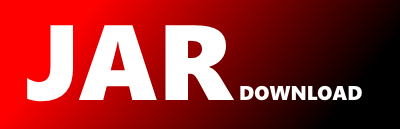
cn.godmao.aspectj.AspectExpress Maven / Gradle / Ivy
package cn.godmao.aspectj;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.reflect.MethodSignature;
import org.springframework.core.StandardReflectionParameterNameDiscoverer;
import org.springframework.expression.EvaluationContext;
import org.springframework.expression.Expression;
import org.springframework.expression.TypedValue;
import org.springframework.expression.spel.standard.SpelExpressionParser;
import org.springframework.expression.spel.support.StandardEvaluationContext;
import java.lang.reflect.Method;
public class AspectExpress {
public static String[] setKeys(ProceedingJoinPoint point, String[] keys) {
for (int i = 0; i < keys.length; i++) {
String spEl = String.valueOf(getSpEl(point, keys[i]));
if (spEl != null && !spEl.isEmpty()) {
keys[i] = spEl;
}
}
return keys;
}
public static T getSpEl(JoinPoint joinPoint, String el, Class clazz) {
return getSpEl(joinPoint, (MethodSignature) joinPoint.getSignature(), el, clazz);
}
public static T getSpEl(JoinPoint joinPoint, MethodSignature methodSignature, String el, Class clazz) {
EvaluationContext context = getContext(joinPoint.getArgs(), methodSignature.getMethod(), joinPoint.getTarget());
return getValue(context, el, clazz);
}
/**
* 获取spel 定义的参数值
*
* @param context 参数容器
* @param key key
* @param clazz 需要返回的类型
* @param 返回泛型
* @return 参数值
*/
private static T getValue(EvaluationContext context, String key, Class clazz) {
SpelExpressionParser spelExpressionParser = new SpelExpressionParser();
Expression expression = spelExpressionParser.parseExpression(key);
return expression.getValue(context, clazz);
}
/**
* 获取参数容器
*
* @param arguments 方法的参数列表
* @param signatureMethod 被执行的方法体
* @return 装载参数的容器
*/
private static EvaluationContext getContext(Object[] arguments, Method signatureMethod, Object rootObject) {
String[] parameterNames = new StandardReflectionParameterNameDiscoverer().getParameterNames(signatureMethod);
// String[] parameterNames = new LocalVariableTableParameterNameDiscoverer().getParameterNames(signatureMethod);
if (parameterNames == null) {
throw new RuntimeException("参数列表不能为null");
}
EvaluationContext context = new StandardEvaluationContext(null == rootObject ? TypedValue.NULL : rootObject);
for (int i = 0; i < arguments.length; i++) {
context.setVariable(parameterNames[i], arguments[i]);
}
return context;
}
// -----------------------------------------
public static Object getSpEl(JoinPoint joinPoint, String el) {
return getSpEl(joinPoint, (MethodSignature) joinPoint.getSignature(), el);
}
public static Object getSpEl(JoinPoint joinPoint, MethodSignature methodSignature, String el) {
EvaluationContext context = getContext(joinPoint.getArgs(), methodSignature.getMethod(), joinPoint.getTarget());
return getValue(context, el);
}
private static Object getValue(EvaluationContext context, String key) {
SpelExpressionParser spelExpressionParser = new SpelExpressionParser();
Expression expression = spelExpressionParser.parseExpression(key);
return expression.getValue(context);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy