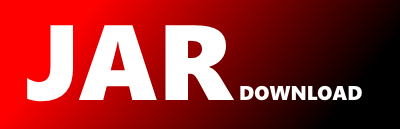
cn.fscode.commons.minio.manager.MinioManager Maven / Gradle / Ivy
package cn.fscode.commons.minio.manager;
import cn.fscode.commons.tool.core.StringUtils;
import cn.fscode.commons.tool.core.UrlUtils;
import cn.fscode.commons.tool.core.exception.Assert;
import cn.fscode.commons.tool.core.file.FileUtils;
import cn.fscode.commons.tool.core.file.MimeTypesUtils;
import cn.hutool.core.util.RandomUtil;
import cn.fscode.commons.minio.utils.MinioUtils;
import cn.fscode.commons.storage.api.config.MinioStorageProperties;
import cn.fscode.commons.storage.api.constants.UrlTypeEnum;
import cn.fscode.commons.storage.api.manager.StorageManager;
import io.minio.http.Method;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty;
import org.springframework.stereotype.Component;
import javax.annotation.PostConstruct;
import javax.annotation.Resource;
import java.io.ByteArrayInputStream;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.List;
import java.util.function.Consumer;
/**
* 为minio 设置 nginx 代理: http://docs.minio.org.cn/docs/master/setup-nginx-proxy-with-minio
* @author shenguangyang
*/
@Component
//@ConditionalOnStorageType(type = StorageTypesEnum.MINIO)
@ConditionalOnProperty(prefix = "storage", name = "type", havingValue = "minio")
public class MinioManager implements StorageManager {
private static final Logger log = LoggerFactory.getLogger(MinioManager.class);
@Resource
private MinioStorageProperties minioStorageProperties;
@PostConstruct
public void init() {
log.info("init {}", this.getClass().getName());
}
@Override
public void uploadText(String text, String objectName) throws Exception {
String bucketName = minioStorageProperties.getBucketName();
InputStream inputStream = new ByteArrayInputStream(text.getBytes(StandardCharsets.UTF_8));
MinioUtils.uploadFile(bucketName, inputStream, "text/plain", objectName);
}
@Override
public void uploadFile(InputStream inputStream, String contentType, String objectName) throws Exception {
String bucketName = minioStorageProperties.getBucketName();
MinioUtils.uploadFile(bucketName, inputStream, contentType, objectName);
}
@Override
public void uploadDir(String dirPath) {
List allFileList = FileUtils.getAllFile(dirPath, false, null);
dirPath = dirPath.replace("\\", "/");
if (!dirPath.endsWith("/")) {
dirPath = dirPath + "/";
}
for (String filePath : allFileList) {
String path = filePath.replace("\\", "/").replace(dirPath, "");
// 针对win路径进行处理
if (path.contains(":")) {
path = path.substring(path.lastIndexOf(":") + 1);
}
String objectName = path;
try (InputStream inputStream = Files.newInputStream(Paths.get(filePath))) {
uploadFile(inputStream, MimeTypesUtils.getInstance().getMimetype(FileUtils.getName(filePath)), objectName);
} catch (Exception e) {
log.error("error: ", e);
}
}
}
@Override
public String getObjectUrl(String objectName) {
String bucketName = minioStorageProperties.getBucketName();
String url = MinioUtils.getFileUrl(bucketName, objectName, Method.GET);
Assert.notEmpty(url, "获取url失败");
// if (UrlTypesEnum.INTERNAL.equals(urlTypes)) {
// String internal = storageProperties.getUrl().getInternal();
// return internal + url.replace(storageProperties.getMinio().getEndpoint(), "");
// } else {
// String external = storageProperties.getUrl().getExternal();
// return external + url.replace(storageProperties.getMinio().getEndpoint(), "");
// }
return url;
}
@Override
public String getPermanentObjectUrl(String objectName, UrlTypeEnum urlTypes) {
String bucketName = minioStorageProperties.getBucketName();
objectName = UrlUtils.removeStartsSlash(objectName);
if (UrlTypeEnum.INTERNAL.equals(urlTypes)) {
String internal = minioStorageProperties.getUrl().getIntranetEndpoint();
return StringUtils.join(internal, "/", bucketName, "/", objectName);
} else {
String external = minioStorageProperties.getUrl().getExternalEndpoint();
return StringUtils.join(external, "/", bucketName, "/", objectName);
}
}
@Override
public InputStream getFile(String objectName) {
String bucketName = minioStorageProperties.getBucketName();
return MinioUtils.getFile(bucketName, objectName);
}
@Override
public List listFilePath(String pathPrefix) {
String bucketName = minioStorageProperties.getBucketName();
return MinioUtils.listFilePath(bucketName, pathPrefix, null);
}
@Override
public List listFilePath(String pathPrefix, Consumer filePathCallback) {
String bucketName = minioStorageProperties.getBucketName();
return MinioUtils.listFilePath(bucketName, pathPrefix, filePathCallback);
}
@Override
public Boolean createBucket(Boolean randomSuffix) {
String bucketName = minioStorageProperties.getBucketName();
try {
if (randomSuffix != null && randomSuffix) {
MinioUtils.createBucket( bucketName + "-" + RandomUtil.randomString(8));
} else {
MinioUtils.createBucket(bucketName);
}
} catch (Exception e) {
log.error("createBucket::bucketName = [{}] message = {}",bucketName, e.getMessage());
return false;
}
return true;
}
@Override
public void deleteObjects(List objectNameList) throws Exception {
String bucketName = minioStorageProperties.getBucketName();
MinioUtils.deleteObjects(bucketName, objectNameList);
}
@Override
public void deleteObject(String objectName) throws Exception {
String bucketName = minioStorageProperties.getBucketName();
MinioUtils.deleteObject(bucketName, objectName);
}
@Override
public boolean checkFileIsExist(String objectName) {
String bucketName = minioStorageProperties.getBucketName();
return MinioUtils.checkFileIsExist(bucketName, objectName);
}
@Override
public boolean checkFolderIsExist(String folderName) {
String bucketName = minioStorageProperties.getBucketName();
return MinioUtils.checkFolderIsExist(bucketName, folderName);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy