddress, mailBody);
transport = getSession().getTransport(getSmtpServer().getProtocol());
// 连接服务器的邮箱
transport.connect(getSmtpServer().getHost(), getSmtpServer().getPort(), getMailUser().getUsername(), getMailUser().getPassword());
/*transport.addTransportListener(new TransportListener() {
@Override
public void messagePartiallyDelivered(TransportEvent e) {
System.out.println("****************************************");
System.out.println("messagePartiallyDelivered");
if (e.getInvalidAddresses() != null) {
System.out.println(e.getInvalidAddresses().length);
System.out.println(Arrays.asList(e.getInvalidAddresses()));
}
}
@Override
public void messageNotDelivered(TransportEvent e) {
System.out.println("****************************************");
System.out.println("messageNotDelivered");
if (e.getInvalidAddresses() != null) {
System.out.println(e.getInvalidAddresses().length);
System.out.println(Arrays.asList(e.getInvalidAddresses()));
}
}
@Override
public void messageDelivered(TransportEvent e) {
System.out.println("****************************************");
System.out.println("messageDelivered");
System.out.println("--------------------------------");
System.out.println("InvalidAddresses");
System.out.println(e.getInvalidAddresses() == null);
if (e.getInvalidAddresses() != null) {
System.out.println(e.getInvalidAddresses().length);
System.out.println(Arrays.asList(e.getInvalidAddresses()));
}
System.out.println("--------------------------------");
System.out.println("ValidSentAddresses");
System.out.println(e.getValidSentAddresses() == null);
if (e.getValidSentAddresses() != null) {
System.out.println(e.getValidSentAddresses().length);
System.out.println(Arrays.asList(e.getValidSentAddresses()));
}
System.out.println("--------------------------------");
System.out.println("ValidUnsentAddresses");
System.out.println(e.getValidUnsentAddresses() == null);
if (e.getValidUnsentAddresses() != null) {
System.out.println(e.getValidUnsentAddresses().length);
System.out.println(Arrays.asList(e.getValidUnsentAddresses()));
}
}
});*/
// 发送邮件
transport.sendMessage(message, message.getAllRecipients());
// transport.close();
} catch (MessagingException e) {
throw new NetException(e);
} finally {
MailApiUtils.close(transport, logger);
}
}
// ********************************************************************
// private method
// ********************************************************************
// 构造邮件的内容
private Message createMessage(MailAddress mailAddress, MailBody mailBody) throws MessagingException {
AssertIllegalArgument.isNotNull(mailAddress, "未设置邮件地址对象[mailAddress]");
AssertIllegalArgument.isNotEmpty(mailAddress.getTo(),
"邮件地址列表为空[mailAddress.getTo() is nul or size = 0]");
AssertIllegalArgument.isNotNull(mailBody, "未设置邮件[mailBody]");
AssertIllegalArgument.isNotNull(mailBody.getSubject(), "未设置邮件标题[mailBody.subject]");
// AssertIllegalArgument.isNotNull(mailBody.getContent(), "未设置邮件正文[mailBody.content]");
// 用session为参数定义消息对象
Message message = new MimeMessage(getSession());
// 加载发件人地址
message.setFrom(new InternetAddress(getMailUser().getAddress()));
if (mailBody.getSentDate() == null) {
message.setSentDate(new Date());
} else {
message.setSentDate(mailBody.getSentDate());
}
// 加载收件人地址
message.addRecipients(Message.RecipientType.TO, getAddress(mailAddress.getTo()));
if (mailAddress.isHasCC()) {
message.addRecipients(Message.RecipientType.CC, getAddress(mailAddress.getCc()));
}
// 加载标题
message.setSubject(encode(mailBody.getSubject()));
// 向multipart对象中添加邮件的各个部分内容,包括文本内容和附件
Multipart multipart = new MimeMultipart();
// 设置邮件的文本内容
MimeBodyPart contentPart = new MimeBodyPart();
if (mailBody.isMimeContent()) {
contentPart.setContent(mailBody.getContent(), "text/html;charset=" + getCharset());
} else {
contentPart.setText(mailBody.getContent(), getCharset());
}
multipart.addBodyPart(contentPart);
// 设置邮件附件
if (mailBody.hasAttach()) {
// 添加附件
for (MailAttach mailAttach : mailBody.getMailAttachs()) {
BodyPart attachBodyPart = new MimeBodyPart();
DataSource source = new FileDataSource(mailAttach.getFile());
// 添加附件的内容
attachBodyPart.setDataHandler(new DataHandler(source));
// 添加附件的标题这里很重要,通过下面的Base64编码的转换可以保证你的
// 中文附件标题名在发送时不会变成乱码
// String fileName = null;
// try {
//// fileName = "=?" + fileNameEncode + "?B?"
//// + Base64.encode(mailAttach.getFileName().getBytes(fileNameEncode))
//// + "?=";
// fileName = MimeUtility.encodeText(mailAttach.getFileName(), getCharset(), getEncoding());
// } catch (Exception e) {
// fileName = mailAttach.getFileName();
// logger.debug("编码附件标题[{}]出错:{}", fileName, e.getMessage());
// }
attachBodyPart.setFileName(encode(mailAttach.getFileName()));
multipart.addBodyPart(attachBodyPart);
}
}
// 设置Message-Id
message.setHeader("Message-Id", UUIDGenerator.generateUUID());
for (Entry property : mailBody.getProperties().entrySet()) {
if (property.getValue() != null) {
try {
message.setHeader(PROPERTY_PREFIX + property.getKey(),
MimeUtility.encodeText(property.getValue(), getCharset(), getEncoding()));
} catch (UnsupportedEncodingException e) {
logger.debug("参数[{}]编码出错:{}", property.getValue(), e.getMessage());
message.setHeader(PROPERTY_PREFIX + property.getKey(), property.getValue());
}
}
}
// 将multipart对象放到message中
message.setContent(multipart);
// 保存邮件
message.saveChanges();
return message;
}
private String encode(String str) {
try {
return MimeUtility.encodeText(str, getCharset(), getEncoding());
} catch (Exception e) {
logger.debug("编码字符串时[{}]出错:{}", str, e.getMessage());
}
return str;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy