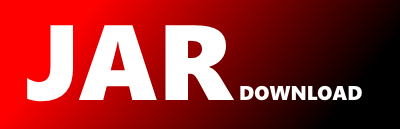
cn.handyplus.lib.core.SecureUtil Maven / Gradle / Ivy
The newest version!
package cn.handyplus.lib.core;
import lombok.SneakyThrows;
import org.bukkit.Bukkit;
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.Base64;
import java.util.Optional;
import java.util.logging.Level;
/**
* 安全处理类
*
* @author handy
* @since 3.4.7
*/
public class SecureUtil {
private SecureUtil() {
}
/**
* md5加密
*
* @param str 入参
* @return 加密字符
*/
public static Optional md5(String str) {
try {
//1.获取算法MD5实例
MessageDigest md = MessageDigest.getInstance("MD5");
//2.MD5加密
byte[] buff = md.digest(str.getBytes());
//3.将128位的二进制编码转为32位的16进制编码
return Optional.of(toHex(buff));
} catch (NoSuchAlgorithmException e) {
Bukkit.getLogger().log(Level.SEVERE, "md5 发生异常", e);
}
return Optional.empty();
}
/**
* 将128位的二进制序列转为32位的16进制编码
*/
private static String toHex(byte[] bytes) {
StringBuilder md5str = new StringBuilder();
for (int aByte : bytes) {
int temp = aByte;
// 0x8* 在经过toHexString转化时,会被转为 ffffff8* ,需要+256保证其正值
if (temp < 0) {
temp += 256;
}
// 0x05 转化会变成 5,缺少一位0
if (temp < 16) {
md5str.append("0");
}
md5str.append(Integer.toHexString(temp));
}
return md5str.toString();
}
private static final String AES_SECRET_KEY = "HANDY_LIB_SECRET";
/**
* 使用AES算法对输入字符串进行加密
*
* @param input 要加密的字符串
* @return 加密后的Base64编码字符串
* @since 3.9.4
*/
@SneakyThrows
public static String encrypt(String input) {
SecretKeySpec key = new SecretKeySpec(AES_SECRET_KEY.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] encryptedBytes = cipher.doFinal(input.getBytes(StandardCharsets.UTF_8));
return Base64.getEncoder().encodeToString(encryptedBytes);
}
/**
* 使用AES算法对输入字符串进行解密
*
* @param input 要解密的Base64编码字符串
* @return 解密后的字符串
* @since 3.9.4
*/
@SneakyThrows
public static String decrypt(String input) {
SecretKeySpec key = new SecretKeySpec(AES_SECRET_KEY.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding");
cipher.init(Cipher.DECRYPT_MODE, key);
byte[] decryptedBytes = cipher.doFinal(Base64.getDecoder().decode(input));
return new String(decryptedBytes, StandardCharsets.UTF_8);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy