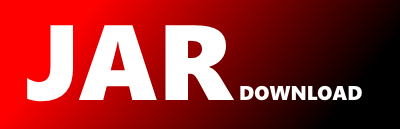
cn.handyplus.lib.core.CollUtil Maven / Gradle / Ivy
The newest version!
package cn.handyplus.lib.core;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Random;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* 集合工具
*
* @author handy
* @since 1.1.8
*/
public class CollUtil {
private CollUtil() {
}
/**
* 集合转,分隔的字符串
*
* @param list 集合
* @param 字符串
* @return 分隔的字符串
*/
public static String listToStr(List list) {
return listToStr(list, ",");
}
/**
* 集合转 delimiter 分隔的字符串
*
* @param list 集合
* @param 字符串
* @param delimiter 要在每个元素之间使用的分隔符
* @return 分隔的字符串
* @since 3.6.8
*/
public static String listToStr(List list, CharSequence delimiter) {
if (isEmpty(list)) {
return "";
}
return list.stream().map(String::valueOf).collect(Collectors.joining(delimiter));
}
/**
* 集合是否为空
*
* @param collection 集合
* @return true 是
*/
public static boolean isEmpty(Collection> collection) {
return collection == null || collection.isEmpty();
}
/**
* 集合是否不为空
*
* @param collection 集合
* @return true 是
*/
public static boolean isNotEmpty(Collection> collection) {
return !isEmpty(collection);
}
/**
* 判断list是否相等
*
* @param list list
* @param list1 list1
* @return true/等于
*/
public static boolean equals(List list, List list1) {
if (list == list1) {
return true;
}
if (list == null) {
return false;
}
return list.equals(list1);
}
/**
* 对list进行分组
*
* @param list list
* @param toIndex 分组数
* @param t
* @return List
* @since 2.3.1
*/
public static List> partition(List list, int toIndex) {
List> listGroup = new ArrayList<>();
int listSize = list.size();
for (int i = 0; i < list.size(); i += toIndex) {
if (i + toIndex > listSize) {
toIndex = listSize - i;
}
List newList = list.subList(i, i + toIndex);
listGroup.add(newList);
}
return listGroup;
}
/**
* 获取List中第一个包含的值
*
* @param list 集合
* @param str 包含的值
* @return List中的值
* @since 3.1.2
*/
public static String getContainsStr(List list, String str) {
if (CollUtil.isEmpty(list) || StrUtil.isEmpty(str)) {
return null;
}
for (String string : list) {
if (string.contains(str)) {
return string;
}
}
return null;
}
/**
* 获取List中对应的的值
*
* @param list 集合
* @param str 包含的值
* @return List中对应的的值
* @since 3.1.2
*/
public static String getStr(List list, String str) {
if (CollUtil.isEmpty(list) || StrUtil.isEmpty(str)) {
return null;
}
int i = list.indexOf(str);
return i != -1 ? list.get(i) : null;
}
/**
* 分割list集合
*
* @param list 集合数据
* @param splitSize 几个分割一组
* @param 类型
* @return 集合分割后的集合
* @since 3.4.7
*/
public static List> splitList(List list, int splitSize) {
//判断集合是否为空
if (CollUtil.isEmpty(list)) {
return Collections.emptyList();
}
//计算分割后的大小
int maxSize = (list.size() + splitSize - 1) / splitSize;
//开始分割
return Stream.iterate(0, n -> n + 1)
.limit(maxSize)
.parallel()
.map(a -> list.parallelStream().skip((long) a * splitSize).limit(splitSize).collect(Collectors.toList()))
.filter(b -> !b.isEmpty())
.collect(Collectors.toList());
}
/**
* 获取随机一个元素
*
* @param list 集合
* @param 类型
* @return 随机一个元素
* @since 3.10.2
*/
public static T randomElement(List list) {
if (CollUtil.isEmpty(list)) {
return null;
}
return list.get(new Random().nextInt(list.size()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy