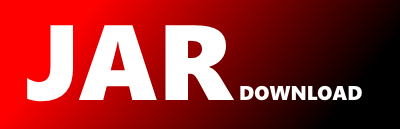
cn.herodotus.stirrup.logic.bpmn.mongodb.entity.ProcessSpecifics Maven / Gradle / Ivy
The newest version!
/*
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS HEADER.
*
* Copyright (c) 2020-2030 郑庚伟 ZHENGGENGWEI (码匠君), Licensed under the AGPL License
*
* This file is part of Herodotus Stirrup.
*
* Herodotus Stirrup is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published
* by the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* Herodotus Stirrup is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package cn.herodotus.stirrup.logic.bpmn.mongodb.entity;
import cn.herodotus.stirrup.data.crud.entity.AbstractMongoEntity;
import com.google.common.base.MoreObjects;
import io.swagger.v3.oas.annotations.media.Schema;
import jakarta.persistence.Id;
import org.springframework.data.mongodb.core.mapping.DBRef;
import org.springframework.data.mongodb.core.mapping.Document;
import java.util.List;
import java.util.Map;
/**
* Description: 流程详情数据
*
* @author : gengwei.zheng
* @date : 2023/2/24 18:05
*/
@Document(collection = "process-specifics")
public class ProcessSpecifics extends AbstractMongoEntity {
@Schema(name = "流程详情ID", title = "对应工作流引擎中的 BusinessKey")
@Id
private String id;
@Schema(name = "租户ID", title = "冗余字段方便其它代码使用")
private String tenantId;
@Schema(name = "流程定义ID", title = "冗余字段方便其它代码使用")
private String processDefinitionId;
@Schema(name = "流程定义KEY", title = "冗余字段方便其它代码使用")
private String processDefinitionKey;
@Schema(name = "当前任务ID", title = "用于获取当前任务对应的表单")
private String taskId;
@Schema(name = "当前节点ID", title = "当前任务节点对应的ID")
private String activityId;
@Schema(name = "当前节点名称", title = "当前任务节点对应的名称")
private String activityName;
@Schema(name = "是否是初次创建", title = "用于区分是为了生成BusinessKey的初次保存还是编辑状态")
private Boolean created = false;
@Schema(name = "表单输入数据")
private Map state;
@Schema(name = "审批意见", title = "对应该流程中的所有审批意见")
@DBRef
private List comments;
@Override
public String getId() {
return id;
}
@Override
public void setId(String id) {
this.id = id;
}
public String getTenantId() {
return tenantId;
}
public void setTenantId(String tenantId) {
this.tenantId = tenantId;
}
public String getProcessDefinitionId() {
return processDefinitionId;
}
public void setProcessDefinitionId(String processDefinitionId) {
this.processDefinitionId = processDefinitionId;
}
public String getProcessDefinitionKey() {
return processDefinitionKey;
}
public void setProcessDefinitionKey(String processDefinitionKey) {
this.processDefinitionKey = processDefinitionKey;
}
public String getTaskId() {
return taskId;
}
public void setTaskId(String taskId) {
this.taskId = taskId;
}
public String getActivityId() {
return activityId;
}
public void setActivityId(String activityId) {
this.activityId = activityId;
}
public String getActivityName() {
return activityName;
}
public void setActivityName(String activityName) {
this.activityName = activityName;
}
public Boolean getCreated() {
return created;
}
public void setCreated(Boolean created) {
this.created = created;
}
public Map getState() {
return state;
}
public void setState(Map state) {
this.state = state;
}
public List getComments() {
return comments;
}
public void setComments(List comments) {
this.comments = comments;
}
@Override
public String toString() {
return MoreObjects.toStringHelper(this)
.add("id", id)
.add("tenantId", tenantId)
.add("processDefinitionId", processDefinitionId)
.add("processDefinitionKey", processDefinitionKey)
.add("taskId", taskId)
.add("activityId", activityId)
.add("activityName", activityName)
.add("created", created)
.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy