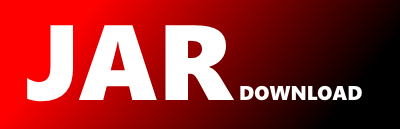
cn.hutool.core.map.multi.Table Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hutool-all Show documentation
Show all versions of hutool-all Show documentation
Hutool是一个小而全的Java工具类库,通过静态方法封装,降低相关API的学习成本,提高工作效率,使Java拥有函数式语言般的优雅,让Java语言也可以“甜甜的”。
package cn.hutool.core.map.multi;
import cn.hutool.core.collection.ListUtil;
import cn.hutool.core.lang.Opt;
import cn.hutool.core.lang.func.Consumer3;
import cn.hutool.core.map.MapUtil;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* 表格数据结构定义
* 此结构类似于Guava的Table接口,使用两个键映射到一个值,类似于表格结构。
*
* @param 行键类型
* @param 列键类型
* @param 值类型
* @since 5.7.23
*/
public interface Table extends Iterable> {
/**
* 是否包含指定行列的映射
* 行和列任意一个不存在都会返回{@code false},如果行和列都存在,值为{@code null},也会返回{@code true}
*
* @param rowKey 行键
* @param columnKey 列键
* @return 是否包含映射
*/
default boolean contains(R rowKey, C columnKey) {
return Opt.ofNullable(getRow(rowKey)).map((map) -> map.containsKey(columnKey))
.orElse(false);
}
//region Row
/**
* 行是否存在
*
* @param rowKey 行键
* @return 行是否存在
*/
default boolean containsRow(R rowKey) {
return Opt.ofNullable(rowMap()).map((map) -> map.containsKey(rowKey)).get();
}
/**
* 获取行
*
* @param rowKey 行键
* @return 行映射,返回的键为列键,值为表格的值
*/
default Map getRow(R rowKey) {
return Opt.ofNullable(rowMap()).map((map) -> map.get(rowKey)).get();
}
/**
* 返回所有行的key,行的key不可重复
*
* @return 行键
*/
default Set rowKeySet() {
return Opt.ofNullable(rowMap()).map(Map::keySet).get();
}
/**
* 返回行列对应的Map
*
* @return map,键为行键,值为列和值的对应map
*/
Map> rowMap();
//endregion
//region Column
/**
* 列是否存在
*
* @param columnKey 列键
* @return 列是否存在
*/
default boolean containsColumn(C columnKey) {
return Opt.ofNullable(columnMap()).map((map) -> map.containsKey(columnKey)).get();
}
/**
* 获取列
*
* @param columnKey 列键
* @return 列映射,返回的键为行键,值为表格的值
*/
default Map getColumn(C columnKey) {
return Opt.ofNullable(columnMap()).map((map) -> map.get(columnKey)).get();
}
/**
* 返回所有列的key,列的key不可重复
*
* @return 列set
*/
default Set columnKeySet() {
return Opt.ofNullable(columnMap()).map(Map::keySet).get();
}
/**
* 返回所有列的key,列的key如果实现Map是可重复key,则返回对应不去重的List。
*
* @return 列set
* @since 5.8.0
*/
default List columnKeys() {
final Map> columnMap = columnMap();
if(MapUtil.isEmpty(columnMap)){
return ListUtil.empty();
}
final List result = new ArrayList<>(columnMap.size());
for (Map.Entry> cMapEntry : columnMap.entrySet()) {
result.add(cMapEntry.getKey());
}
return result;
}
/**
* 返回列-行对应的map
*
* @return map,键为列键,值为行和值的对应map
*/
Map> columnMap();
//endregion
//region value
/**
* 指定值是否存在
*
* @param value 值
* @return 值
*/
default boolean containsValue(V value){
final Collection
© 2015 - 2024 Weber Informatics LLC | Privacy Policy