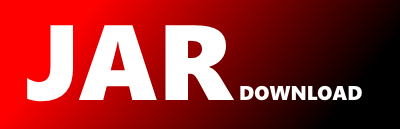
cn.handyplus.lib.core.DateUtil Maven / Gradle / Ivy
The newest version!
package cn.handyplus.lib.core;
import org.bukkit.Bukkit;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.time.LocalDateTime;
import java.time.ZoneId;
import java.time.ZoneOffset;
import java.time.temporal.ChronoUnit;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.Locale;
import java.util.logging.Level;
/**
* 时间工具类
*
* @author handy
* @since 1.5.8
*/
public class DateUtil {
private DateUtil() {
}
/**
* 简化格式
*/
public final static String YYYY = "yyyy-MM-dd";
/**
* 完整格式
*/
public final static String YYYY_HH = "yyyy-MM-dd HH:mm:ss";
/**
* 字符串转date
*
* @param str 字符串
* @param format 格式
* @return date
*/
public static Date parse(String str, String format) {
SimpleDateFormat sdf = new SimpleDateFormat(format);
try {
return sdf.parse(str);
} catch (ParseException e) {
Bukkit.getLogger().log(Level.SEVERE, "parse 发生异常", e);
}
return null;
}
/**
* date转字符串
*
* @param date 时间
* @param format 格式
* @return 字符串
*/
public static String format(Date date, String format) {
SimpleDateFormat sdf = new SimpleDateFormat(format);
return sdf.format(date);
}
/**
* 通过时间秒毫秒数判断两个时间的间隔
*
* @param dateTime 时间
* @return 时间间隔
*/
public static int getDifferDay(Long dateTime) {
return (int) ((System.currentTimeMillis() - dateTime) / (1000 * 3600 * 24));
}
/**
* 时间增加
*
* @param day 增加天数
* @return 时间
*/
public static Date getDate(Integer day) {
Date date = new Date();
Calendar calendar = new GregorianCalendar();
calendar.setTime(date);
//把日期往后增加一天.整数往后推,负数往前移动
calendar.add(Calendar.DATE, day);
return calendar.getTime();
}
/**
* 判断传入的时间是否大于2120-01-01 00:00:00
*
* @param date 日期
* @return true 是
*/
public static boolean isPerpetual(Date date) {
return date.getTime() > 4733481600000L;
}
/**
* 获取今日日期
*
* @return 今日开始日期
*/
public static Date getToday() {
Date date = new Date();
SimpleDateFormat sdf = new SimpleDateFormat(YYYY);
try {
date = sdf.parse(sdf.format(date));
} catch (ParseException e) {
Bukkit.getLogger().log(Level.SEVERE, "getToday 发生异常", e);
}
return date;
}
/**
* 获取今日结束
*
* @return 今日结束时间
* @since 3.5.4
*/
public static Date getTodayEnd() {
return DateUtil.parse(DateUtil.format(getToday(), YYYY) + " 23:59:59", YYYY_HH);
}
/**
* 获取周一日期
*
* @return 周一日期
* @since 2.3.4
*/
public static Date getMonday() {
Calendar calendar = Calendar.getInstance(Locale.CHINA);
calendar.setFirstDayOfWeek(Calendar.MONDAY);
calendar.setTimeInMillis(getToday().getTime());
calendar.set(Calendar.DAY_OF_WEEK, Calendar.MONDAY);
return calendar.getTime();
}
/**
* 获取周日日期
*
* @return 周日日期
* @since 2.3.4
*/
public static Date getSunday() {
Calendar calendar = Calendar.getInstance(Locale.CHINA);
calendar.setFirstDayOfWeek(Calendar.MONDAY);
calendar.setTimeInMillis(getToday().getTime());
calendar.set(Calendar.DAY_OF_WEEK, Calendar.SUNDAY);
return DateUtil.parse(DateUtil.format(calendar.getTime(), YYYY) + " 23:59:59", YYYY_HH);
}
/**
* 获得指定日期是星期几
*
* @param date 日期
* @return 对应的星期
* @since 2.2.4
*/
public static Integer dayOfWeekEnum(Date date) {
Integer[] weekDays = {7, 1, 2, 3, 4, 5, 6};
Calendar calendar = Calendar.getInstance();
return weekDays[calendar.get(Calendar.DAY_OF_WEEK) - 1];
}
/**
* LocalDateTime 转date
*
* @param localDateTime localDateTime
* @return Date
* @since 2.6.2
*/
public static Date toDate(LocalDateTime localDateTime) {
return Date.from(localDateTime.atZone(ZoneId.systemDefault()).toInstant());
}
/**
* date 转 LocalDateTime
*
* @param date date
* @return LocalDateTime
* @since 2.6.2
*/
public static LocalDateTime toLocalDateTime(Date date) {
return LocalDateTime.ofInstant(date.toInstant(), ZoneId.systemDefault());
}
/**
* LocalDateTime 转毫秒时间戳
*
* @param localDateTime localDateTime
* @return 毫秒时间戳
* @since 2.6.2
*/
public static long toEpochSecond(LocalDateTime localDateTime) {
return localDateTime.toInstant(ZoneOffset.of("+8")).toEpochMilli();
}
/**
* 计算相差小时
*
* @param dateOne 第一个时间
* @param dateTwo 第二个时间
* @return 相差小时
* @since 2.6.2
*/
public static long between(Date dateOne, Date dateTwo) {
return between(dateOne, dateTwo, ChronoUnit.HOURS);
}
/**
* 计算时间相差
*
* @param dateOne 第一个时间
* @param dateTwo 第二个时间
* @param unit unit
* @return 相差多久
* @since 2.6.2
*/
public static long between(Date dateOne, Date dateTwo, ChronoUnit unit) {
return unit.between(toLocalDateTime(dateOne), toLocalDateTime(dateTwo));
}
/**
* 根据日期获取本周几
*
* @param date 时间
* @param week 周几1-7
* @return 对应周日期
* @since 2.8.5
*/
public static Date getWeek(Date date, int week) {
week = week + 1;
if (week > 7) {
week = 1;
}
Calendar calendar = Calendar.getInstance(Locale.CHINA);
calendar.setFirstDayOfWeek(Calendar.MONDAY);
calendar.setTimeInMillis(date.getTime());
calendar.set(Calendar.DAY_OF_WEEK, week);
return calendar.getTime();
}
/**
* 时间增加
* 整数往后推,负数往前移动
*
* @param date 日期
* @param day 增加天数
* @return 时间
* @since 2.8.5
*/
public static Date addDate(Date date, Integer day) {
Calendar calendar = new GregorianCalendar();
calendar.setTime(date);
calendar.add(Calendar.DATE, day);
return calendar.getTime();
}
/**
* 获取月初
*
* @return 月初
* @since 3.5.4
*/
public static Date getFirstDayOfMonth() {
return toDate(LocalDateTime.now().withDayOfMonth(1).truncatedTo(ChronoUnit.DAYS));
}
/**
* 获取月初
*
* @param date 日期
* @return 月初
* @since 2.8.5
*/
public static Date getFirstDayOfMonth(Date date) {
return toDate(toLocalDateTime(date).withDayOfMonth(1).truncatedTo(ChronoUnit.DAYS));
}
/**
* 获取月末
*
* @return 月末
* @since 2.8.5
*/
public static Date getLastDayOfMonth() {
return toDate(LocalDateTime.now().plusMonths(1L).withDayOfMonth(1).truncatedTo(ChronoUnit.DAYS).plus(-1L, ChronoUnit.MILLIS));
}
/**
* 获取月末
*
* @param date 日期
* @return 月末
* @since 2.8.5
*/
public static Date getLastDayOfMonth(Date date) {
return toDate(toLocalDateTime(date).plusMonths(1L).withDayOfMonth(1).truncatedTo(ChronoUnit.DAYS).plus(-1L, ChronoUnit.MILLIS));
}
/**
* 根据日期获取本月几号
*
* @param date 时间
* @param month 几号
* @return 对应月日期
* @since 2.8.5
*/
public static Date getMonth(Date date, int month) {
Calendar calendar = Calendar.getInstance(Locale.CHINA);
calendar.setFirstDayOfWeek(Calendar.MONDAY);
calendar.setTimeInMillis(date.getTime());
calendar.set(Calendar.DAY_OF_MONTH, month);
return calendar.getTime();
}
/**
* 调整日期和时间
*
* @param date 时间
* @param field 类型 参考Calendar
* @param offset 偏移量,正数为向后偏移,负数为向前偏移
* @return 新时间对象
* @since 2.8.6
*/
public static Date offset(Date date, int field, int offset) {
Calendar cal = Calendar.getInstance();
cal.setTime(date);
cal.add(field, offset);
return cal.getTime();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy