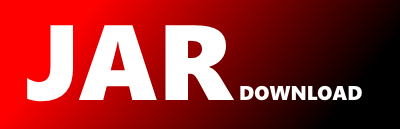
cn.hiboot.mcn.autoconfigure.web.exception.handler.DefaultExceptionHandler Maven / Gradle / Ivy
package cn.hiboot.mcn.autoconfigure.web.exception.handler;
import cn.hiboot.mcn.autoconfigure.config.ConfigProperties;
import cn.hiboot.mcn.autoconfigure.web.exception.ExceptionProperties;
import cn.hiboot.mcn.autoconfigure.web.exception.ExceptionResolver;
import cn.hiboot.mcn.autoconfigure.web.exception.GenericExceptionResolver;
import cn.hiboot.mcn.autoconfigure.web.exception.HttpStatusCodeResolver;
import cn.hiboot.mcn.core.exception.BaseException;
import cn.hiboot.mcn.core.exception.ErrorMsg;
import cn.hiboot.mcn.core.exception.ExceptionKeys;
import cn.hiboot.mcn.core.model.result.RestResp;
import cn.hiboot.mcn.core.util.McnUtils;
import cn.hiboot.mcn.core.util.SpringBeanUtils;
import com.fasterxml.jackson.databind.exc.InvalidFormatException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.aop.support.AopUtils;
import org.springframework.beans.factory.NoSuchBeanDefinitionException;
import org.springframework.beans.factory.ObjectProvider;
import org.springframework.beans.factory.config.BeanDefinition;
import org.springframework.beans.factory.support.DefaultListableBeanFactory;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.GenericApplicationContext;
import org.springframework.core.ResolvableType;
import org.springframework.http.converter.HttpMessageNotReadableException;
import org.springframework.util.ClassUtils;
import org.springframework.util.ConcurrentReferenceHashMap;
import org.springframework.validation.BindException;
import org.springframework.validation.BindingResult;
import org.springframework.validation.FieldError;
import org.springframework.web.method.annotation.MethodArgumentTypeMismatchException;
import org.springframework.web.multipart.MaxUploadSizeExceededException;
import org.springframework.web.server.ResponseStatusException;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.concurrent.ConcurrentHashMap;
import java.util.stream.Collectors;
/**
* DefaultExceptionHandler
*
* @author DingHao
* @since 2023/5/24 13:25
*/
public class DefaultExceptionHandler implements ExceptionHandler{
private final Logger log = LoggerFactory.getLogger(DefaultExceptionHandler.class);
/**
* 非BaseException的默认code码
*/
private static final int DEFAULT_ERROR_CODE = 999998;
private static final Map, List>> exceptionResolverCache = new ConcurrentHashMap<>();
private static final Map, ResolvableType> exceptionResolverTypeCache = new ConcurrentReferenceHashMap<>();
private final boolean validationExceptionPresent;
private final String basePackage;
private final boolean overrideHttpError;
private final ExceptionProperties properties;
private final String[] exceptionResolverNames;
private final ApplicationContext applicationContext;
private final ObjectProvider httpStatusCodeResolvers;
protected DefaultExceptionHandler(ExceptionProperties properties) {
this.properties = properties;
this.validationExceptionPresent = ClassUtils.isPresent("javax.validation.ValidationException", getClass().getClassLoader());
this.applicationContext = SpringBeanUtils.getApplicationContext();
this.httpStatusCodeResolvers = applicationContext.getBeanProvider(HttpStatusCodeResolver.class);
this.exceptionResolverNames = applicationContext.getBeanNamesForType(ExceptionResolver.class);
this.basePackage = applicationContext.getEnvironment().getProperty(ConfigProperties.APP_BASE_PACKAGE);
this.overrideHttpError = applicationContext.getEnvironment().getProperty("http.error.override",Boolean.class,true);
}
@Override
public ExceptionProperties config(){
return properties;
}
@Override
public RestResp handleException(Throwable exception) {
RestResp resp = null;
Class extends Throwable> exClass = exception.getClass();
List> exceptionResolvers = exceptionResolverCache.get(exClass);
if (exceptionResolvers == null) {
exceptionResolvers = Arrays.stream(exceptionResolverNames).map(s -> supportsExceptionType(s, exClass)).filter(Objects::nonNull).collect(Collectors.toList());
exceptionResolverCache.put(exClass,exceptionResolvers);
}
for (ExceptionResolver exceptionResolver : exceptionResolvers) {
resp = exceptionResolver.resolve(exception);
if (resp != null) {
break;
}
}
if(Objects.isNull(resp)){
RestResp
© 2015 - 2025 Weber Informatics LLC | Privacy Policy