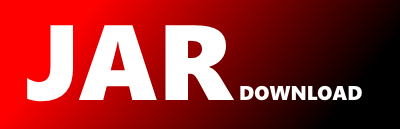
cn.handyplus.lib.core.StrUtil Maven / Gradle / Ivy
The newest version!
package cn.handyplus.lib.core;
import cn.handyplus.lib.constants.BaseConstants;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.regex.Matcher;
import java.util.stream.Collectors;
/**
* 字符串工具
*
* @author handy
* @since 1.1.8
*/
public class StrUtil {
private StrUtil() {
}
/**
* 是否为空
*
* @param str 字符串
* @return true/是
*/
public static boolean isEmpty(CharSequence str) {
return str == null || str.length() == 0;
}
/**
* 是否不为空
*
* @param str 字符串
* @return true/是
*/
public static boolean isNotEmpty(CharSequence str) {
return !isEmpty(str);
}
/**
* 转换小写
*
* @param str 字符串
* @return 小写字符串
*/
public static String toLowerCase(String str) {
return str != null ? str.toLowerCase() : null;
}
/**
* 将#替换成空格
*
* @param str 字符串
* @return 替换后的字符串
*/
public static String replaceSpace(String str) {
if (isEmpty(str)) {
return str;
}
return str.replace("#", " ");
}
/**
* 字符串转集合
*
* @param str 字符串
* @return 集合
*/
public static List strToStrList(String str) {
return strToStrList(str, ",");
}
/**
* 字符串转集合
*
* @param str 字符串
* @param split 分割符
* @return 集合
* @since 3.7.9
*/
public static List strToStrList(String str, String split) {
List list = new ArrayList<>();
if (isEmpty(str)) {
return list;
}
return Arrays.stream(str.split(split)).map(String::trim).collect(Collectors.toList());
}
/**
* 字符串转集合
*
* @param str 字符串
* @return 集合
*/
public static List strToLongList(String str) {
List list = new ArrayList<>();
if (isEmpty(str)) {
return list;
}
return Arrays.stream(str.split(",")).map(s -> Long.parseLong(s.trim())).collect(Collectors.toList());
}
/**
* 字符串转集合
*
* @param str 字符串
* @return 集合
*/
public static List strToIntList(String str) {
List list = new ArrayList<>();
if (isEmpty(str)) {
return list;
}
return Arrays.stream(str.split(",")).map(s -> Integer.valueOf(s.trim())).collect(Collectors.toList());
}
/**
* 下划线转驼峰
*
* @param str 字符
* @return 结果
* @since 1.4.8
*/
public static String lineToHump(String str) {
str = str.toLowerCase();
Matcher matcher = BaseConstants.LINE_PATTERN.matcher(str);
StringBuffer sb = new StringBuffer();
while (matcher.find()) {
matcher.appendReplacement(sb, matcher.group(1).toUpperCase());
}
matcher.appendTail(sb);
return sb.toString();
}
/**
* 驼峰转下划线
*
* @param str 字符
* @return 结果
* @since 1.4.8
*/
public static String humpToLine(String str) {
Matcher matcher = BaseConstants.HUMP_PATTERN.matcher(str);
StringBuffer sb = new StringBuffer();
while (matcher.find()) {
matcher.appendReplacement(sb, "_" + matcher.group(0).toLowerCase());
}
matcher.appendTail(sb);
return sb.toString();
}
/**
* 去除字符串中所有的空白符
*
* @param str 字符串
* @return 新字符串
* @since 3.1.0
*/
public static String deleteWhitespace(String str) {
if (isEmpty(str)) {
return str;
}
int sz = str.length();
char[] chs = new char[sz];
int count = 0;
for (int i = 0; i < sz; ++i) {
if (!Character.isWhitespace(str.charAt(i))) {
chs[count++] = str.charAt(i);
}
}
if (count == sz) {
return str;
} else {
return new String(chs, 0, count);
}
}
/**
* 替换表达式
*
* @param str 字符串
* @param format 格式
* @param value 替换的值
* @return 替换后的字符串
* @since 3.7.1
*/
public static String replace(String str, String format, String value) {
if (isEmpty(str) || isEmpty(format)) {
return str;
}
return str.replace("${" + format + "}", value);
}
/**
* 比较两个字符串是否相等,规则如下
*
* @param str1 要比较的字符串1
* @param str2 要比较的字符串2
* @return 如果两个字符串相同,或者都是{@code null},则返回{@code true}
* @since 3.7.4
*/
public static boolean equals(CharSequence str1, CharSequence str2) {
if (null == str1) {
// 只有两个都为null才判断相等
return str2 == null;
}
if (null == str2) {
// 字符串2空,字符串1非空,直接false
return false;
}
return str1.toString().contentEquals(str2);
}
/**
* 替换指定字符串中最后出现的与给定正则表达式匹配的子字符串。
*
* @param text 要搜索和替换的字符串
* @param regex 要搜索的正则表达式
* @param replacement 替换的字符串
* @return 替换最后一次出现的与正则表达式匹配的子字符串后的结果字符串
* @since 3.8.6
*/
public static String replaceLast(String text, String regex, String replacement) {
int lastIndex = text.lastIndexOf(regex);
if (lastIndex != -1) {
return text.substring(0, lastIndex) + replacement + text.substring(lastIndex + regex.length());
}
return text;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy