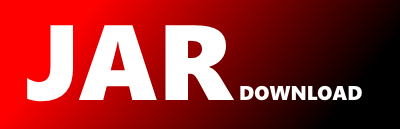
cn.handyplus.lib.util.LegacyUtil Maven / Gradle / Ivy
The newest version!
package cn.handyplus.lib.util;
import cn.handyplus.lib.constants.BaseConstants;
import cn.handyplus.lib.constants.VersionCheckEnum;
import org.bukkit.GameRule;
import org.bukkit.Particle;
import org.bukkit.World;
import org.bukkit.attribute.Attribute;
import org.bukkit.attribute.AttributeInstance;
import org.bukkit.enchantments.Enchantment;
import org.bukkit.entity.EntityType;
import org.bukkit.entity.Player;
import org.bukkit.potion.PotionEffectType;
import java.util.Arrays;
import java.util.List;
import java.util.Objects;
import java.util.stream.Collectors;
/**
* 兼容历史版本
*
* @author handy
* @since 3.7.9
*/
@SuppressWarnings("deprecation")
public class LegacyUtil {
private LegacyUtil() {
}
/**
* 设置最大血量
*
* @param player 玩家
* @param maxHealth 血量
* @since 3.7.9
*/
public static void setMaxHealth(Player player, double maxHealth) {
// 高版本处理
if (BaseConstants.VERSION_ID > VersionCheckEnum.V_1_8_8.getVersionId()) {
AttributeInstance attribute = player.getAttribute(Attribute.GENERIC_MAX_HEALTH);
if (attribute != null) {
attribute.setBaseValue(maxHealth);
return;
}
}
player.setMaxHealth(maxHealth);
}
/**
* 获取最大血量
*
* @param player 玩家
* @since 3.7.9
*/
public static double getMaxHealth(Player player) {
// 高版本处理
if (BaseConstants.VERSION_ID > VersionCheckEnum.V_1_8_8.getVersionId()) {
AttributeInstance attribute = player.getAttribute(Attribute.GENERIC_MAX_HEALTH);
if (attribute != null) {
return attribute.getValue();
}
}
return player.getMaxHealth();
}
/**
* 设置世界游戏规则
*
* @param world 世界
* @param ruleName 规则名称
* @param value 值
*/
@SuppressWarnings({"rawtypes", "unchecked"})
public static void setGameRuleValue(World world, String ruleName, Object value) {
if (value == null) {
return;
}
if (BaseConstants.VERSION_ID > VersionCheckEnum.V_1_12.getVersionId()) {
GameRule gameRule = GameRule.getByName(ruleName);
if (gameRule == null) {
MessageUtil.sendConsoleMessage("世界规则: " + ruleName + " 不存在.");
return;
}
if (value instanceof Boolean) {
world.setGameRule(gameRule, (Boolean) value);
return;
}
if (value instanceof Integer) {
world.setGameRule(gameRule, (Integer) value);
return;
}
}
world.setGameRuleValue(ruleName, String.valueOf(value));
}
/**
* 获取耐久附魔
*
* @return 耐久附魔
* @since 3.9.0
*/
public static Enchantment getDurability() {
if (BaseConstants.VERSION_ID > VersionCheckEnum.V_1_20_4.getVersionId()) {
return Enchantment.UNBREAKING;
}
return getEnchantmentByName("DURABILITY");
}
/**
* 获取力量附魔
*
* @return 力量附魔
* @since 3.9.9
*/
public static Enchantment getArrowDamage() {
if (BaseConstants.VERSION_ID > VersionCheckEnum.V_1_20_4.getVersionId()) {
return Enchantment.POWER;
}
return getEnchantmentByName("ARROW_DAMAGE");
}
/**
* 获取烟花粒子
*
* @return 烟花粒子
* @since 3.9.1
*/
public static Particle getFirework() {
if (BaseConstants.VERSION_ID > VersionCheckEnum.V_1_20_4.getVersionId()) {
return Particle.FIREWORK;
}
return Particle.valueOf("FIREWORKS_SPARK");
}
/**
* 获取滴水熔岩
*
* @return 滴水熔岩
* @since 3.9.1
*/
public static Particle getDripLava() {
if (BaseConstants.VERSION_ID > VersionCheckEnum.V_1_20_4.getVersionId()) {
return Particle.DRIPPING_LAVA;
}
return Particle.valueOf("DRIP_LAVA");
}
/**
* 获取红石
*
* @return 红石
* @since 3.9.9
*/
public static Particle getRedStone() {
if (BaseConstants.VERSION_ID > VersionCheckEnum.V_1_20_4.getVersionId()) {
return Particle.DUST;
}
return Particle.valueOf("REDSTONE");
}
/**
* 获取附魔
*
* @param name 名称
* @since 3.7.9
*/
public static Enchantment getEnchantmentByName(String name) {
return Enchantment.getByName(name);
}
/**
* 获取附魔名称
*
* @param enchantment 附魔
* @since 3.7.9
*/
public static String getName(Enchantment enchantment) {
return enchantment.getName();
}
/**
* 获取实体名称
*
* @param entityType 实体
* @since 3.7.9
*/
public static String getName(EntityType entityType) {
try {
// 高版本处理
if (BaseConstants.VERSION_ID > VersionCheckEnum.V_1_13_2.getVersionId()) {
return entityType.getKey().getKey();
}
return entityType.getName();
} catch (Exception exception) {
return entityType.getName();
}
}
/**
* 获取全部实体名称list
*
* @return 实体名称list
*/
public static List getEntityTypeList() {
// 高版本处理
if (BaseConstants.VERSION_ID > VersionCheckEnum.V_1_13_2.getVersionId()) {
return Arrays.stream(EntityType.values()).filter(e -> e != EntityType.UNKNOWN).map(e -> e.getKey().getKey()).collect(Collectors.toList());
}
return Arrays.stream(EntityType.values()).map(EntityType::getName).filter(Objects::nonNull).collect(Collectors.toList());
}
/**
* 获取全部附魔名称list
*
* @return 附魔名称list
*/
public static List getEnchantmentList() {
return Arrays.stream(Enchantment.values()).map(Enchantment::getName).collect(Collectors.toList());
}
/**
* 获取伤害增加药水
*
* @return PotionEffectType.INCREASE_DAMAGE
* @since 3.9.9
*/
public static PotionEffectType getIncreaseDamage() {
if (BaseConstants.VERSION_ID > VersionCheckEnum.V_1_20_4.getVersionId()) {
return PotionEffectType.getByName("strength");
}
return PotionEffectType.getByName("increase_damage");
}
/**
* 获取实体
*
* @param name 实体名称
* @return 实体
* @since 3.7.9
*/
public static EntityType getEntityType(String name) {
return EntityType.fromName(name);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy