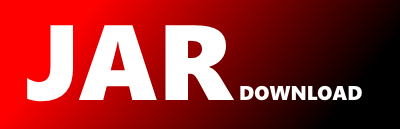
cn.handyplus.lib.util.RgbTextUtil Maven / Gradle / Ivy
package cn.handyplus.lib.util;
import cn.handyplus.lib.constants.BaseConstants;
import cn.handyplus.lib.constants.VersionCheckEnum;
import cn.handyplus.lib.core.StrUtil;
import net.md_5.bungee.api.chat.BaseComponent;
import net.md_5.bungee.api.chat.ClickEvent;
import net.md_5.bungee.api.chat.HoverEvent;
import net.md_5.bungee.api.chat.TextComponent;
import net.md_5.bungee.api.chat.hover.content.Text;
import java.util.Arrays;
import java.util.stream.Stream;
/**
* RGB处理
*
* @author handy
* @since 3.7.1
*/
public class RgbTextUtil {
private BaseComponent[] baseComponents;
private RgbTextUtil() {
}
/**
* 获取实例
*
* @return 实例
*/
public static RgbTextUtil getInstance() {
return new RgbTextUtil();
}
/**
* 初始化
*
* @param msg 消息内容
* @return this
*/
public RgbTextUtil init(String msg) {
return init(msg, true);
}
/**
* 初始化
*
* @param msg 消息内容
* @param isColor 是否加载颜色代码
* @return this
* @since 3.6.8
*/
public RgbTextUtil init(String msg, boolean isColor) {
this.baseComponents = TextComponent.fromLegacyText(isColor ? BaseUtil.replaceChatColor(msg) : msg);
return this;
}
/**
* 添加鼠标点击事件
*
* @param action 類型
* @param msg 消息
* @return this
* @since 3.0.9
*/
public RgbTextUtil addClick(ClickEvent.Action action, String msg) {
if (StrUtil.isEmpty(msg)) {
return this;
}
for (BaseComponent baseComponent : baseComponents) {
baseComponent.setClickEvent(new ClickEvent(action, msg));
}
return this;
}
/**
* 添加鼠标点击打开url
*
* @param url url
* @return this
*/
public RgbTextUtil addClickUrl(String url) {
return this.addClick(ClickEvent.Action.OPEN_URL, url);
}
/**
* 添加鼠标点击执行命令
*
* @param command 命令
* @return this
*/
public RgbTextUtil addClickCommand(String command) {
return this.addClick(ClickEvent.Action.RUN_COMMAND, command);
}
/**
* 添加鼠标点击 将给定的字符串插入到玩家的文本框中。
*
* @param suggestCommand 字符串
* @return this
* @since 3.0.9
*/
public RgbTextUtil addClickSuggestCommand(String suggestCommand) {
return this.addClick(ClickEvent.Action.SUGGEST_COMMAND, suggestCommand);
}
/**
* 添加文本内容复制到剪贴板 1.15+可用
*
* @param text 文字
* @return this
* @since 3.4.9
*/
public RgbTextUtil addClickCopyToClipboard(String text) {
if (BaseConstants.VERSION_ID < VersionCheckEnum.V_1_15.getVersionId()) {
return this;
}
return this.addClick(ClickEvent.Action.COPY_TO_CLIPBOARD, BaseUtil.replaceChatColor(text));
}
/**
* 添加鼠标移动上去显示效果 1.16+可用
*
* @param text 文字
* @return this
*/
public RgbTextUtil addHoverText(String text) {
if (BaseConstants.VERSION_ID < VersionCheckEnum.V_1_16.getVersionId()) {
return this;
}
if (StrUtil.isEmpty(text)) {
return this;
}
for (BaseComponent baseComponent : baseComponents) {
baseComponent.setHoverEvent(new HoverEvent(HoverEvent.Action.SHOW_TEXT, new Text(TextComponent.fromLegacyText(text))));
}
return this;
}
/**
* 添加组件
*
* @param extra 要附加的组件
* @return this
* @since 3.6.8
*/
public RgbTextUtil addExtra(BaseComponent[] extra) {
this.baseComponents = Stream.concat(Arrays.stream(this.baseComponents), Arrays.stream(extra)).toArray(BaseComponent[]::new);
return this;
}
/**
* 获取 TextComponent
*
* @return BaseComponent[]
*/
public BaseComponent[] build() {
return this.baseComponents;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy