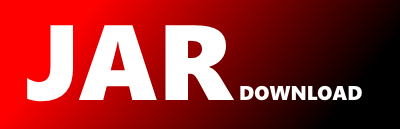
cn.handyplus.lib.core.LockUtil Maven / Gradle / Ivy
The newest version!
package cn.handyplus.lib.core;
import java.util.Map;
import java.util.UUID;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.concurrent.locks.ReentrantLock;
/**
* 资源锁
*
* @author handy
* @since 3.2.5
*/
public class LockUtil {
private LockUtil() {
}
/**
* Atomic锁
*
* @since 3.2.5
*/
private static final ConcurrentHashMap ATOMIC_LOCK_MAP = new ConcurrentHashMap<>();
/**
* time 锁
*/
private static final ConcurrentHashMap TIME_LOCK_MAP = new ConcurrentHashMap<>();
/**
* ReentrantLock 锁
*/
private static final Map REENTRANT_LOCK_MAP = new ConcurrentHashMap<>();
/**
* 锁时间
*/
private static final Integer LOCK_TIME = 350;
/**
* 加锁
*
* @param key 锁key
* @return AtomicInteger
*/
public static AtomicInteger lock(String key) {
// 当实体ID锁资源为空,初始化锁
if (ATOMIC_LOCK_MAP.get(key) == null) {
// 初始化一个竞争数为0的原子资源
ATOMIC_LOCK_MAP.putIfAbsent(key, new AtomicInteger(0));
}
// 线程得到该资源,原子性+1
ATOMIC_LOCK_MAP.get(key).incrementAndGet();
// 返回该ID资源锁
return ATOMIC_LOCK_MAP.get(key);
}
/**
* 释放锁
*
* @param key key
*/
public static void unLock(String key) {
ATOMIC_LOCK_MAP.remove(key);
}
/**
* 时间锁
*
* @param key 锁key
* @return true 可执行
* @since 3.3.9
*/
public static boolean timeLock(UUID key) {
// 当实体ID锁资源为空,初始化锁
Long time = TIME_LOCK_MAP.get(key);
long currentTimeMillis = System.currentTimeMillis();
if (time == null) {
// 初始化一个竞争数为0的原子资源
TIME_LOCK_MAP.putIfAbsent(key, System.currentTimeMillis());
return true;
}
// 线程得到该资源,判断是否可执行
if (currentTimeMillis - time < LOCK_TIME) {
return false;
}
// 时间重新赋值
TIME_LOCK_MAP.put(key, currentTimeMillis);
return true;
}
/**
* 释放时间锁
*
* @param key 锁key
* @since 3.3.9
*/
public static void unTimeLock(UUID key) {
TIME_LOCK_MAP.remove(key);
}
/**
* 加锁
*
* @param key 锁key
* @since 3.7.7
*/
public static void reentrantLock(String key) {
REENTRANT_LOCK_MAP.computeIfAbsent(key, k -> new ReentrantLock(true)).lock();
}
/**
* 释放锁
*
* @param key 锁key
* @since 3.7.7
*/
public static void unReentrantLock(String key) {
// 先从容器中拿锁,确保锁的存在
ReentrantLock lock = REENTRANT_LOCK_MAP.get(key);
// 释放锁
lock.unlock();
// 检查是否需要淘汰锁
if (lock.getQueueLength() < 1) {
REENTRANT_LOCK_MAP.remove(key);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy