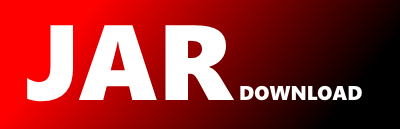
cn.foxtech.common.entity.utils.ExtendUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fox-edge-server-common-entity-service Show documentation
Show all versions of fox-edge-server-common-entity-service Show documentation
fox-edge-server-common-entity-service
The newest version!
/* ----------------------------------------------------------------------------
* Copyright (c) Guangzhou Fox-Tech Co., Ltd. 2020-2024. All rights reserved.
* --------------------------------------------------------------------------- */
package cn.foxtech.common.entity.utils;
import cn.foxtech.common.entity.entity.BaseEntity;
import cn.foxtech.common.utils.pair.Pair;
import java.util.*;
public class ExtendUtils {
/**
* 扩展信息
*
* @param mapList 基本数据
* @param extendEntityList 扩展数据
* @param key 基础数据和扩展数据,彼此关联的字段,相当于SQL语句中JOIN ON A.KEY=B.KEY
* @param property 需要从extendEntityList中添加的字段列表
*/
public static void extend(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy