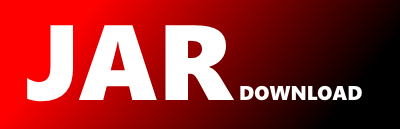
cn.geektool.kafka.consumer.generator.KafkaConsumerGenerator Maven / Gradle / Ivy
The newest version!
package cn.geektool.kafka.consumer.generator;
import cn.geektool.core.util.StrUtil;
import cn.geektool.kafka.admin.event.KafkaEvent;
import cn.geektool.kafka.consumer.bean.ConsumerBean;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import org.apache.kafka.clients.consumer.KafkaConsumer;
import org.apache.kafka.common.PartitionInfo;
import org.apache.kafka.common.TopicPartition;
import cn.geektool.core.util.CollectionUtil;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* 消费者生成器
*
* @author jiangdi
* @since 0.0.1
*/
public final class KafkaConsumerGenerator {
/**
* 生成消费者参数
*
* @param consumerBean 消费者的bean
* @return 生成的参数
*/
public static List generatorParam(ConsumerBean consumerBean) {
List result = new ArrayList();
Map map = ((JSONObject) JSON.toJSON(consumerBean)).getInnerMap();
String[] topics = StrUtil.split(consumerBean.getTopics(), ",");
if (CollectionUtil.isNotEmpty(topics)) {
for (String topic : topics) {
initTopic(map, topic, consumerBean, result);
}
}
return result;
}
/**
* 根据主题初始化
*
* @param props 初始化的参数
* @param topic 初始化的主题
* @param consumerBean 初始化
*/
private static void initTopic(Map props, String topic, ConsumerBean consumerBean, List result) {
Map cloneProps = new HashMap<>(props);
KafkaConsumer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy