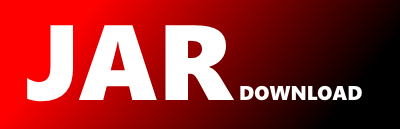
cn.handyplus.lib.db.SqlManagerUtil Maven / Gradle / Ivy
The newest version!
package cn.handyplus.lib.db;
import cn.handyplus.lib.InitApi;
import cn.handyplus.lib.constants.BaseConstants;
import cn.handyplus.lib.core.StrUtil;
import cn.handyplus.lib.db.enums.DbTypeEnum;
import cn.handyplus.lib.util.HandyConfigUtil;
import cn.handyplus.lib.util.MessageUtil;
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
import org.bukkit.Bukkit;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.logging.Level;
/**
* 连接池管理
*
* @author handy
*/
public class SqlManagerUtil {
private static final SqlManagerUtil INSTANCE = new SqlManagerUtil();
/**
* 数据源
*/
private HikariDataSource ds;
private SqlManagerUtil() {
}
/**
* 获取唯一实例
*
* @return SqlManagerUtil
*/
public static SqlManagerUtil getInstance() {
return INSTANCE;
}
/**
* 加载storage文件,并初始化连接池
*/
public static void enableSql() {
enableSql(null);
}
/**
* 加载storage文件,并初始化连接池
*
* @param storageMethod 连接方式
* @since 2.9.2
*/
public static void enableSql(String storageMethod) {
BaseConstants.STORAGE_CONFIG = HandyConfigUtil.load("storage.yml");
// 关闭当前数据源
SqlManagerUtil.getInstance().close();
// 初始化连接池
SqlManagerUtil.getInstance().enableTable(storageMethod);
}
/**
* 初始化连接
*
* @param storageMethod 连接方式
*/
public void enableTable(String storageMethod) {
// 没有指定链接获取默认方式
if (StrUtil.isEmpty(storageMethod)) {
storageMethod = this.getStorageMethod();
}
HikariConfig hikariConfig = new HikariConfig();
hikariConfig.setPoolName((InitApi.PLUGIN != null ? InitApi.PLUGIN.getName() : "HandyLib") + "HikariPool");
if (DbTypeEnum.MySQL.getType().equalsIgnoreCase(storageMethod)) {
String host = BaseConstants.STORAGE_CONFIG.getString("MySQL.Host");
String database = BaseConstants.STORAGE_CONFIG.getString("MySQL.Database");
int port = BaseConstants.STORAGE_CONFIG.getInt("MySQL.Port");
String useSsl = BaseConstants.STORAGE_CONFIG.getString("MySQL.UseSSL");
String param = BaseConstants.STORAGE_CONFIG.getString("MySQL.param", "");
String jdbcUrl = "jdbc:mysql://" + host + ":" + port + "/" + database + "?useSSL=" + useSsl + "&useUnicode=true&characterEncoding=UTF-8&rewriteBatchedStatements=true" + param;
hikariConfig.setJdbcUrl(jdbcUrl);
hikariConfig.setUsername(BaseConstants.STORAGE_CONFIG.getString("MySQL.User"));
hikariConfig.setPassword(BaseConstants.STORAGE_CONFIG.getString("MySQL.Password"));
} else {
String jdbcUrl;
if (InitApi.PLUGIN != null) {
jdbcUrl = "jdbc:sqlite:" + InitApi.PLUGIN.getDataFolder().getAbsolutePath() + "/" + InitApi.PLUGIN.getName() + ".db";
} else {
jdbcUrl = "jdbc:sqlite:HandyLib.db";
}
hikariConfig.setDriverClassName("org.sqlite.JDBC");
hikariConfig.setConnectionTestQuery("SELECT 1");
hikariConfig.setJdbcUrl(jdbcUrl);
}
ds = new HikariDataSource(hikariConfig);
}
/**
* 获取连接
*
* @param storageMethod 连接方式
* @return conn
*/
public Connection getConnection(String storageMethod) {
// 没有指定链接获取默认方式
if (StrUtil.isEmpty(storageMethod)) {
storageMethod = this.getStorageMethod();
}
// 如果链接被关闭了,就重新打开
if (ds == null || ds.isClosed()) {
MessageUtil.sendConsoleDebugMessage(" HikariDataSource 链接异常关闭,重新打开");
enableTable(storageMethod);
}
try {
return ds.getConnection();
} catch (SQLException e) {
Bukkit.getLogger().log(Level.SEVERE, "getConnection 发生异常", e);
throw new RuntimeException(e);
}
}
/**
* 安静设置自动提交
*
* @param conn 链接
* @param autoCommit 自动提交
* @since 3.10.8
*/
public void quietSetAutoCommit(Connection conn, Boolean autoCommit) {
if (null != conn && null != autoCommit) {
try {
conn.setAutoCommit(autoCommit);
} catch (Exception e) {
Bukkit.getLogger().log(Level.SEVERE, "quietSetAutoCommit 发生异常", e);
}
}
}
/**
* 归还数据连接
*
* @param ps PreparedStatement
* @param conn Connection
* @param rst ResultSet
*/
public void closeSql(Connection conn, PreparedStatement ps, ResultSet rst) {
try {
if (rst != null) {
rst.close();
}
if (ps != null) {
ps.close();
}
if (conn != null && conn.getAutoCommit()) {
conn.close();
}
} catch (SQLException e) {
Bukkit.getLogger().log(Level.SEVERE, "closeSql 发生异常", e);
}
}
/**
* 关闭数据源
*/
public void close() {
if (ds != null) {
ds.close();
}
}
/**
* 判断当前是什么存储类型
*
* @return 存储类型
* @since 1.1.9
*/
public String getStorageMethod() {
if (BaseConstants.STORAGE_CONFIG == null) {
return DbTypeEnum.SQLite.getType();
}
String storageMethod = BaseConstants.STORAGE_CONFIG.getString(BaseConstants.STORAGE_METHOD);
if (DbTypeEnum.MySQL.getType().equalsIgnoreCase(storageMethod)) {
return DbTypeEnum.MySQL.getType();
}
return DbTypeEnum.SQLite.getType();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy