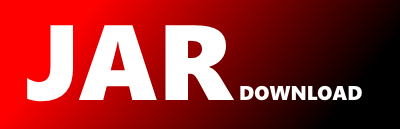
cn.foxtech.common.entity.entity.ConfigEntity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fox-edge-server-common-entity-service Show documentation
Show all versions of fox-edge-server-common-entity-service Show documentation
fox-edge-server-common-entity-service
The newest version!
/* ----------------------------------------------------------------------------
* Copyright (c) Guangzhou Fox-Tech Co., Ltd. 2020-2024. All rights reserved.
* --------------------------------------------------------------------------- */
package cn.foxtech.common.entity.entity;
import lombok.AccessLevel;
import lombok.Getter;
import lombok.Setter;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* 配置实体:各服务需要读取预置的全局配置参数
*/
@Getter(value = AccessLevel.PUBLIC)
@Setter(value = AccessLevel.PUBLIC)
public class ConfigEntity extends ConfigBase {
/**
* 数值信息
*/
private Map configValue = new HashMap<>();
/**
* 参数信息
*/
private Map configParam = new HashMap<>();
/**
* 业务Key
*
* @return 业务Key
*/
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy