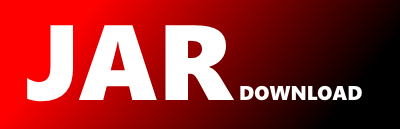
cn.foxtech.common.utils.http.HttpClientUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fox-edge-server-common-utils-hutool-http Show documentation
Show all versions of fox-edge-server-common-utils-hutool-http Show documentation
fox-edge-server-common-utils-hutool-http
The newest version!
/* ----------------------------------------------------------------------------
* Copyright (c) Guangzhou Fox-Tech Co., Ltd. 2020-2024. All rights reserved.
* --------------------------------------------------------------------------- */
package cn.foxtech.common.utils.http;
import cn.hutool.http.HttpRequest;
import cn.hutool.http.HttpResponse;
import java.util.HashMap;
import java.util.Map;
public class HttpClientUtils {
public static void main(String[] args) {
try {
HttpResponse result3 = HttpClientUtils.executeRestful("http://localhost:9000/manager/system/channel/entity", "get");
String json = "{\"name\":\"COM3\",\"send\":\"b0 01 00 fe fe\",\"timeout\":3000}";
HttpClientUtils util = new HttpClientUtils();
HttpResponse result = HttpClientUtils.executeRestful("http://localhost:9000/user/login?username=admin&password=12345678", "get");
// List cookies = result.getCookies();
// HttpCookie cookie = result.getCookie("token");
// String value = cookie.getValue();
// String body = result.body();
HttpResponse result1 = HttpClientUtils.executeRestful("http://localhost:9000/manager/system/channel/entity", "get");
String body1 = result1.body();
body1 = result1.body();
} catch (Exception e) {
e.printStackTrace();
}
}
public static HttpResponse executeRestful(String url, String method) {
Map headers = new HashMap<>();
return execute(url, method, headers, null);
}
public static HttpResponse executeRestful(String url, String method, Map headers) {
return execute(url, method, headers, null);
}
public static HttpResponse executeRestful(String url, String method, String json) {
Map headers = new HashMap<>();
headers.put("Content-Type", "application/json");
return execute(url, method, headers, json);
}
public static HttpResponse executeRestful(String url, String method, Map headers, String json) {
return execute(url, method, headers, json);
}
public static HttpResponse execute(String url, String method, Map headers, String body) {
HttpRequest httpRequest = null;
// 确定Method
if ("post".equalsIgnoreCase(method)) {
httpRequest = HttpRequest.post(url);
}
if ("put".equalsIgnoreCase(method)) {
httpRequest = HttpRequest.put(url);
}
if ("get".equalsIgnoreCase(method)) {
httpRequest = HttpRequest.get(url);
}
if ("delete".equalsIgnoreCase(method)) {
httpRequest = HttpRequest.delete(url);
}
if ("patch".equalsIgnoreCase(method)) {
httpRequest = HttpRequest.patch(url);
}
// 确定header
if (headers != null && !headers.isEmpty()) {
httpRequest = httpRequest.addHeaders(headers);
}
// 确定body
if (body != null && !body.isEmpty()) {
httpRequest = httpRequest.body(body);
}
// 发出请求
return httpRequest.execute();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy