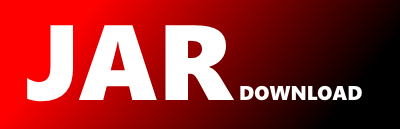
cn.handyplus.lib.core.NumberUtil Maven / Gradle / Ivy
package cn.handyplus.lib.core;
import cn.handyplus.lib.constants.BaseConstants;
import java.math.BigDecimal;
import java.util.Optional;
import java.util.regex.Matcher;
/**
* 数字工具类
*
* @author handy
* @since 3.7.5
*/
public class NumberUtil {
private NumberUtil() {
}
/**
* 利用正则表达式判断字符串是否是数字
*
* @param str 字符串
* @return 数字或null
*/
public static Integer isNumericToInt(String str) {
return isNumericToInt(str, null);
}
/**
* 利用正则表达式判断字符串是否是数字
*
* @param str 字符串
* @param def 默认值
* @return 数字
* @since 3.6.8
*/
public static Integer isNumericToInt(String str, Integer def) {
return isNumericToBigDecimal(str).map(BigDecimal::intValue).orElse(def);
}
/**
* 利用正则表达式判断字符串是否是数字
*
* @param str 字符串
* @return true是数字
* @since 3.5.2
*/
public static Double isNumericToDouble(String str) {
return isNumericToDouble(str, null);
}
/**
* 利用正则表达式判断字符串是否是数字
*
* @param str 字符串
* @param def 默认值
* @return true是数字
* @since 3.7.1
*/
public static Double isNumericToDouble(String str, Double def) {
return isNumericToBigDecimal(str).map(BigDecimal::doubleValue).orElse(def);
}
/**
* 利用正则表达式判断字符串是否是数字
*
* @param str 字符串
* @return true是数字
*/
public static Long isNumericToLong(String str) {
return isNumericToLong(str, null);
}
/**
* 利用正则表达式判断字符串是否是数字
*
* @param str 字符串
* @param def 默认
* @return true是数字
* @since 3.7.0
*/
public static Long isNumericToLong(String str, Long def) {
return isNumericToBigDecimal(str).map(BigDecimal::longValue).orElse(def);
}
/**
* 利用正则表达式判断字符串是否是数字
*
* @param str 字符串
* @return true是数字
* @since 3.7.5
*/
public static Optional isNumericToBigDecimal(String str) {
return isNumericToBigDecimal(str, null);
}
/**
* 利用正则表达式判断字符串是否是数字
*
* @param str 字符串
* @param def 默认
* @return true是数字
* @since 3.7.5
*/
public static Optional isNumericToBigDecimal(String str, BigDecimal def) {
try {
Matcher isNum = BaseConstants.BIG_DECIMAL_NUMERIC.matcher(str);
if (isNum.matches()) {
return Optional.of(new BigDecimal(str));
}
} catch (NumberFormatException e) {
return Optional.ofNullable(def);
}
return Optional.ofNullable(def);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy